用Python根据现有xml脚本和需求文件,生成新xml脚本,举例说明
时间: 2024-03-20 07:39:11 浏览: 132
好的,我可以给您举一个例子。
假设现有一个名为 `example.xml` 的 XML 脚本文件,其内容如下:
```xml
<?xml version="1.0"?>
<catalog>
<book id="001">
<author>John Doe</author>
<title>The Book of John</title>
<genre>Fiction</genre>
<price>20.00</price>
</book>
<book id="002">
<author>Jane Doe</author>
<title>The Book of Jane</title>
<genre>Non-Fiction</genre>
<price>15.00</price>
</book>
</catalog>
```
现在,我们需要根据需求文件生成一个新的 XML 脚本文件。需求文件的内容如下:
```text
- 添加一本名为 "The Book of Bob" 的书,作者为 "Bob Brown",类型为 "Fiction",价格为 25.00。
- 删除 id 为 "002" 的书。
```
我们可以使用 Python 中的 `xml.etree.ElementTree` 模块来解析现有的 XML 脚本文件,并根据需求文件生成新的 XML 脚本。下面是示例代码:
```python
import xml.etree.ElementTree as ET
# 解析现有的 XML 脚本文件
tree = ET.parse('example.xml')
root = tree.getroot()
# 根据需求文件添加新的书籍
new_book = ET.SubElement(root, 'book', {'id': '003'})
new_book_author = ET.SubElement(new_book, 'author')
new_book_author.text = 'Bob Brown'
new_book_title = ET.SubElement(new_book, 'title')
new_book_title.text = 'The Book of Bob'
new_book_genre = ET.SubElement(new_book, 'genre')
new_book_genre.text = 'Fiction'
new_book_price = ET.SubElement(new_book, 'price')
new_book_price.text = '25.00'
# 根据需求文件删除指定的书籍
for book in root.findall('book'):
if book.get('id') == '002':
root.remove(book)
# 生成新的 XML 脚本文件
tree.write('new_example.xml', encoding='utf-8', xml_declaration=True)
```
在上面的示例代码中,我们首先使用 `ET.parse()` 函数解析现有的 XML 脚本文件,然后使用 `ET.SubElement()` 函数根据需求文件添加新的书籍。接着,我们使用 `root.findall()` 函数查找要删除的书籍,并使用 `root.remove()` 函数删除它们。最后,我们使用 `tree.write()` 函数将生成的新的 XML 脚本文件写入到磁盘上。
阅读全文
相关推荐
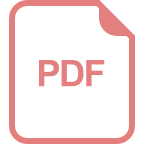

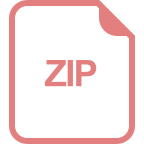
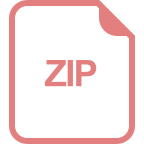
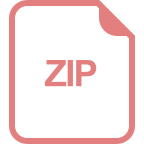
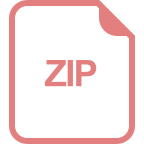
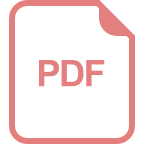
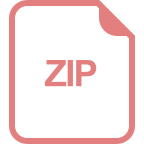
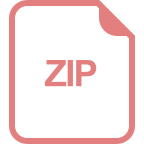
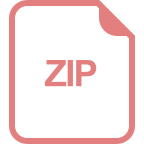
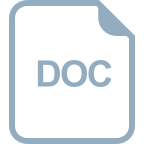
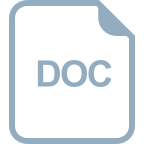
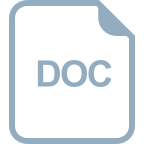


