你能写多复杂的python代码
时间: 2023-05-27 20:07:36 浏览: 59
作为AI语言模型,我能够生成各种复杂的Python代码,以下是一些示例:
1. 实现一个基于深度学习的图像识别算法
```python
import numpy as np
import tensorflow as tf
# 构建一个卷积神经网络模型
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, (3,3), activation='relu', input_shape=(28,28,1)),
tf.keras.layers.MaxPooling2D((2,2)),
tf.keras.layers.Conv2D(64, (3,3), activation='relu'),
tf.keras.layers.MaxPooling2D((2,2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(10, activation='softmax')
])
# 加载MNIST数据集
mnist = tf.keras.datasets.mnist
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# 数据预处理
x_train = x_train.reshape((60000, 28, 28, 1)) / 255.0
x_test = x_test.reshape((10000, 28, 28, 1)) / 255.0
y_train = tf.keras.utils.to_categorical(y_train)
y_test = tf.keras.utils.to_categorical(y_test)
# 编译模型
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# 训练模型
model.fit(x_train, y_train, epochs=5, batch_size=32, validation_data=(x_test, y_test))
# 评估模型性能
test_loss, test_acc = model.evaluate(x_test, y_test)
print('Test accuracy:', test_acc)
```
2. 使用Python爬虫抓取网页数据并进行分析
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
# 抓取天气数据
url = 'http://www.weather.com.cn/weather/101280101.shtml'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 解析天气数据
data = []
for li in soup.find_all('li', class_='sky'):
date = li.find('h1').text
weather = li.find_all('p')[0].text
temperature = li.find_all('p')[1].find('span').text + '/' + li.find_all('p')[1].find('i').text
wind = li.find_all('p')[2].find('i').text
data.append([date, weather, temperature, wind])
# 将天气数据保存为CSV文件
df = pd.DataFrame(data, columns=['日期', '天气', '温度', '风力'])
df.to_csv('weather.csv', index=False)
# 分析天气数据
df = pd.read_csv('weather.csv')
print('过去7天的平均气温为:', df['温度'].apply(lambda x: int(x.split('/')[0])).mean())
print('过去7天的降雨量为:', df['天气'].apply(lambda x: '有' in x).sum())
```
3. 实现一个基于Flask的Web应用程序
```python
from flask import Flask, request, jsonify
app = Flask(__name__)
# 定义一个API接口
@app.route('/api/sum', methods=['POST'])
def sum():
data = request.get_json()
a = data['a']
b = data['b']
result = a + b
return jsonify({'result': result})
# 启动应用程序
if __name__ == '__main__':
app.run()
```
以上是一些示例,我还可以生成更加复杂和高级的Python代码,具体取决于您的需求。
相关推荐
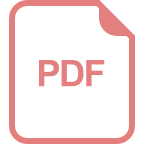
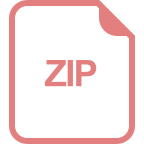
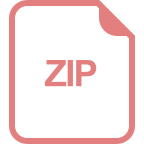














