使用websocket 和apache ssd写一个从前端输入命令到远程服务器执行的Java程序
时间: 2024-03-12 21:46:23 浏览: 21
这里是一个基本的Java程序示例,可以使用WebSocket和Apache SSH库将从前端输入的命令发送到远程服务器并执行:
```java
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.TimeUnit;
import org.apache.sshd.client.SshClient;
import org.apache.sshd.client.channel.ChannelExec;
import org.apache.sshd.client.keyverifier.PromiscuousVerifier;
import org.apache.sshd.client.session.ClientSession;
import org.apache.sshd.common.keyprovider.FileKeyPairProvider;
import org.apache.sshd.common.util.io.NoCloseInputStream;
import org.glassfish.tyrus.client.ClientManager;
import org.glassfish.tyrus.client.ClientProperties;
import org.glassfish.tyrus.client.SslContextConfigurator;
import org.glassfish.tyrus.client.auth.AuthConfig;
import org.glassfish.tyrus.client.auth.Credentials;
import javax.net.ssl.SSLContext;
import javax.websocket.ClientEndpoint;
import javax.websocket.CloseReason;
import javax.websocket.ContainerProvider;
import javax.websocket.DeploymentException;
import javax.websocket.OnClose;
import javax.websocket.OnError;
import javax.websocket.OnMessage;
import javax.websocket.OnOpen;
import javax.websocket.Session;
import javax.websocket.WebSocketContainer;
@ClientEndpoint
public class RemoteShellClient {
private static final String REMOTE_HOST = "remote.hostname.com";
private static final int REMOTE_PORT = 22;
private static final String REMOTE_USERNAME = "remote_username";
private static final String REMOTE_PASSWORD = "remote_password";
private static final String REMOTE_PRIVATE_KEY_PATH = "/path/to/remote/private/key";
private static final String REMOTE_PUBLIC_KEY_PATH = "/path/to/remote/public/key";
private static final String WEBSOCKET_URL = "wss://websocket.server.com";
private Session websocketSession;
private ClientSession sshSession;
private ChannelExec sshChannel;
private CountDownLatch latch;
public RemoteShellClient() {
this.latch = new CountDownLatch(1);
}
@OnOpen
public void onWebSocketConnect(Session session) {
this.websocketSession = session;
}
@OnMessage
public void onWebSocketText(String command) {
try {
// Connect to remote host
SshClient client = SshClient.setUpDefaultClient();
client.setKeyPairProvider(new FileKeyPairProvider(new String[] { REMOTE_PRIVATE_KEY_PATH }));
client.setServerKeyVerifier(new PromiscuousVerifier());
client.start();
// Authenticate with remote host
AuthConfig authConfig = AuthConfig.builder()
.setCredentials(new Credentials() {
@Override
public boolean matches(String username, String password) {
return REMOTE_USERNAME.equals(username) && REMOTE_PASSWORD.equals(password);
}
})
.build();
this.sshSession = client.connect(REMOTE_HOST, REMOTE_PORT).auth(authConfig).getSession();
// Start shell channel
this.sshChannel = (ChannelExec) this.sshSession.createChannel("exec");
this.sshChannel.setCommand(command);
this.sshChannel.setIn(new NoCloseInputStream(System.in));
this.sshChannel.setOut(System.out);
this.sshChannel.setErr(System.err);
this.sshChannel.open();
// Wait for channel to close
this.latch.await();
// Disconnect from remote host
this.sshChannel.disconnect();
this.sshSession.close();
client.stop();
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
@OnClose
public void onWebSocketClose(CloseReason reason) {
this.latch.countDown();
}
@OnError
public void onWebSocketError(Throwable cause) {
cause.printStackTrace();
}
public void run() throws URISyntaxException, DeploymentException, IOException, InterruptedException {
// Configure SSL context
SSLContext sslContext = SSLContext.getDefault();
SslContextConfigurator sslContextConfigurator = new SslContextConfigurator();
sslContextConfigurator.setSslContext(sslContext);
// Configure WebSocket client
ClientManager client = ClientManager.createClient();
client.getProperties().put(ClientProperties.SSL_CONTEXT, sslContext);
client.getProperties().put(ClientProperties.AUTH_CONFIG, AuthConfig.Builder.create().build());
client.connectToServer(this, new URI(WEBSOCKET_URL));
// Wait for WebSocket session to close
this.latch.await(1, TimeUnit.DAYS);
}
public static void main(String[] args) {
try {
RemoteShellClient client = new RemoteShellClient();
client.run();
} catch (URISyntaxException | DeploymentException | IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
```
在这个示例中,我们首先配置了远程主机的连接信息和WebSocket服务器的URL。当从WebSocket接收到文本消息时,我们使用Apache SSH库连接到远程主机并启动一个shell通道来执行接收到的命令。然后等待shell通道关闭,并断开与远程主机的连接。
要运行此程序,您需要将WebSocket和Apache SSH库添加到类路径中,并将示例中的REMOTE_HOST、REMOTE_PORT、REMOTE_USERNAME、REMOTE_PASSWORD、REMOTE_PRIVATE_KEY_PATH、REMOTE_PUBLIC_KEY_PATH和WEBSOCKET_URL替换为实际值。
相关推荐
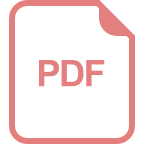
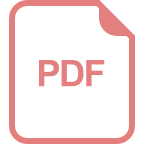
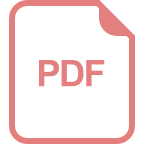
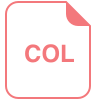
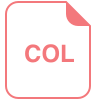
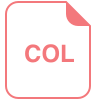
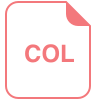
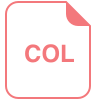









