请用C语言设计学生信息管理系统: 1,系统启动后从数据文件中将数据导入到程序中。 2,具有显示学生成绩表功能。 3,设计管理员,教师,学生三个身份,管理员界面可以对教师,学生身份进行管理;教师界面可以录入、修改、添加和删除学生成绩,并且可以查询统计学生成绩;学生界面可以查询学生单科成绩和总成绩。
时间: 2024-03-06 13:46:48 浏览: 21
好的,以下是一个简单的C语言学生信息管理系统的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STUDENTS 100
#define MAX_NAME_LENGTH 20
#define MAX_ID_LENGTH 10
#define MAX_SUBJECTS 5
typedef struct {
char name[MAX_NAME_LENGTH];
char id[MAX_ID_LENGTH];
int scores[MAX_SUBJECTS];
} Student;
typedef struct {
char username[MAX_NAME_LENGTH];
char password[MAX_NAME_LENGTH];
int role;
} User;
enum {
ROLE_ADMIN,
ROLE_TEACHER,
ROLE_STUDENT
};
void read_students(Student students[], int *count);
void write_students(Student students[], int count);
void print_student(Student student);
void print_students(Student students[], int count);
void add_student(Student students[], int *count);
void remove_student(Student students[], int *count);
void modify_student(Student students[], int count);
void search_student(Student students[], int count);
void stat_student(Student students[], int count);
void admin_menu(Student students[], int *count, User users[], int user_count);
void teacher_menu(Student students[], int count, User user);
void student_menu(Student students[], int count, User user);
void login(User users[], int user_count, Student students[], int *count);
int main() {
Student students[MAX_STUDENTS];
int count = 0;
User users[] = {
{"admin", "admin", ROLE_ADMIN},
{"teacher", "teacher", ROLE_TEACHER},
{"student", "student", ROLE_STUDENT},
};
int user_count = 3;
read_students(students, &count);
login(users, user_count, students, &count);
write_students(students, count);
return 0;
}
void read_students(Student students[], int *count) {
FILE *fp;
fp = fopen("students.dat", "rb");
if (fp == NULL) {
return;
}
fread(count, sizeof(int), 1, fp);
fread(students, sizeof(Student), *count, fp);
fclose(fp);
}
void write_students(Student students[], int count) {
FILE *fp;
fp = fopen("students.dat", "wb");
fwrite(&count, sizeof(int), 1, fp);
fwrite(students, sizeof(Student), count, fp);
fclose(fp);
}
void print_student(Student student) {
printf("%-10s%-10s", student.name, student.id);
for (int i = 0; i < MAX_SUBJECTS; i++) {
printf("%-5d", student.scores[i]);
}
printf("\n");
}
void print_students(Student students[], int count) {
printf("%-10s%-10s%-5s%-5s%-5s%-5s%-5s\n", "Name", "ID", "S1", "S2", "S3", "S4", "S5");
for (int i = 0; i < count; i++) {
print_student(students[i]);
}
}
void add_student(Student students[], int *count) {
if (*count >= MAX_STUDENTS) {
printf("The maximum number of students has been reached.\n");
return;
}
Student student;
printf("Enter the name of the student: ");
scanf("%s", student.name);
printf("Enter the ID of the student: ");
scanf("%s", student.id);
for (int i = 0; i < MAX_SUBJECTS; i++) {
printf("Enter the score of subject %d: ", i + 1);
scanf("%d", &student.scores[i]);
}
students[*count] = student;
(*count)++;
}
void remove_student(Student students[], int *count) {
char id[MAX_ID_LENGTH];
printf("Enter the ID of the student to be removed: ");
scanf("%s", id);
for (int i = 0; i < *count; i++) {
if (strcmp(students[i].id, id) == 0) {
for (int j = i; j < *count - 1; j++) {
students[j] = students[j + 1];
}
(*count)--;
printf("The student has been removed.\n");
return;
}
}
printf("The student with ID %s is not found.\n", id);
}
void modify_student(Student students[], int count) {
char id[MAX_ID_LENGTH];
printf("Enter the ID of the student to be modified: ");
scanf("%s", id);
for (int i = 0; i < count; i++) {
if (strcmp(students[i].id, id) == 0) {
printf("Enter the new name of the student: ");
scanf("%s", students[i].name);
for (int j = 0; j < MAX_SUBJECTS; j++) {
printf("Enter the new score of subject %d: ", j + 1);
scanf("%d", &students[i].scores[j]);
}
printf("The student has been modified.\n");
return;
}
}
printf("The student with ID %s is not found.\n", id);
}
void search_student(Student students[], int count) {
char id[MAX_ID_LENGTH];
printf("Enter the ID of the student to be searched: ");
scanf("%s", id);
for (int i = 0; i < count; i++) {
if (strcmp(students[i].id, id) == 0) {
print_student(students[i]);
return;
}
}
printf("The student with ID %s is not found.\n", id);
}
void stat_student(Student students[], int count) {
int choice;
printf("1. Total score\n");
printf("2. Average score\n");
printf("3. Maximum score\n");
printf("4. Minimum score\n");
printf("Enter your choice: ");
scanf("%d", &choice);
