给我一段在revit中自动铺设构件的代码
时间: 2023-09-06 09:03:59 浏览: 226
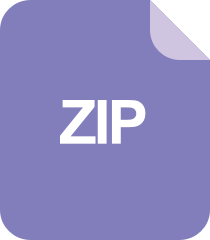
revitmep在电气设计中的应用共4页.pdf.zip
### 回答1:
要想自动铺设构件,你可以使用Revit API中的PlaceFamilyInstance()方法来实现。下面是一段示例代码:Document doc = this.ActiveUIDocument.Document; // 初始化文档
FamilySymbol fs = new FilteredElementCollector(doc) // 获取要安装的族类型
.OfClass(typeof(FamilySymbol))
.FirstElement();// 在此处添加你的铺设参数
XYZ point = new XYZ(1,1,1);
Level level = new FilteredElementCollector(doc)
.OfClass(typeof(Level))
.FirstElement() as Level;// 开始安装族
doc.Create.NewFamilyInstance(point, fs, level, Autodesk.Revit.DB.Structure.StructuralType.NonStructural);
### 回答2:
在Revit中,要实现自动铺设构件的代码需要使用Revit API。以下是一个简单的示例代码:
```python
import clr
clr.AddReference('RevitAPI')
import Autodesk
from Autodesk.Revit.DB import*
doc = __revit__.ActiveUIDocument.Document
uidoc = __revit__.ActiveUIDocument
def AutoPlaceComponents():
collector = FilteredElementCollector(doc).OfCategory(BuiltInCategory.OST_Furniture).OfClass(FamilyInstance)
for instance in collector:
# 获取构件类型
family_type = doc.GetElement(instance.GetTypeId())
if family_type.Category.Id == BuiltInCategory.OST_Furniture:
# 获取构件族的Symbol
family_symbol = family_type.Symbol
# 获取构件的定位点
location = instance.Location
if isinstance(location, LocationPoint):
point = location.Point
# 创建新的构件实例
new_instance = doc.Create.NewFamilyInstance(point, family_symbol, instance.Level, StructuralType.NonStructural)
# 将新构件放置在合适的位置
# 这里可以根据具体需求进行自定义
# 设定构件参数
# 这里可以根据具体需求进行自定义
# 将构件加入文档
doc.Regenerate()
uidoc.RefreshActiveView()
uidoc.Select(new_instance.Id)
uidoc.ShowElements(new_instance.Id)
AutoPlaceComponents()
```
此代码可以在Revit中自动铺设家具构件。具体步骤包括:获取家具构件实例、获取家具族的符号、获取构件的定位点、创建新的构件实例、放置构件在适当位置、设定构件参数以及将构件加入文档中等。请注意,这只是一个简单示例,具体代码需要根据实际需求进行修改和完善。
### 回答3:
在Revit中,自动铺设构件的代码可以通过使用Revit API来实现。以下是一个示例代码,用于在Revit中自动生成一系列墙体构件的铺设:
```
using Autodesk.Revit.ApplicationServices;
using Autodesk.Revit.Attributes;
using Autodesk.Revit.DB;
using Autodesk.Revit.UI;
[Transaction(TransactionMode.Manual)]
public class AutoLayoutWalls : IExternalCommand
{
public Result Execute(ExternalCommandData commandData, ref string message, ElementSet elements)
{
UIApplication uiApp = commandData.Application;
UIDocument uiDoc = uiApp.ActiveUIDocument;
Document doc = uiDoc.Document;
using (Transaction tx = new Transaction(doc))
{
tx.Start("Auto Layout Walls");
// 构件类型过滤器
ElementClassFilter wallFilter = new ElementClassFilter(typeof(Wall));
// 构件Collector
FilteredElementCollector collector = new FilteredElementCollector(doc);
collector.WherePasses(wallFilter);
// 获取所有墙体类型
IList<Element> walls = collector.ToElements();
// 铺设起始点及间距
XYZ startPoint = new XYZ(0, 0, 0);
double spacing = 5000; // 5000毫米
foreach (Element wall in walls)
{
WallType wallType = wall.WallType as WallType;
// 创建新的墙体实例
Wall newWall = Wall.Create(doc, wall.Location.Curve, wall.LevelId, wallType.Id, startPoint, false);
// 设置墙体其他属性,例如高度、材质等
startPoint += new XYZ(spacing, 0, 0); // 更新起始点
// 将新墙体添加到文档中
doc.Create.NewFamilyInstance(newWall.Location.Point, wallType, wall.Level, StructuralType.NonStructural);
// 提交事务
tx.Commit();
}
}
return Result.Succeeded;
}
}
```
这段代码首先使用Revit API中的相关类和方法实现了外部命令,然后通过API中的过滤器和Collector获取所有墙体构件的实例。接着通过遍历墙体实例,依次创建新的墙体构件,并设置墙体的其他属性。最后将新的墙体构件添加到文档中,并提交事务,完成墙体的自动铺设。请注意,代码中某些部分可能需要根据具体需求进行调整。
阅读全文
相关推荐
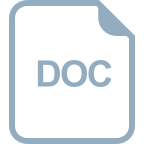
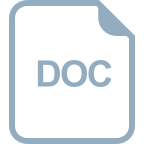


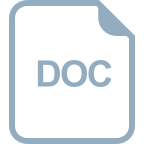
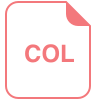











