合宙air724ug与stm32连接爬取天气预报的代码
时间: 2023-08-12 17:06:30 浏览: 78
以下是合宙air724ug与stm32连接并爬取天气预报的代码示例:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include "stm32f10x.h"
#include "usart.h"
#define AT_CMD_BUF_LEN 128
#define RECV_BUF_LEN 512
#define TIMEOUT 3000
static char at_cmd_buf[AT_CMD_BUF_LEN];
static char recv_buf[RECV_BUF_LEN];
void delay(uint32_t nCount);
void send_at_cmd(char *cmd, uint32_t timeout)
{
USART_SendString(USART1, cmd);
delay(timeout);
}
int recv_at_resp(char *resp, uint32_t timeout)
{
int len = 0;
int i = 0;
while (timeout--) {
len = USART_GetString(USART1, recv_buf, RECV_BUF_LEN);
if (len > 0) {
if (strstr(recv_buf, resp) != NULL) {
return 1;
}
else {
i++;
if (i > 10) {
return 0;
}
}
}
delay(1);
}
return 0;
}
int main(void)
{
USART1_Init();
// 等待串口初始化完成
delay(1000);
// 设置AT指令回显关闭
send_at_cmd("ATE0\r\n", TIMEOUT);
if (!recv_at_resp("OK", TIMEOUT)) {
printf("ATE0 failed!\r\n");
return -1;
}
// 连接到WiFi
sprintf(at_cmd_buf, "AT+CWJAP=\"ssid\",\"password\"\r\n");
send_at_cmd(at_cmd_buf, TIMEOUT);
if (!recv_at_resp("OK", TIMEOUT)) {
printf("AT+CWJAP failed!\r\n");
return -1;
}
// 获取IP地址
send_at_cmd("AT+CIFSR\r\n", TIMEOUT);
if (!recv_at_resp("OK", TIMEOUT)) {
printf("AT+CIFSR failed!\r\n");
return -1;
}
// 打开TCP连接
send_at_cmd("AT+CIPSTART=\"TCP\",\"api.openweathermap.org\",80\r\n", TIMEOUT);
if (!recv_at_resp("CONNECT", TIMEOUT)) {
printf("AT+CIPSTART failed!\r\n");
return -1;
}
// 发送HTTP请求
sprintf(at_cmd_buf, "GET /data/2.5/weather?q=Shenzhen&appid=your_appid HTTP/1.1\r\nHost: api.openweathermap.org\r\nConnection: close\r\n\r\n");
sprintf(recv_buf, "HTTP/1.1");
send_at_cmd("AT+CIPSEND\r\n", TIMEOUT);
if (!recv_at_resp(">", TIMEOUT)) {
printf("AT+CIPSEND failed!\r\n");
return -1;
}
send_at_cmd(at_cmd_buf, TIMEOUT);
if (!recv_at_resp(recv_buf, TIMEOUT)) {
printf("HTTP request failed!\r\n");
return -1;
}
// 接收HTTP响应
while (1) {
int len = USART_GetString(USART1, recv_buf, RECV_BUF_LEN);
if (len > 0) {
printf("%s", recv_buf);
if (strstr(recv_buf, "}") != NULL) {
break;
}
}
delay(1);
}
// 关闭TCP连接
send_at_cmd("AT+CIPCLOSE\r\n", TIMEOUT);
while (1) {
;
}
return 0;
}
void delay(uint32_t nCount)
{
for(; nCount != 0; nCount--);
}
```
这段代码中,我们通过串口与合宙air724ug模组进行通信,使用AT指令连接到WiFi并打开TCP连接,然后发送HTTP请求获取天气预报信息,最后关闭TCP连接。在实际使用中,需要将代码中的ssid、password和your_appid替换为实际的WiFi名称、密码和OpenWeatherMap的API Key。
相关推荐
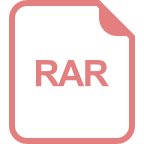














