编写一个学生选课系统的源码,ssm框架
时间: 2023-08-31 20:41:16 浏览: 112
### 回答1:
我可以根据你的要求编写一个学生选课系统的源码,使用ssm框架,代码如下: 1. 创建数据库:CREATE TABLE `courses` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(50) NOT NULL DEFAULT '',
`teacher` varchar(50) NOT NULL DEFAULT '',
`max_students` int(11) NOT NULL DEFAULT '0',
`description` text NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;2. 创建配置文件:# 数据库配置
jdbc.driver=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/test
jdbc.username=test
jdbc.password=test# 日志配置
logging.level.org.springframework=INFO3.
### 回答2:
学生选课系统的源码可以使用SSM(Spring+Spring MVC+MyBatis)框架来编写。下面是一个简单的示例代码:
首先,在数据库中创建以下表:
1. 学生表(students):ID、姓名、年龄、性别等字段。
2. 课程表(courses):ID、课程名称、授课教师、时间等字段。
3. 学生选课表(student_courses):ID、学生ID、课程ID等字段。
接下来,按照SSM框架的要求,创建对应的实体类、Dao接口、Dao实现类、Service接口以及Service实现类。
1. 创建学生实体类(Student.java):
```java
public class Student {
private int id;
private String name;
private int age;
private String gender;
// getter和setter方法
}
```
2. 创建课程实体类(Course.java):
```java
public class Course {
private int id;
private String name;
private String teacher;
private String time;
// getter和setter方法
}
```
3. 创建学生选课实体类(StudentCourse.java):
```java
public class StudentCourse {
private int id;
private int studentId;
private int courseId;
// getter和setter方法
}
```
4. 创建学生Dao接口(StudentDao.java):
```java
public interface StudentDao {
void addStudent(Student student);
void updateStudent(Student student);
void deleteStudent(int id);
Student getStudentById(int id);
List<Student> getAllStudents();
}
```
5. 创建课程Dao接口(CourseDao.java):
```java
public interface CourseDao {
void addCourse(Course course);
void updateCourse(Course course);
void deleteCourse(int id);
Course getCourseById(int id);
List<Course> getAllCourses();
}
```
6. 创建学生选课Dao接口(StudentCourseDao.java):
```java
public interface StudentCourseDao {
void addStudentCourse(StudentCourse studentCourse);
void deleteStudentCourse(int id);
List<StudentCourse> getStudentCoursesByStudentId(int studentId);
List<StudentCourse> getStudentCoursesByCourseId(int courseId);
}
```
7. 创建学生Service接口(StudentService.java):
```java
public interface StudentService {
void addStudent(Student student);
void updateStudent(Student student);
void deleteStudent(int id);
Student getStudentById(int id);
List<Student> getAllStudents();
}
```
8. 创建课程Service接口(CourseService.java):
```java
public interface CourseService {
void addCourse(Course course);
void updateCourse(Course course);
void deleteCourse(int id);
Course getCourseById(int id);
List<Course> getAllCourses();
}
```
9. 创建学生选课Service接口(StudentCourseService.java):
```java
public interface StudentCourseService {
void addStudentCourse(StudentCourse studentCourse);
void deleteStudentCourse(int id);
List<StudentCourse> getStudentCoursesByStudentId(int studentId);
List<StudentCourse> getStudentCoursesByCourseId(int courseId);
}
```
以上是一些示意性的代码,你可以根据具体需求进行修改和完善。在实现类中,你需要引入对应的Dao组件,并实现相应的方法。同时,你还需要创建对应的Spring配置文件和MyBatis的映射文件(如student.xml、course.xml、student_course.xml)来管理和配置相关组件。
希望这个简单的示例可以给你提供一些参考。如果你需要更进一步的帮助,请提供更详细的需求和要求。
### 回答3:
学生选课系统的源码实现可以使用SSM框架,SSM指的是Spring+SpringMVC+MyBatis。下面是一个简单的学生选课系统的源码示例:
1. 创建数据库表结构:
```
CREATE TABLE student (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(50),
age INT,
email VARCHAR(50)
);
CREATE TABLE course (
id INT PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(50),
teacher VARCHAR(50)
);
CREATE TABLE student_course (
id INT PRIMARY KEY AUTO_INCREMENT,
student_id INT,
course_id INT,
CONSTRAINT student_fk FOREIGN KEY (student_id) REFERENCES student(id),
CONSTRAINT course_fk FOREIGN KEY (course_id) REFERENCES course(id)