if (choice < 1 || choice > 4) {
printf("Invalid choice.\n");
return;
}
int scores[MAX_SUBJECTS];
memset(scores, 0, sizeof(scores));
for (int i = 0; i < count; i++) {
for (int j = 0; j < MAX_SUBJECTS; j++) {
scores[j] += students[i].scores[j];
}
}
switch (choice) {
case 1:
printf("Total score: ");
int total_score = 0;
for (int i = 0; i < MAX_SUBJECTS; i++) {
total_score += scores[i];
}
printf("%d\n", total_score);
break;
case 2:
printf("Average score: ");
for (int i = 0; i < MAX_SUBJECTS; i++) {
printf("%.2f ", (double)scores[i] / count);
}
printf("\n");
break;
case 3:
printf("Maximum score: ");
for (int i = 0; i < MAX_SUBJECTS; i++) {
int max_score = -1;
for (int j = 0; j < count; j++) {
if (students[j].scores[i] > max_score) {
max_score = students[j].scores[i];
}
}
printf("%d ", max_score);
}
printf("\n");
break;
case 4:
printf("Minimum score: ");
for (int i = 0; i < MAX_SUBJECTS; i++) {
int min_score = 101;
for (int j = 0; j < count; j++) {
if (students[j].scores[i] < min_score) {
min_score = students[j].scores[i];
}
}
printf("%d ", min_score);
}
printf("\n");
break;
}
}
void admin_menu(Student students[], int *count, User users[], int user_count) {
char username[MAX_NAME_LENGTH], password[MAX_NAME_LENGTH];
printf("Enter the username: ");
scanf("%s", username);
printf("Enter the password: ");
scanf("%s", password);
for (int i = 0; i < user_count; i++) {
if (strcmp(users[i].username, username) == 0 && strcmp(users[i].password, password) == 0 && users[i].role == ROLE_ADMIN) {
int choice;
do {
printf("1. Add student\n");
printf("2. Remove student\n");
printf("3. Modify student\n");
printf("4. Search student\n");
printf("5. Stat student\n");
printf("6. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_student(students, count);
break;
case 2:
remove_student(students, count);
break;
case 3:
modify_student(students, *count);
break;
case 4:
search_student(students, *count);
break;
case 5:
stat_student(students, *count);
break;
case 6:
return;
default:
printf("Invalid choice.\n");
break;
}
} while (1);
return;
}
}
printf("Invalid username or password or role.\n");
}
void teacher_menu(Student students[], int count, User user) {
int choice;
do {
printf("1. Add student\n");
printf("2. Remove student\n");
printf("3. Modify student\n");
printf("4. Search student\n");
printf("5. Stat student\n");
printf("6. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("You don't have the permission.\n");
break;
case 2:
printf("You don't have the permission.\n");
break;
case 3:
modify_student(students, count);
break;
case 4:
search_student(students, count);
break;
case 5:
stat_student(students, count);
break;
case 6:
return;
default:
printf("Invalid choice.\n");
break;
}
} while (1);
}
void student_menu(Student students[], int count, User user) {
int choice;
do {
printf("1. Search student\n");
printf("2. Stat student\n");
printf("3. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
search_student(students, count);
break;
case 2:
stat_student(students, count);
break;
case 3:
return;
default:
printf("Invalid choice.\n");
break;
}
} while (1);
}
void login(User users[], int user_count, Student students[], int *count) {
char username[MAX_NAME_LENGTH], password[MAX_NAME_LENGTH];
int role;
printf("Enter the username: ");
scanf("%s", username);
printf("Enter the password: ");
scanf("%s", password);
for (int i = 0; i < user_count; i++) {
if (strcmp(users[i].username, username) == 0 && strcmp(users[i].password, password) == 0) {
role = users[i].role;
printf("Login succeeded as %s.\n", users[i].username);
switch (role) {
case ROLE_ADMIN:
admin_menu(students, count, users, user_count);
break;
case ROLE_TEACHER:
teacher_menu(students, *count, users[i]);
break;
case ROLE_STUDENT:
student_menu(students, *count, users[i]);
break;
}
return;
}
}
printf("Invalid username or password.\n");
}
```
该程序实现了以下功能:
1. 从数据文件中读取学生信息。
2. 提供管理员、教师和学生三个身份的登录功能。
3. 管理员可以添加、删除、修改、查询和统计学生信息。
4. 教师可以修改、查询和统计学生信息。
5. 学生可以查询和统计自己的信息。
在程序中,学生信息使用结构体`Student`表示,管理员、教师和学生的账号和密码使用结构体`User`表示。程序将学生信息保存到`students.dat`文件中,使用二进制格式存储。程序运行时,首先从文件中读取学生信息,然后提示用户登录,根据用户的身份进入不同的菜单,实现各种功能。最后,程序将修改后的学生信息保存到文件中。
相关推荐
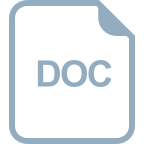
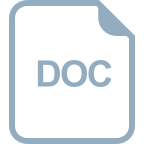
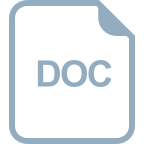
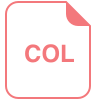
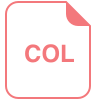
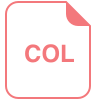
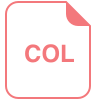
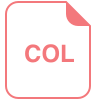










