使用【c语言】写【讲用户输入到任意个整数入栈,然后按升序对该整数栈进行排序,最小元素位于栈顶。最多只能使用一个额外的栈存放临时数据。并输出栈排序过程】的代码
时间: 2024-10-21 19:17:31 浏览: 30
在C语言中,我们可以使用两个栈来完成这个任务。一个栈用于存储用户的输入,另一个栈作为辅助栈用于排序。以下是实现这一功能的步骤和代码:
首先,我们需要创建两个栈结构体(这里假设为`struct Stack`),包含一个数组和两个指针。
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int* arr;
size_t top;
size_t capacity;
} Stack;
Stack create_stack(int capacity) {
Stack s;
s.arr = (int*)malloc(capacity * sizeof(int));
if (!s.arr) {
perror("Memory allocation failed");
exit(1);
}
s.top = -1;
s.capacity = capacity;
return s;
}
void push(Stack* s, int value) {
if (s->top == s->capacity - 1) {
printf("Stack overflow\n");
return;
}
s->arr[++s->top] = value;
}
```
接下来,读取用户输入并将值压入第一个栈:
```c
void input_and_push(Stack* in, int n) {
for (int i = 0; i < n; ++i) {
int num;
scanf("%d", &num);
push(in, num);
}
}
```
接着,我们使用第二个栈对输入栈进行升序排序。这里通过不断交换第一个栈顶元素(当前最大值)和辅助栈顶元素(次大值),直到辅助栈为空:
```c
void sort(Stack* in, Stack* aux) {
while (in->top > 0 && aux->top > 0) {
if (in->arr[aux->top] < in->arr[in->top]) {
int temp = in->arr[aux->top];
in->arr[aux->top] = in->arr[in->top];
in->arr[in->top] = temp;
--aux->top;
} else {
--in->top;
}
}
}
```
最后,遍历并打印已排序的栈:
```c
void print_sorted(Stack* s) {
for (size_t i = 0; i <= s->top; ++i) {
printf("%d ", s->arr[i]);
}
printf("\n");
}
```
主函数将所有部分组合起来:
```c
int main() {
int n;
printf("Enter the number of integers: ");
scanf("%d", &n);
Stack in = create_stack(n);
input_and_push(&in, n);
Stack aux = create_stack(n);
sort(&in, &aux);
print_sorted(&in);
free(in.arr); // Don't forget to free memory when done
free(aux.arr);
return 0;
}
```
运行此程序时,输入一些整数,它会按照升序顺序将它们放入栈,并打印出排序后的结果。注意,在实际操作中,需要处理边界情况和错误处理。
阅读全文
相关推荐
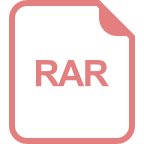
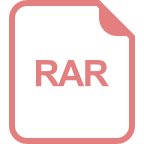
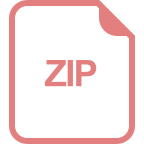
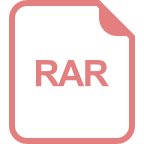
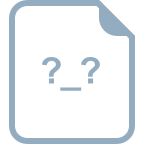
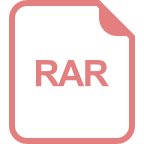
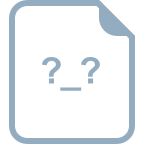
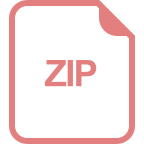
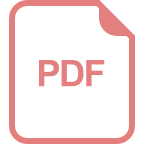
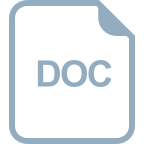
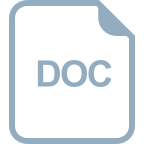
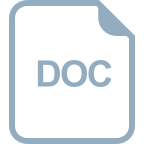
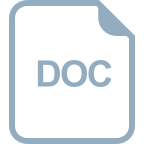