利用JAVA,做一个除法运算的异常处理。需求描述∶编写一个简单的程序,完成两个整数的除法运算,同时处理以下异常情况∶除数为零时,抛出 算术异常,输入的不是整数时,抛出 输入格式异常
时间: 2024-12-17 16:26:14 浏览: 18
在Java中,我们可以使用`try-catch`结构来捕获并处理异常。对于除法运算,如果遇到除数为零的情况,我们会抛出`ArithmeticException`,而输入非整数的情况可以使用`InputMismatchException`。以下是满足您需求的代码示例:
```java
import java.util.Scanner;
public class DivisionWithExceptions {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
try {
System.out.print("Enter the first number: ");
int num1 = scanner.nextInt();
if (!scanner.hasNextInt()) { // 检查是否有下一个整数
throw new InputMismatchException("Invalid input format, only integers are allowed.");
}
System.out.print("Enter the second number (for division): ");
int num2 = scanner.nextInt();
if (num2 == 0) {
throw new ArithmeticException("Division by zero is not allowed.");
}
int result = num1 / num2;
System.out.println("Result: " + result);
} catch (InputMismatchException ime) {
System.out.println("Error: " + ime.getMessage());
scanner.next(); // 清空输入缓冲区,避免后续干扰
} catch (ArithmeticException ae) {
System.out.println("Error: " + ae.getMessage());
} finally {
scanner.close(); // 关闭Scanner资源
}
}
}
```
在这个程序中,我们首先读取用户输入,然后检查输入是否是整数以及除数是否为零。如果遇到不符合条件的情况,就抛出相应的异常,并在对应的`catch`块中处理。
阅读全文
相关推荐
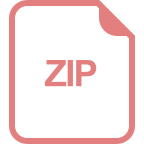
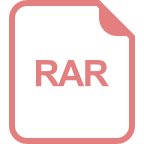
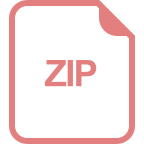








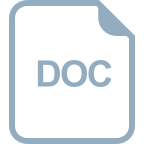
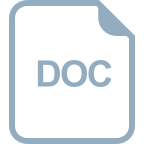





