o 编写一个C语言程序,使用标准库函数open(), write(), read(), close()等来打开和操作虚拟串口,实现向其中一个虚拟串口发送数据,并从另一个虚拟串口接收数据。
时间: 2024-04-30 16:18:33 浏览: 161
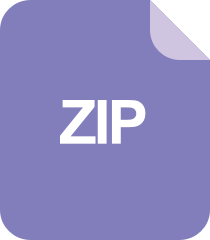
一个c语言编写的串口编程

这里提供一个简单的示例程序,可以参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <unistd.h>
#include <termios.h>
#define SERIAL_PORT_1 "/dev/pts/1"
#define SERIAL_PORT_2 "/dev/pts/2"
int main()
{
int fd1, fd2;
struct termios tio1, tio2;
char buf1[256], buf2[256];
int n1, n2;
// 打开两个虚拟串口
fd1 = open(SERIAL_PORT_1, O_RDWR | O_NOCTTY);
if (fd1 < 0) {
perror("open");
exit(1);
}
fd2 = open(SERIAL_PORT_2, O_RDWR | O_NOCTTY);
if (fd2 < 0) {
perror("open");
exit(1);
}
// 设置串口参数
memset(&tio1, 0, sizeof(tio1));
tio1.c_cflag = B9600 | CS8 | CLOCAL | CREAD;
tio1.c_iflag = IGNPAR;
tcsetattr(fd1, TCSANOW, &tio1);
memset(&tio2, 0, sizeof(tio2));
tio2.c_cflag = B9600 | CS8 | CLOCAL | CREAD;
tio2.c_iflag = IGNPAR;
tcsetattr(fd2, TCSANOW, &tio2);
// 向串口1发送数据
strcpy(buf1, "Hello, serial port!");
write(fd1, buf1, strlen(buf1));
// 从串口2接收数据
n2 = read(fd2, buf2, sizeof(buf2));
buf2[n2] = '\0';
printf("Received: %s\n", buf2);
// 关闭串口
close(fd1);
close(fd2);
return 0;
}
```
需要注意的是,这里使用的是 Linux 系统下的虚拟串口 `/dev/pts/1` 和 `/dev/pts/2`,在其他系统上可能会有所不同。另外,设置串口参数的部分可能需要根据实际情况进行调整。
阅读全文
相关推荐

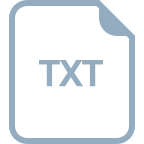




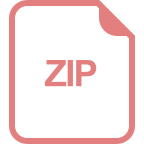
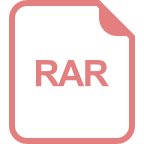
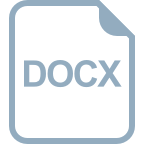
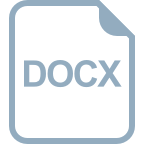