java弹窗读取表格文件当中的书,并实现打印到控制台,不用数据库
时间: 2024-03-21 07:42:38 浏览: 14
好的,可以使用Java Swing中的JFileChooser类选择要读取的表格文件,再使用Apache POI库来读取表格文件中的内容,并将读取到的内容打印到控制台上。以下是示例代码:
```
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.WorkbookFactory;
public class ExcelFileChooserExample {
public static void main(String[] args) {
JFrame frame = new JFrame("表格文件选择器示例");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel();
JButton button = new JButton("选择表格文件");
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JFileChooser fileChooser = new JFileChooser();
int result = fileChooser.showOpenDialog(panel);
if (result == JFileChooser.APPROVE_OPTION) {
File selectedFile = fileChooser.getSelectedFile();
try {
Sheet sheet = WorkbookFactory.create(selectedFile).getSheetAt(0);
for (Row row : sheet) {
for (Cell cell : row) {
System.out.print(cell.toString() + "\t");
}
System.out.println();
}
JOptionPane.showMessageDialog(panel, "表格文件读取成功并已打印!");
} catch (Exception ex) {
ex.printStackTrace();
JOptionPane.showMessageDialog(panel, "表格文件读取失败:" + ex.getMessage());
}
}
}
});
panel.add(button);
frame.add(panel);
frame.pack();
frame.setVisible(true);
}
}
```
运行代码后,点击“选择表格文件”按钮,弹出文件选择器,选择要打印的表格文件,程序会自动读取表格文件中的内容,并将内容打印到控制台上,最后弹出提示框提示表格文件读取成功并已打印。需要注意的是,代码中使用的Apache POI库需要提前导入到项目中。
相关推荐
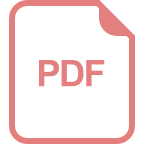
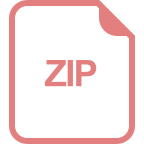














