File "C:\Users\lenovo\PycharmProjects\pythonProject1\PythonProject.py", line 399 labelImgPath = 'F:\huya\Python\xiaohui.jpg' ^ SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 14-15: truncated \xXX escape
时间: 2023-07-19 15:32:17 浏览: 143
这个错误提示是因为在你的代码中,路径中的反斜杠(\)被解释成了转义字符。你可以尝试在字符串前面加上r或者把反斜杠换成正斜杠(/)来避免这个问题。例如:
使用r:
```python
labelImgPath = r'F:\huya\Python\xiaohui.jpg'
```
使用正斜杠:
```python
labelImgPath = 'F:/huya/Python/xiaohui.jpg'
```
这样就可以避免这个错误。
相关问题
import requests from lxml import etree import os from urllib.request import urlretrieve import sys url='https://www.huya.com/g' headers={'User-Agent':'Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/49.0.2623.112 Safari/537.36'} response=requests.get(url,headers=headers).content.decode() html=etree.HTML(response) req=html.xpath('//li[@class="g-gameCard-item"]//a//@data-gid') names=html.xpath('//li[@class="g-gameCard-item"]//a//p/text()') i=0 mylog=open('关键字大全.log',mode='a',encoding='utf-8') print(' -----------查找关键字大全-----------',file=mylog) #这里只是为了查看关键字美观而已,保存到记事本里 for re,name in zip(req,names): print('关键字:%s 查找id号:%s'%(name,re),end=' ',file=mylog) i+=1 if i==5: print('\n',file=mylog) i=0 mylog.close() name_1=input('\n请输入查找关键字:') for re,name in zip(req,names): if name_1==name: break if name_1!=name: print('没有你想查找的关键字') else: url='https://www.huya.com/g/{}#tag0'.format(re) data=requests.get(url,headers=headers).content.decode() html=etree.HTML(data) #链接 link_list=html.xpath('//a/img[@class="pic"]/@data-original') #名字 names_1_list=html.xpath('//span[@class="avatar fl"]//img/@title') # 文件名字 os.mkdir(name) for link, names_1 in zip(link_list, names_1_list): # 获取链接 link = link.split('?')[0] try: # 保存数据 urlretrieve(link, name + '/' + names_1 + '.jpg') print(names_1 + '100%') except: pass
这段代码是一个Python爬虫程序,用于从虎牙直播网站上爬取游戏直播分类下的主播头像,保存到本地文件夹中。具体实现过程如下:
1. 导入requests、lxml、os和urllib库,用于发送http请求、解析html文档、创建文件夹和下载图片。
2. 设置headers,伪装成浏览器的请求头,避免被服务器识别为爬虫。
3. 发送http请求,获取游戏直播分类下的html文档。
4. 使用xpath解析html文档,获取所有游戏id和游戏名字。
5. 将关键字和对应的id保存到本地记事本中,以便后续查看。
6. 等待用户输入要查找的关键字,根据关键字查找对应的游戏id。
7. 根据游戏id构造新的url,重新发送http请求,获取游戏主播列表的html文档。
8. 使用xpath解析html文档,获取所有主播头像的链接和主播名字。
9. 根据主播名字创建文件夹,遍历所有主播头像链接,下载头像并保存到对应的文件夹中。
需要注意的是,这段代码仅供学习和参考使用,对于未经授权的爬取行为,请勿使用。同时,爬取过程中应当遵守相关法律法规和网站的使用协议,不得侵犯他人的隐私和知识产权。
https://www.huya.com/ 写个爬虫程序
### 回答1:
好的,这里是一个简单的 Python 爬虫程序,用于爬取虎牙直播(https://www.huya.com/)的热门主播列表:
```python
import requests
from bs4 import BeautifulSoup
# 发送 HTTP 请求
url = 'https://www.huya.com/g'
response = requests.get(url)
# 解析 HTML 页面
soup = BeautifulSoup(response.text, 'html.parser')
# 获取热门主播列表
anchors = []
for anchor in soup.find_all('a', {'class': 'avatar fl'}):
name = anchor.find('i', {'class': 'nick'}).get_text()
viewers = anchor.find('i', {'class': 'js-num'}).get_text()
anchors.append({'name': name, 'viewers': viewers})
# 输出结果
for anchor in anchors:
print('主播:{},观众人数:{}'.format(anchor['name'], anchor['viewers']))
```
这个程序首先发送 HTTP 请求,获取虎牙直播首页的 HTML 页面。然后使用 BeautifulSoup 对页面进行解析,找到所有热门主播的标签和属性,并将它们保存到一个列表中。最后,程序输出每个主播的名称和观众人数。
需要注意的是,这个程序只是一个简单的示例,爬取网站数据时需要注意遵守网站的使用协议和法律法规,并且不要过度访问网站,以免给网站带来不必要的负担。
### 回答2:
爬虫程序通常用于从互联网上获取数据,本例中我们将使用Python编写一个简单的爬虫程序来爬取 https://www.huya.com/ 网站上的数据。
首先,我们需要导入相关的库:requests用于发送HTTP请求,BeautifulSoup用于解析HTML,和re用于正则表达式。
接下来,我们发送一个GET请求到 https://www.huya.com/ 网站,并获得响应的HTML内容。
然后,我们使用BeautifulSoup解析HTML内容,并通过查找相关的HTML标签和类名,提取出我们需要的数据。
最后,我们打印或保存提取出来的数据。
下面是一个简单的示例代码:
```python
import requests
from bs4 import BeautifulSoup
import re
# 发送GET请求并获得响应
response = requests.get("https://www.huya.com/")
html_content = response.text
# 使用BeautifulSoup解析HTML内容
soup = BeautifulSoup(html_content, 'html.parser')
# 通过查找HTML标签和类名提取数据
data = soup.find_all('a', class_='game-info-item-title')
# 打印或保存提取出的数据
for item in data:
print(item.get_text())
```
这个爬虫程序将会从 https://www.huya.com/ 网站上提取出所有游戏信息的标题,并打印出来。你可以根据自己的需求进行进一步的数据处理和保存。
阅读全文
相关推荐
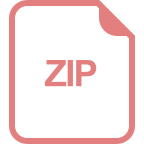
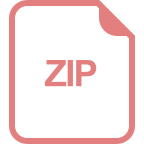
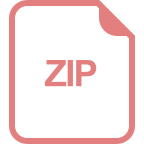
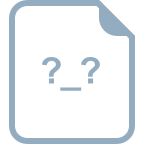
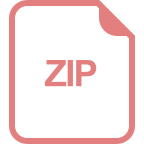
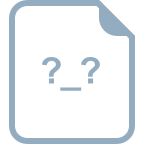
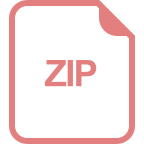
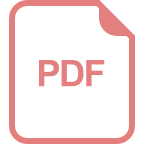
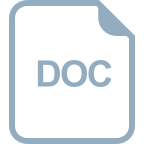



