STM32F103C8T6与128x64 OLED显示屏i2c
时间: 2024-04-25 07:05:07 浏览: 12
要使用STM32F103C8T6与128x64 OLED显示屏i2c进行通信,您需要在STM32F103C8T6上配置I2C总线,并将其连接到OLED显示屏。以下是一些步骤:
1. 在STM32F103C8T6上配置I2C总线。您需要配置I2C的GPIO引脚,并设置I2C的时钟频率和协议。
2. 连接I2C总线到OLED显示屏。您需要将I2C总线的SCL和SDA引脚连接到OLED显示屏的SCL和SDA引脚。
3. 编写代码以控制OLED显示屏。您需要使用I2C总线发送数据到OLED显示屏,以便控制其显示内容。
以下是一个简单的示例代码,用于通过I2C总线向OLED显示屏发送数据:
```c
#include "stm32f10x.h"
#include "oled.h"
#define OLED_I2C_ADDR 0x78
void oled_init(void)
{
// 初始化I2C总线
i2c_init();
// 初始化OLED显示屏
const uint8_t init_cmd[] = {
0xAE, // 关闭显示
0xD5, 0xF0, // 设置时钟分频因子,震荡频率
0xA8, 0x3F, // 设置驱动路数
0xD3, 0x00, // 设置显示偏移
0x40, // 设置起始行
0x8D, 0x14, // 启用内部电源,设置电源系数
0x20, 0x00, // 设置内存地址模式
0xA1, // 按列地址从左到右
0xC8, // 按行地址从上到下
0xDA, 0x12, // 设置COM硬件引脚配置
0x81, 0xCF, // 设置对比度
0xD9, 0xF1, // 设置预充电周期
0xDB, 0x40, // 设置VCOMH电压倍率
0xA4, // 全局显示开
0xA6, // 设置显示方式
0xAF // 开启显示
};
i2c_write(OLED_I2C_ADDR, init_cmd, sizeof(init_cmd));
}
void oled_clear(void)
{
const uint8_t clear_cmd[] = {
0x21, 0, 127, // 设置列地址范围
0x22, 0, 7 // 设置页地址范围
};
i2c_write(OLED_I2C_ADDR, clear_cmd, sizeof(clear_cmd));
uint8_t data[128 * 8] = {0};
i2c_write(OLED_I2C_ADDR, data, sizeof(data));
}
void oled_draw_pixel(uint8_t x, uint8_t y, uint8_t color)
{
const uint8_t set_col_cmd[] = {
0x21, x, x // 设置列地址范围
};
i2c_write(OLED_I2C_ADDR, set_col_cmd, sizeof(set_col_cmd));
const uint8_t set_page_cmd[] = {
0x22, y / 8, y / 8 // 设置页地址范围
};
i2c_write(OLED_I2C_ADDR, set_page_cmd, sizeof(set_page_cmd));
uint8_t data = 1 << (y % 8);
if (color == 0) {
data = ~data;
}
i2c_write(OLED_I2C_ADDR, &data, sizeof(data));
}
void oled_draw_rect(uint8_t x, uint8_t y, uint8_t w, uint8_t h, uint8_t color)
{
for (uint8_t i = 0; i < w; i++) {
for (uint8_t j = 0; j < h; j++) {
oled_draw_pixel(x + i, y + j, color);
}
}
}
void oled_draw_char(uint8_t x, uint8_t y, char ch, uint8_t color)
{
const uint8_t *font = font8x8_basic[ch];
for (uint8_t i = 0; i < 8; i++) {
for (uint8_t j = 0; j < 8; j++) {
if (font[i] & (1 << j)) {
oled_draw_pixel(x + j, y + i, color);
}
}
}
}
void oled_draw_string(uint8_t x, uint8_t y, const char *str, uint8_t color)
{
while (*str) {
oled_draw_char(x, y, *str++, color);
x += 8;
}
}
```
这个示例代码使用了一个名为`i2c_init()`的函数,这个函数用于初始化I2C总线。您需要根据您的具体硬件配置和I2C协议规范来实现这个函数。
在上面的代码中,`oled_init()`函数用于初始化OLED显示屏,`oled_clear()`函数用于清空OLED显示屏,`oled_draw_pixel()`函数用于绘制单个像素,`oled_draw_rect()`函数用于绘制矩形,`oled_draw_char()`函数用于绘制单个字符,`oled_draw_string()`函数用于绘制字符串。
这个示例代码中使用了一个名为`font8x8_basic[]`的数组,这个数组包含了8x8像素大小的基本ASCII字符的字形数据。您需要根据您的具体需求和字体库来修改这个数组。
希望这个示例代码能够帮助您开始使用STM32F103C8T6和128x64 OLED显示屏i2c进行通信。
相关推荐
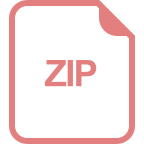
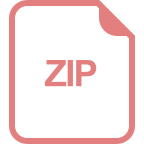














