tensorflow实现LSTM
时间: 2023-11-10 07:51:29 浏览: 88
LSTM(长短期记忆网络)是一种常用于处理序列数据的深度学习模型。下面是在TensorFlow中实现LSTM的示例代码:
```
import tensorflow as tf
# 定义输入数据的形状和大小
input_dim = 100
sequence_length = 50
batch_size = 32
# 定义LSTM的参数
num_hidden = 64
num_layers = 2
# 定义输入和输出的占位符
inputs = tf.placeholder(tf.float32, shape=[None, sequence_length, input_dim], name='inputs')
targets = tf.placeholder(tf.float32, shape=[None, 1], name='targets')
# 定义LSTM层
lstm_cell = tf.nn.rnn_cell.BasicLSTMCell(num_hidden)
lstm_cell = tf.nn.rnn_cell.MultiRNNCell([lstm_cell] * num_layers)
outputs, final_state = tf.nn.dynamic_rnn(lstm_cell, inputs, dtype=tf.float32)
# 定义输出层
last_output = outputs[:, -1, :]
weights = tf.Variable(tf.truncated_normal([num_hidden, 1], stddev=0.1))
bias = tf.Variable(tf.constant(0.1, shape=[1]))
predictions = tf.matmul(last_output, weights) + bias
# 定义损失函数和优化器
loss = tf.reduce_mean(tf.square(predictions - targets))
optimizer = tf.train.AdamOptimizer().minimize(loss)
# 训练模型
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for i in range(num_iterations):
batch_inputs, batch_targets = get_batch(batch_size)
feed_dict = {inputs: batch_inputs, targets: batch_targets}
_, batch_loss = sess.run([optimizer, loss], feed_dict=feed_dict)
if i % 100 == 0:
print('Iteration', i, 'Loss', batch_loss)
```
在这个示例中,我们首先定义了输入数据的形状和大小,然后定义了LSTM的参数,包括隐藏层的大小和LSTM层的数量。接下来,我们定义了输入和输出的占位符,以及LSTM层和输出层。最后,我们定义了损失函数和优化器,并使用训练数据训练模型。
需要注意的是,这只是一个简单的示例,实际使用中还需要根据数据和任务特点进行调整和优化。
阅读全文
相关推荐

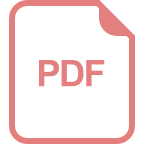
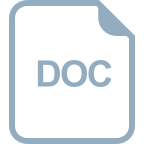




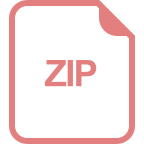
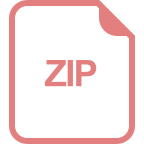
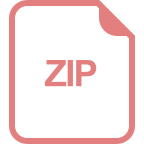
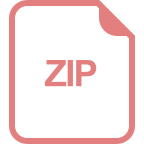
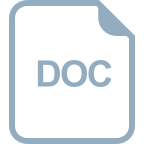
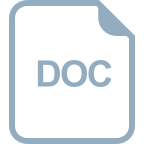
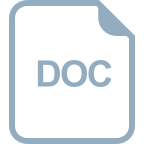

