如何通过pycharm获取带token的API接口https://api.wintalent.cn/wt/api/3.0/auth/position/getPositionInformation数据
时间: 2023-10-05 14:14:30 浏览: 152
首先,你需要安装requests库。在PyCharm中,可以通过以下步骤安装:
1. 打开PyCharm。
2. 点击菜单栏的“File” -> “Settings”。
3. 在弹出的窗口中,选择“Project” -> “Project Interpreter”。
4. 点击右上角的“+”号,搜索并安装“requests”库。
接下来,你可以使用以下代码来获取API接口数据:
```
import requests
url = "https://api.wintalent.cn/wt/api/3.0/auth/position/getPositionInformation"
headers = {"Authorization": "Bearer YOUR_TOKEN_HERE"}
response = requests.get(url, headers=headers)
data = response.json()
print(data)
```
请注意将“YOUR_TOKEN_HERE”替换为你的实际token值。如果API接口需要其他参数,你可以将它们添加到请求中。例如:
```
payload = {"param1": "value1", "param2": "value2"}
response = requests.get(url, headers=headers, params=payload)
```
阅读全文
相关推荐
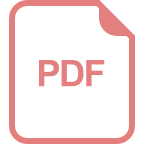
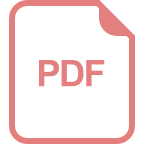
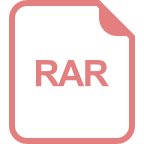
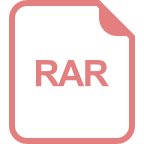
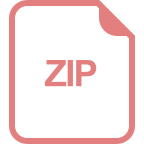
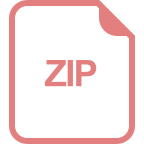
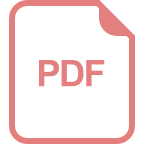
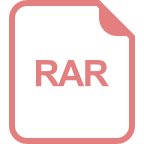
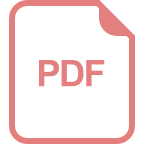
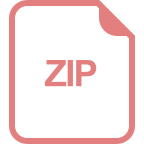
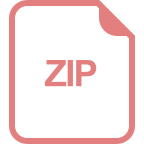
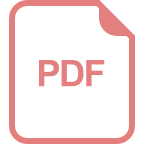
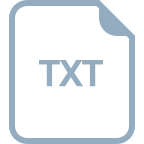
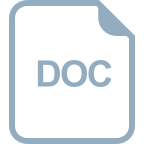
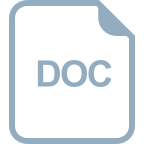
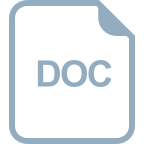