C++编写一个实验程序,输入一个正整数n(n>5),随机产生n个0~99的整数,采用递归算法求其中的最大整数和次大整数。
时间: 2024-12-19 07:13:54 浏览: 18
在C++中,你可以使用递归来寻找一组数字中的最大值和次大值。这里是一个简单的示例程序,它首先生成指定数量的随机整数,然后通过递归找到最大值和次大值:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
#include <cstdlib>
#include <ctime>
// 辅助函数,用于找出给定数组中的最大值
int findMax(std::vector<int>& arr, int n, int currentMax, int secondMax) {
if (n == 1) {
// 当只有一个元素时,更新当前最大值和次大值
return currentMax = std::max(currentMax, arr[0]);
} else {
int temp = arr[n - 1];
// 更新数组,并继续查找剩余部分的最大值
arr[n - 1] = arr[0];
arr[0] = temp;
int nextMax = findMax(arr, n - 1, currentMax, secondMax);
// 返回当前找到的最大值和次大值
return nextMax > currentMax ? nextMax : currentMax;
}
}
// 主函数
int main() {
srand(time(0)); // 设置随机数种子
int n;
std::cout << "请输入一个正整数n(n > 5): ";
std::cin >> n;
if (n <= 5) {
std::cerr << "n需要大于5。\n";
return 1; // 错误处理
}
std::vector<int> numbers(n);
for (int i = 0; i < n; ++i) {
numbers[i] = rand() % 100; // 生成0到99之间的随机整数
}
int maxNum = findMax(numbers, n, INT_MIN, INT_MIN); // 初始化为负极大值
std::cout << "最大值: " << maxNum << "\n";
// 因为findMax只返回最大值,我们需要再次遍历数组找到次大值
int secondMax = INT_MIN;
for (int num : numbers) {
secondMax = std::max(secondMax, num);
}
std::cout << "次大值: " << secondMax << "\n";
return 0;
}
```
阅读全文
相关推荐
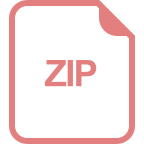
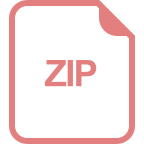

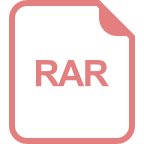
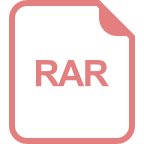
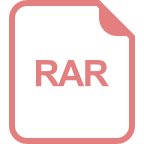
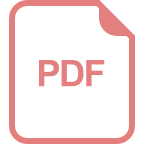
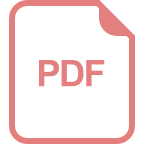
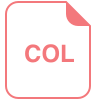


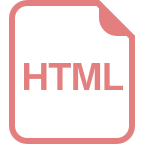