C语言写代码实验要求: 1.先序遍历创建二叉树; 2.中序线索化二叉树; 3.分别通过 找前驱 和 找后继 的方式,遍历中序线索二叉树。
时间: 2023-12-14 10:36:54 浏览: 70
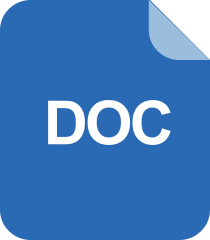
对所建立的二叉树分别进行先序、中序和后序遍历,并输出结果。

好的,以下是C语言的代码实现。
1. 先序遍历创建二叉树
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
char data;
struct node *left;
struct node *right;
} Node;
Node *create_tree() {
char ch;
scanf("%c", &ch);
if (ch == '#') {
return NULL;
}
Node *root = (Node *) malloc(sizeof(Node));
root->data = ch;
root->left = create_tree();
root->right = create_tree();
return root;
}
void pre_order_traversal(Node *root) {
if (root == NULL) {
return;
}
printf("%c ", root->data);
pre_order_traversal(root->left);
pre_order_traversal(root->right);
}
int main() {
printf("请输入先序遍历的字符序列:\n");
Node *root = create_tree();
printf("先序遍历结果:\n");
pre_order_traversal(root);
return 0;
}
```
2. 中序线索化二叉树
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
char data;
struct node *left;
struct node *right;
int ltag; // 左线索标记
int rtag; // 右线索标记
} Node;
Node *create_tree() {
char ch;
scanf("%c", &ch);
if (ch == '#') {
return NULL;
}
Node *root = (Node *) malloc(sizeof(Node));
root->data = ch;
root->left = create_tree();
root->right = create_tree();
root->ltag = 0;
root->rtag = 0;
return root;
}
Node *pre_node(Node *p) {
Node *q = p->left;
while (q->rtag == 0) {
q = q->right;
}
return q;
}
Node *next_node(Node *p) {
Node *q = p->right;
if (p->rtag == 0) {
return pre_node(q);
}
return q;
}
void in_threading(Node *p, Node **pre) {
if (p == NULL) {
return;
}
in_threading(p->left, pre);
if (p->left == NULL) {
p->ltag = 1;
p->left = *pre;
}
if (*pre != NULL && (*pre)->right == NULL) {
(*pre)->rtag = 1;
(*pre)->right = p;
}
*pre = p;
in_threading(p->right, pre);
}
void in_order_traversal(Node *root) {
Node *p = root;
while (p->ltag == 0) {
p = p->left;
}
while (p != NULL) {
printf("%c ", p->data);
p = next_node(p);
}
}
int main() {
printf("请输入先序遍历的字符序列:\n");
Node *root = create_tree();
Node *pre = NULL;
in_threading(root, &pre);
printf("中序遍历结果:\n");
in_order_traversal(root);
return 0;
}
```
3. 找前驱和找后继的方式遍历中序线索二叉树
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
char data;
struct node *left;
struct node *right;
int ltag; // 左线索标记
int rtag; // 右线索标记
} Node;
Node *create_tree() {
char ch;
scanf("%c", &ch);
if (ch == '#') {
return NULL;
}
Node *root = (Node *) malloc(sizeof(Node));
root->data = ch;
root->left = create_tree();
root->right = create_tree();
root->ltag = 0;
root->rtag = 0;
return root;
}
Node *pre_node(Node *p) {
Node *q = p->left;
while (q->rtag == 0) {
q = q->right;
}
return q;
}
Node *next_node(Node *p) {
Node *q = p->right;
if (p->rtag == 0) {
return pre_node(q);
}
return q;
}
void in_threading(Node *p, Node **pre) {
if (p == NULL) {
return;
}
in_threading(p->left, pre);
if (p->left == NULL) {
p->ltag = 1;
p->left = *pre;
}
if (*pre != NULL && (*pre)->right == NULL) {
(*pre)->rtag = 1;
(*pre)->right = p;
}
*pre = p;
in_threading(p->right, pre);
}
void in_order_traversal(Node *root) {
Node *p = root;
while (p->ltag == 0) {
p = p->left;
}
while (p != NULL) {
printf("%c ", p->data);
p = next_node(p);
}
}
void pre_order_traversal(Node *root) {
if (root == NULL) {
return;
}
printf("%c ", root->data);
if (root->ltag == 0) {
pre_order_traversal(root->left);
}
if (root->rtag == 0) {
pre_order_traversal(root->right);
}
}
int main() {
printf("请输入先序遍历的字符序列:\n");
Node *root = create_tree();
Node *pre = NULL;
in_threading(root, &pre);
printf("中序遍历结果:\n");
in_order_traversal(root);
printf("\n先序遍历结果:\n");
pre_order_traversal(root);
return 0;
}
```
阅读全文
相关推荐




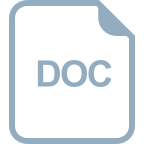


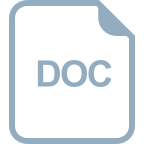


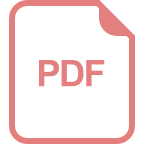