python编写彩色蟒蛇
时间: 2024-10-10 17:03:40 浏览: 28
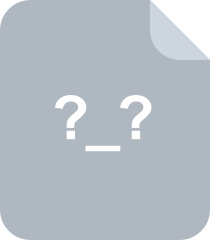
python标志七彩蟒蛇.py
Python中可以使用pygame库来创建交互式的彩色蟒蛇游戏。以下是一个简单的示例代码,演示如何创建基本的游戏框架:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置窗口大小
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("彩色蟒蛇")
# 定义颜色
colors = [(255, 0, 0), (0, 255, 0), (0, 0, 255)] # 红、绿、蓝
snake_color = colors[random.randint(0, len(colors) - 1)]
# 蛇的相关设置
snake_speed = 15
snake_pos = [50, 50]
snake_size = 10
snake_direction = "right"
# 主游戏循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT and snake_direction != "right":
snake_direction = "left"
elif event.key == pygame.K_RIGHT and snake_direction != "left":
snake_direction = "right"
elif event.key == pygame.K_UP and snake_direction != "down":
snake_direction = "up"
elif event.key == pygame.K_DOWN and snake_direction != "up":
snake_direction = "down"
# 移动蛇并处理边界
new_head = [snake_pos[0] + snake_direction_offset[0], snake_pos[1] + snake_direction_offset[1]]
if new_head[0] > screen_width or new_head[0] < 0 or new_head[1] > screen_height or new_head[1] < 0:
print("Game Over!")
running = False
else:
snake_pos.insert(0, new_head)
if snake_pos[-1] == snake_pos[0]:
print("You hit the wall!")
running = False
# 绘制蛇和食物
screen.fill((0, 0, 0)) # 清屏黑色背景
pygame.draw.rect(screen, snake_color, (snake_pos[0], snake_pos[1], snake_size, snake_size))
food_x, food_y = random.randint(0, screen_width // snake_size) * snake_size, random.randint(0, screen_height // snake_size) * snake_size
pygame.draw.rect(screen, (255, 255, 255), (food_x, food_y, snake_size, snake_size))
# 更新屏幕
pygame.display.update()
pygame.time.delay(snake_speed)
# 游戏结束,关闭窗口
pygame.quit()
```
这个例子只是一个基础版本,实际游戏中还需要增加蛇吃食物的增长、碰撞检测等功能,并可能需要更复杂的逻辑来控制游戏流程。
阅读全文
相关推荐
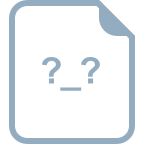
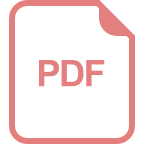
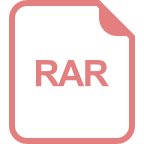
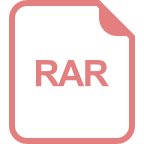
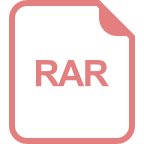
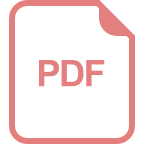
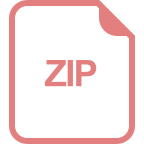
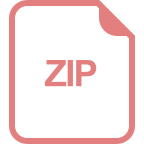
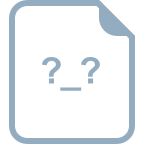
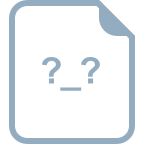
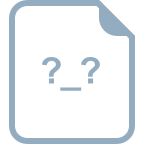
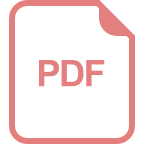