在idle里运用lucene的代码
时间: 2024-09-27 14:06:35 浏览: 35
在IDLE(Python自带的集成开发环境)中使用Apache Lucene需要几个步骤,因为IDLE本身并不支持直接运行Java应用。以下是使用PyLucene的基本步骤,假设你已经安装了Jython和PyLucene:
1. **安装依赖**:
- 首先,你需要确保已经在Jython环境中安装了PyLucene。如果你还没有安装,可以在IDLE命令行里输入`import org.apache.lucene.*`,如果无误则说明已安装。
2. **初始化索引**:
```python
from org.apache.lucene.document import Document, TextField
from org.apache.lucene.index import DirectoryReader, IndexWriterConfig
from org.apache.lucene.store import SimpleFSDirectory
# 创建索引目录
directory = SimpleFSDirectory('path_to_your_index_directory')
# 设置配置
config = IndexWriterConfig(StandardAnalyzer())
# 创建IndexWriter
writer = IndexWriter(directory, config)
```
3. **添加文档**:
```python
doc = Document()
doc.add(TextField('field_name', 'document_content', Field.Store.YES))
writer.addDocument(doc)
```
4. **查询搜索**:
```python
query = QueryParser('field_name', StandardAnalyzer()).parse('your_search_query')
reader = DirectoryReader.open(directory)
searcher = IndexSearcher(reader)
results = searcher.search(query)
for result in results:
print(result.doc)
```
5. **关闭资源**:
```python
writer.close()
reader.close()
directory.close()
```
注意,这需要你在IDLE的交互模式下逐行运行,因为Python解释器无法像IDE那样自动管理文件和内存。另外,这个例子假设你正在使用Java API,如果是在纯Python环境下(如Elasticsearch),操作会有所不同。
阅读全文
相关推荐
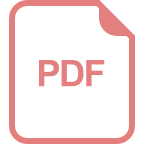
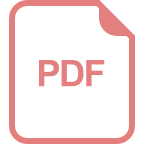
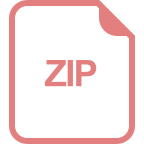
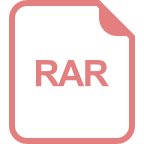
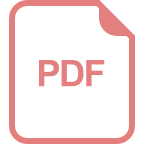
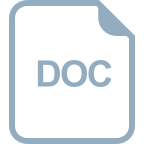
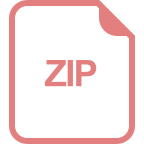
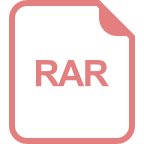
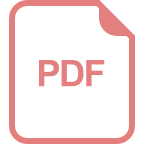
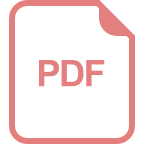
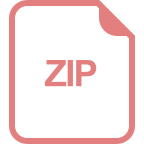
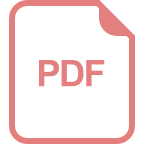
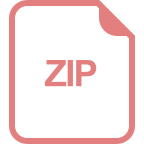