请帮我用c语言写一个linux下spi通信的样例
时间: 2024-03-16 07:45:01 浏览: 117
好的,下面是一个简单的 Linux 下 SPI 通信的样例(假设使用的是 /dev/spidev0.0 设备):
```c
#include <stdio.h>
#include <stdint.h>
#include <string.h>
#include <fcntl.h>
#include <unistd.h>
#include <linux/spi/spidev.h>
int main(int argc, char *argv[]) {
int fd;
uint8_t tx_buf[2], rx_buf[2];
struct spi_ioc_transfer xfer;
// 打开 SPI 设备
fd = open("/dev/spidev0.0", O_RDWR);
if (fd < 0) {
perror("can't open device");
return -1;
}
// 配置 SPI 设备
uint32_t speed_hz = 1000000; // 1MHz
uint8_t bits_per_word = 8;
uint8_t mode = SPI_MODE_0;
if (ioctl(fd, SPI_IOC_WR_MODE, &mode) < 0) {
perror("can't set SPI mode");
return -1;
}
if (ioctl(fd, SPI_IOC_WR_BITS_PER_WORD, &bits_per_word) < 0) {
perror("can't set bits per word");
return -1;
}
if (ioctl(fd, SPI_IOC_WR_MAX_SPEED_HZ, &speed_hz) < 0) {
perror("can't set max speed hz");
return -1;
}
// 发送和接收数据
tx_buf[0] = 0x12; // 发送的数据
xfer.tx_buf = (unsigned long)tx_buf;
xfer.rx_buf = (unsigned long)rx_buf;
xfer.len = sizeof(tx_buf);
xfer.speed_hz = speed_hz;
xfer.bits_per_word = bits_per_word;
xfer.delay_usecs = 0;
xfer.cs_change = 0;
if (ioctl(fd, SPI_IOC_MESSAGE(1), &xfer) < 0) {
perror("can't transfer SPI message");
return -1;
}
// 输出接收到的数据
printf("Received: 0x%02x 0x%02x\n", rx_buf[0], rx_buf[1]);
// 关闭 SPI 设备
close(fd);
return 0;
}
```
这个样例中,我们使用了 `spi_ioc_transfer` 结构体,它用于描述 SPI 传输参数,包括发送和接收缓冲区、传输长度、传输速度、传输模式等等。我们通过 `ioctl` 函数设置 SPI 设备的参数,然后使用 `SPI_IOC_MESSAGE` 宏发送并接收数据。注意,这个样例只是一个简单的示例,实际使用时还需要根据具体设备的要求进行调整。
阅读全文
相关推荐








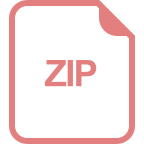



