输入Excel文档,遗传算法解决TSP问题 matlab
时间: 2023-08-18 20:07:54 浏览: 123
在 MATLAB 中,也可以基于遗传算法来解决 TSP 问题。下面是一个简单的实现过程:
1. 读取 Excel 文件中的数据,得到城市的坐标信息。
2. 计算城市之间的距离矩阵。
3. 初始化遗传算法的参数,包括种群大小、交叉概率、变异概率等。
4. 初始化种群,将每个个体表示为一个城市的序列,并随机排列。
5. 评估每个个体的适应度,即计算其路径长度。
6. 进行选择、交叉和变异操作,生成新的种群。
7. 重复步骤 5 和 6,直到达到预定的迭代次数或找到满足要求的最优解。
下面是一个简单的 MATLAB 代码实现:
```matlab
% 读取 Excel 文件中的数据
data = xlsread('city_data.xlsx');
% 计算城市之间的距离矩阵
n_cities = length(data);
dist_matrix = zeros(n_cities);
for i = 1:n_cities
for j = i+1:n_cities
dist_matrix(i,j) = norm(data(i,:)-data(j,:));
dist_matrix(j,i) = dist_matrix(i,j);
end
end
% 初始化遗传算法参数
pop_size = 100;
n_generations = 1000;
crossover_prob = 0.8;
mutation_prob = 0.01;
% 初始化种群
pop = zeros(pop_size,n_cities);
for i = 1:pop_size
pop(i,:) = randperm(n_cities);
end
% 迭代
for g = 1:n_generations
% 评估适应度
fitness = zeros(pop_size,1);
for i = 1:pop_size
fitness(i) = evaluate_fitness(pop(i,:),dist_matrix);
end
% 选择、交叉和变异
new_pop = zeros(pop_size,n_cities);
for i = 1:pop_size
% 选择
p1 = tournament_selection(pop,fitness);
p2 = tournament_selection(pop,fitness);
% 交叉
if rand() < crossover_prob
offspring = crossover(p1,p2);
else
offspring = p1;
end
% 变异
if rand() < mutation_prob
offspring = mutation(offspring);
end
new_pop(i,:) = offspring;
end
% 更新种群
pop = new_pop;
end
% 输出最优解
[best_fitness,idx] = min(fitness);
best_path = pop(idx,:);
fprintf('最优路径长度:%.2f\n',best_fitness);
fprintf('最优路径:');
fprintf('%d ',best_path);
fprintf('\n');
% 计算适应度函数
function f = evaluate_fitness(path,dist_matrix)
f = 0;
n_cities = length(path);
for i = 1:n_cities-1
f = f + dist_matrix(path(i),path(i+1));
end
f = f + dist_matrix(path(n_cities),path(1));
end
% 锦标赛选择
function p = tournament_selection(pop,fitness,k)
if nargin < 3
k = 3;
end
idx = randi(size(pop,1),[k,1]);
[~,best_idx] = min(fitness(idx));
p = pop(idx(best_idx),:);
end
% 交叉
function offspring = crossover(p1,p2)
n_cities = length(p1);
idx = randi(n_cities-1);
offspring = zeros(1,n_cities);
offspring(1:idx) = p1(1:idx);
for i = idx+1:n_cities
if ~ismember(p2(i),offspring)
offspring(i) = p2(i);
else
remaining = setdiff(p1,offspring);
offspring(i) = remaining(1);
end
end
end
% 变异
function offspring = mutation(path)
n_cities = length(path);
idx = randperm(n_cities,2);
offspring = path;
offspring(idx(1)) = path(idx(2));
offspring(idx(2)) = path(idx(1));
end
```
其中,`evaluate_fitness` 函数用于计算适应度函数(即路径长度),`tournament_selection` 函数用于进行锦标赛选择,`crossover` 函数用于进行交叉操作,`mutation` 函数用于进行变异操作。
阅读全文
相关推荐
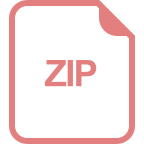
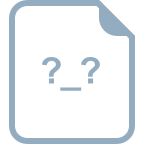



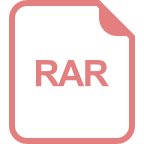
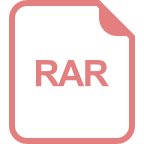
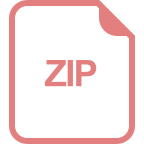
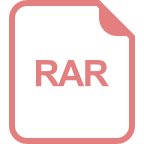
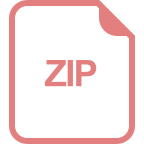
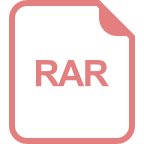
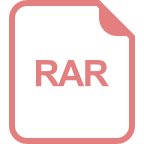
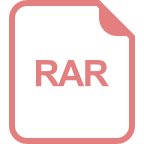