java扫雷游戏并写每一步注释
时间: 2023-10-18 16:38:14 浏览: 92
很好,我可以帮你回答这个问题。以下是一个简单的Java扫雷游戏代码,并且我会在每一步都加上注释。请注意,这个代码仅仅是为了展示Java的基础语法,并不是一个完整的扫雷游戏。
```java
import java.util.Scanner; // 导入Scanner类
public class Minesweeper {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in); // 创建Scanner对象,用于读取用户输入
int rows, cols, bombs; // 定义行数、列数和地雷数量
System.out.println("Welcome to Minesweeper!"); // 输出欢迎信息
System.out.print("Enter the number of rows: "); // 提示用户输入行数
rows = scanner.nextInt(); // 读取用户输入的行数
System.out.print("Enter the number of columns: "); // 提示用户输入列数
cols = scanner.nextInt(); // 读取用户输入的列数
System.out.print("Enter the number of bombs: "); // 提示用户输入地雷数量
bombs = scanner.nextInt(); // 读取用户输入的地雷数量
char[][] board = new char[rows][cols]; // 创建二维字符数组,用于存储游戏面板
initializeBoard(board, rows, cols, bombs); // 调用initializeBoard方法,初始化游戏面板
printBoard(board, rows, cols); // 调用printBoard方法,输出游戏面板
}
// 初始化游戏面板
public static void initializeBoard(char[][] board, int rows, int cols, int bombs) {
// 将面板中所有方块都设置为'-',表示未被揭开
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
board[i][j] = '-';
}
}
// 随机放置地雷
int count = 0; // 记录放置的地雷数量
while (count < bombs) {
int row = (int) (Math.random() * rows);
int col = (int) (Math.random() * cols);
if (board[row][col] != 'B') { // 如果该位置没有地雷,则将其设置为地雷
board[row][col] = 'B';
count++; // 放置的地雷数量加1
}
}
}
// 输出游戏面板
public static void printBoard(char[][] board, int rows, int cols) {
// 输出列号
System.out.print(" ");
for (int j = 0; j < cols; j++) {
System.out.print(j);
}
System.out.println();
// 输出行号和每个方块的状态
for (int i = 0; i < rows; i++) {
System.out.print(i + " ");
for (int j = 0; j < cols; j++) {
System.out.print(board[i][j]);
}
System.out.println();
}
}
}
```
这个代码主要包括三个方法:
1. `initializeBoard`:用于初始化游戏面板,将面板中所有方块都设置为'-',并随机放置指定数量的地雷。
2. `printBoard`:用于输出游戏面板,包括行号、列号和每个方块的状态。
3. `main`:用于主程序,先提示用户输入行数、列数和地雷数量,然后调用`initializeBoard`方法初始化游戏面板,最后调用`printBoard`方法输出游戏面板。
希望这个简单的示例能够帮助你更好地理解Java的基础语法。
阅读全文
相关推荐

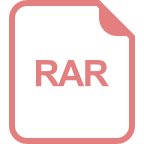
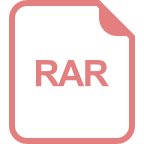

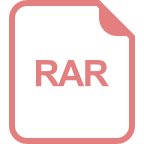

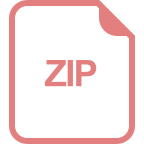

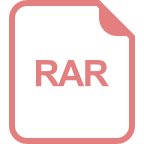