用C++实现一个加密算法
时间: 2024-05-17 20:14:31 浏览: 165
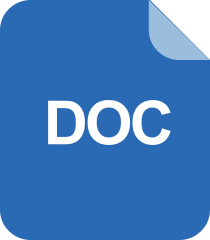
C++加密算法
好的,我可以给你一个简单的加密算法示例,使用C++实现。
这个算法叫做凯撒密码,也叫移位密码。它的原理是将明文中的每个字母按照一定的偏移量进行替换,从而得到密文。偏移量可以是任意数字,通常为一个固定的值。
下面是一个使用C++实现的凯撒密码加密算法:
```c++
#include <iostream>
#include <string>
using namespace std;
string encrypt(string plaintext, int shift) {
string ciphertext = "";
for (int i = 0; i < plaintext.length(); i++) {
char c = plaintext[i];
// 判断是否是字母
if (isalpha(c)) {
// 将字母向后移动 shift 个位置
c = toupper(c) + shift;
if (c > 'Z') {
c -= 26;
}
}
ciphertext += c;
}
return ciphertext;
}
int main() {
string plaintext = "hello world";
int shift = 3;
string ciphertext = encrypt(plaintext, shift);
cout << "明文: " << plaintext << endl;
cout << "密文: " << ciphertext << endl;
return 0;
}
```
这个程序实现了一个简单的凯撒密码加密算法。它将明文中的每个字母向后移动3个位置,并输出密文。输出结果如下:
```
明文: hello world
密文: KHOOR ZRUOG
```
需要注意的是,这个加密算法非常简单,容易被破解。在实际应用中应该使用更加安全的加密算法,比如AES、RSA等。
阅读全文
相关推荐
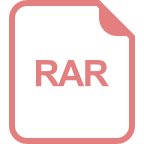
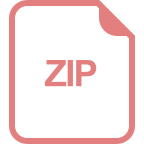
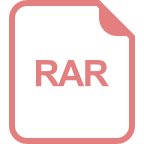
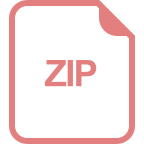
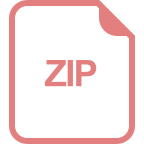
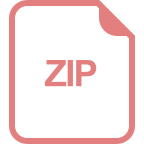
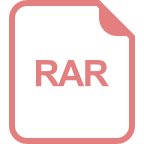
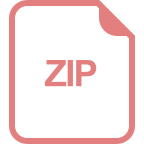
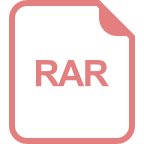
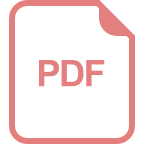
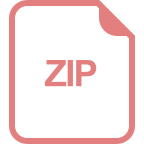
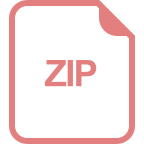
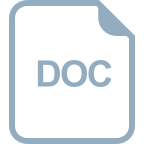