python request 大文件切片上传代码
时间: 2023-11-02 11:03:34 浏览: 55
下面是使用 Python requests 库实现大文件切片上传的示例代码:
```python
import os
import requests
# 定义上传文件的路径和名称
filepath = 'path/to/file'
filename = os.path.basename(filepath)
# 定义分片大小(500KB)
chunk_size = 500 * 1024
# 获取文件大小
file_size = os.path.getsize(filepath)
# 计算分片数量
chunk_num = file_size // chunk_size
if file_size % chunk_size != 0:
chunk_num += 1
# 上传文件
with open(filepath, 'rb') as f:
for i in range(chunk_num):
# 计算分片的起始位置和结束位置
start = i * chunk_size
if i == chunk_num - 1:
end = file_size
else:
end = start + chunk_size
# 读取分片数据
f.seek(start)
data = f.read(end - start)
# 构造请求头
headers = {
'Content-Type': 'application/octet-stream',
'Content-Disposition': 'attachment; filename="{}"'.format(filename),
'Content-Range': 'bytes {}-{}/{}'.format(start, end - 1, file_size),
}
# 发送分片数据
resp = requests.put(
'http://example.com/upload',
headers=headers,
data=data
)
# 打印响应结果
print(resp.status_code, resp.text)
```
以上代码实现了将一个大文件切片上传到远程服务器的功能。其中,我们通过 `os.path.getsize()` 获取文件大小,通过 `range()` 函数计算分片数量,然后循环读取每个分片并发送到远程服务器。在发送每个分片时,我们需要设置请求头中的 `Content-Type` 为 `application/octet-stream`,表示二进制数据流,同时设置 `Content-Disposition` 为 `attachment` 并指定文件名,以及设置 `Content-Range` 表示当前分片的范围。最后,我们通过 `requests.put()` 函数发送请求,并打印响应结果。
相关推荐
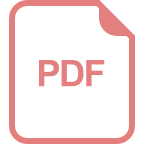
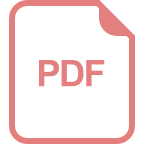














