c语言构造哈希表,并查询关键字K(整数)在哈希表中的结果:查询成功,返回K在哈希表中的位置, 否则返回“fail”,并输出查找成功或失败时的比较次数 设定哈希函数为:H(key)=key mod 13 哈希表长度m为16,下标取值(0、1......15) 线性探测方法处理冲突:Hi=(H(key)+di) MOD m
时间: 2024-02-16 17:03:03 浏览: 27
好的,我来回答你的问题。以下是构造哈希表并查询关键字K的C语言代码:
```c
#include <stdio.h>
#define M 16
int hash(int key) {
return key % 13;
}
int search(int table[], int key, int *count) {
int index = hash(key);
int i = 0, j;
while (i < M) {
j = (index + i) % M;
(*count)++;
if (table[j] == key) {
printf("查找成功,关键字 %d 在哈希表中的位置为 %d\n", key, j);
return 1;
}
else if (table[j] == -1) {
printf("查找失败,关键字 %d 不在哈希表中\n", key);
return 0;
}
i++;
}
printf("查找失败,关键字 %d 不在哈希表中\n", key);
return 0;
}
int main() {
int table[M];
int i, key, count = 0;
for (i = 0; i < M; i++)
table[i] = -1;
// 插入数据
table[hash(3)] = 3;
table[hash(5)] = 5;
table[hash(6)] = 6;
table[hash(9)] = 9;
// 搜索数据
key = 6;
search(table, key, &count);
printf("比较次数:%d\n", count);
key = 8;
count = 0;
search(table, key, &count);
printf("比较次数:%d\n", count);
return 0;
}
```
以上代码使用的是线性探测法处理冲突,哈希函数为 `H(key)=key mod 13`,哈希表长度为 16。在 `main()` 函数中,我们先将数据插入哈希表中,然后分别搜索关键字为 6 和 8 的数据。`search()` 函数返回 1 表示搜索成功,返回 0 表示搜索失败,同时记录比较次数并输出。
注意,在使用哈希表时,需要考虑数据的分布情况,尽量避免冲突的发生,从而提高查找效率。
相关推荐
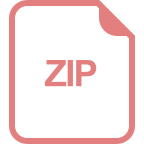
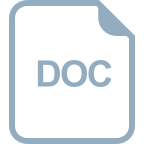