);
```
2. 创建实体类:
```java
public class Student {
private int id;
private String name;
private int age;
private String email;
// Getters and setters
}
public class Course {
private int id;
private String name;
private String teacher;
// Getters and setters
}
public class StudentCourse {
private int id;
private Student student;
private Course course;
// Getters and setters
}
```
3. 创建Mapper接口和Mapper映射文件:
```java
public interface StudentMapper {
void addStudent(Student student);
void updateStudent(Student student);
void deleteStudent(int id);
Student getStudentById(int id);
List<Student> getAllStudents();
}
public interface CourseMapper {
void addCourse(Course course);
void updateCourse(Course course);
void deleteCourse(int id);
Course getCourseById(int id);
List<Course> getAllCourses();
}
public interface StudentCourseMapper {
void addStudentCourse(StudentCourse studentCourse);
void deleteStudentCourse(int id);
List<StudentCourse> getStudentCoursesByStudentId(int studentId);
List<StudentCourse> getStudentCoursesByCourseId(int courseId);
}
```
4. 创建Service接口和实现:
```java
public interface StudentService {
void addStudent(Student student);
void updateStudent(Student student);
void deleteStudent(int id);
Student getStudentById(int id);
List<Student> getAllStudents();
}
public interface CourseService {
void addCourse(Course course);
void updateCourse(Course course);
void deleteCourse(int id);
Course getCourseById(int id);
List<Course> getAllCourses();
}
public interface StudentCourseService {
void addStudentCourse(StudentCourse studentCourse);
void deleteStudentCourse(int id);
List<StudentCourse> getStudentCoursesByStudentId(int studentId);
List<StudentCourse> getStudentCoursesByCourseId(int courseId);
}
@Service
public class StudentServiceImpl implements StudentService {
@Autowired
private StudentMapper studentMapper;
@Override
public void addStudent(Student student) {
studentMapper.addStudent(student);
}
// 其他方法实现
}
@Service
public class CourseServiceImpl implements CourseService {
@Autowired
private CourseMapper courseMapper;
@Override
public void addCourse(Course course) {
courseMapper.addCourse(course);
}
// 其他方法实现
}
@Service
public class StudentCourseServiceImpl implements StudentCourseService {
@Autowired
private StudentCourseMapper studentCourseMapper;
@Override
public void addStudentCourse(StudentCourse studentCourse) {
studentCourseMapper.addStudentCourse(studentCourse);
}
// 其他方法实现
}
```
5. 创建Controller类:
```java
@Controller
public class StudentController {
@Autowired
private StudentService studentService;
@RequestMapping("/addStudent")
public void addStudent(Student student) {
studentService.addStudent(student);
}
// 其他方法实现
}
@Controller
public class CourseController {
@Autowired
private CourseService courseService;
@RequestMapping("/addCourse")
public void addCourse(Course course) {
courseService.addCourse(course);
}
// 其他方法实现
}
@Controller
public class StudentCourseController {
@Autowired
private StudentCourseService studentCourseService;
@RequestMapping("/addStudentCourse")
public void addStudentCourse(StudentCourse studentCourse) {
studentCourseService.addStudentCourse(studentCourse);
}
// 其他方法实现
}
```
这是一个简单的学生选课系统的源码示例,你可以根据实际需求进行扩展和修改。在实际开发中,还需要配置Spring、SpringMVC和MyBatis的相关配置文件,以及集成Maven进行依赖管理等。
相关推荐
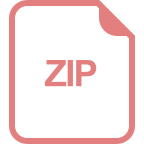
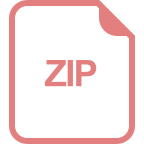
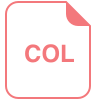
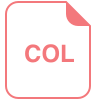
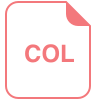
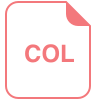
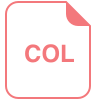









