帮我用java语言解决基于优先队列式分支限界法解决装载问题,给出实例具体展示,给出具体实现代码
时间: 2023-12-31 08:07:14 浏览: 74
下面是基于优先队列式分支限界法解决装载问题的Java代码实现。
```java
import java.util.*;
public class KnapsackProblem {
static class Item implements Comparable<Item> {
int weight;
int value;
double density;
public Item(int weight, int value) {
this.weight = weight;
this.value = value;
this.density = (double) value / weight;
}
@Override
public int compareTo(Item other) {
if (this.density > other.density) {
return -1;
} else if (this.density < other.density) {
return 1;
} else {
return 0;
}
}
}
static class Node implements Comparable<Node> {
int level;
int profit;
int weight;
boolean[] include;
public Node(int level, int profit, int weight, boolean[] include) {
this.level = level;
this.profit = profit;
this.weight = weight;
this.include = include.clone();
}
@Override
public int compareTo(Node other) {
return Integer.compare(other.profit, this.profit);
}
}
public static int knapsack(int capacity, int[] weights, int[] values) {
int n = weights.length;
Item[] items = new Item[n];
for (int i = 0; i < n; i++) {
items[i] = new Item(weights[i], values[i]);
}
Arrays.sort(items);
PriorityQueue<Node> queue = new PriorityQueue<>();
boolean[] initialInclude = new boolean[n];
queue.offer(new Node(-1, 0, 0, initialInclude));
int maxProfit = 0;
while (!queue.isEmpty()) {
Node node = queue.poll();
if (node.level == n - 1) {
if (node.profit > maxProfit) {
maxProfit = node.profit;
}
continue;
}
int level = node.level + 1;
boolean[] include = node.include.clone();
include[level] = true;
int weight = node.weight + items[level].weight;
if (weight <= capacity) {
int profit = node.profit + items[level].value;
queue.offer(new Node(level, profit, weight, include));
}
int bound = bound(level, capacity, items, node);
if (bound > maxProfit) {
queue.offer(new Node(level, node.profit, node.weight, node.include.clone()));
}
}
return maxProfit;
}
private static int bound(int level, int capacity, Item[] items, Node node) {
int profit = node.profit;
int weight = node.weight;
for (int i = level + 1; i < items.length; i++) {
if (weight + items[i].weight <= capacity) {
weight += items[i].weight;
profit += items[i].value;
} else {
profit += (capacity - weight) * items[i].density;
break;
}
}
return profit;
}
public static void main(String[] args) {
int capacity = 50;
int[] weights = {10, 20, 30};
int[] values = {60, 100, 120};
int maxProfit = knapsack(capacity, weights, values);
System.out.println("Maximum profit is: " + maxProfit);
}
}
```
在上面的代码中,我们定义了两个内部类`Item`和`Node`。`Item`表示物品,包含重量、价值和价值密度;`Node`表示搜索树中的节点,包含当前层数、当前总价值、当前总重量和是否选择了每个物品。
我们首先将物品按照价值密度从大到小排序,然后使用优先队列保存搜索树中的节点。每次从队列中取出价值最大的节点,根据它的状态进行分支。如果选择当前物品能够放入背包,则生成一个新节点,加入队列中。如果选择当前物品不能放入背包,则计算当前节点的上界,如果该上界大于当前最优解,则生成一个新节点,加入队列中。
最后,我们输出得到的最大价值即可。
阅读全文
相关推荐
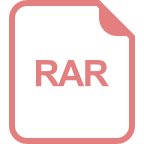
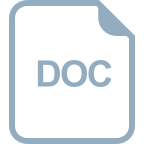
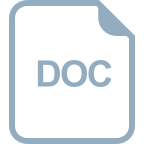
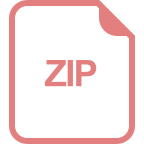
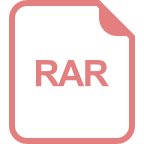
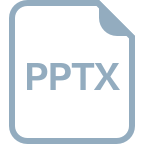
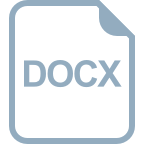
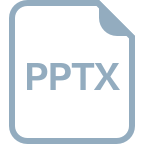
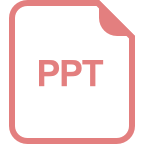
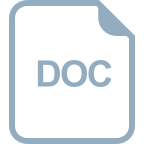
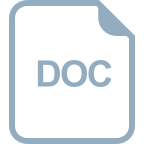
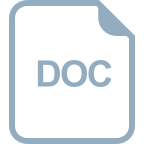
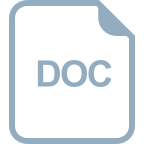
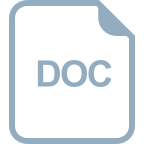
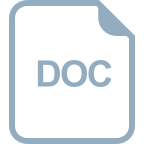
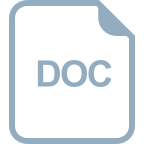
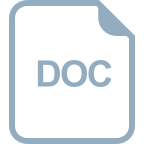