用c语言设计企业员工管理系统程序,要求先以管理员身份登录(自己设定进入密码)。输入密码正确后,录入某企业员工(假定不超过 50人)的工资。密码限定最多输入三次,三次不成功退出系统。将员工工资按照从高到低顺序排序;假定工资超过3500 元的,超出部分按 5%缴税,给出该企业上缴工资税金总数,并统计缴税员工占总员工的比例
时间: 2023-07-16 12:16:06 浏览: 46
很好,这是一个比较典型的企业员工管理系统的需求,我可以给你提供一些思路和代码实现。
1. 设计管理员登录功能
首先,我们需要设计管理员登录功能,可以设置一个固定的密码,最多输入三次机会。如果输入正确,就可以进入员工工资录入界面;如果输入错误三次,就退出程序。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_ATTEMPTS 3 // 最多尝试次数
#define PASSWORD "123456" // 管理员密码
int main()
{
char password[20];
int attempts = 0;
printf("Please enter password to login as administrator:\n");
while (attempts < MAX_ATTEMPTS) {
scanf("%s", password);
if (strcmp(password, PASSWORD) == 0) {
printf("Login successful!\n");
break;
} else {
attempts++;
printf("Incorrect password, please try again.\n");
}
}
if (attempts == MAX_ATTEMPTS) {
printf("Too many attempts, exit program.\n");
exit(1);
}
// TODO: 进入员工工资录入界面
return 0;
}
```
2. 录入员工工资
登录成功后,我们就可以进入员工工资录入界面。由于假设员工不超过50人,我们可以使用一个数组来存储员工的工资信息。
```c
#define MAX_EMPLOYEES 50 // 最多员工数
struct Employee {
char name[20];
float salary;
};
struct Employee employees[MAX_EMPLOYEES];
int count = 0; // 当前员工数
void input_employee_info()
{
printf("Please input employee information (name salary):\n");
printf("(Press Enter to exit input)\n");
while (count < MAX_EMPLOYEES) {
scanf("%s", employees[count].name);
if (strlen(employees[count].name) == 0) {
break;
}
scanf("%f", &employees[count].salary);
count++;
}
}
```
3. 排序员工工资
录入员工工资后,我们需要按照从高到低的顺序对员工工资进行排序。可以使用快速排序算法来实现。
```c
void swap(struct Employee *a, struct Employee *b)
{
struct Employee tmp = *a;
*a = *b;
*b = tmp;
}
int partition(struct Employee arr[], int low, int high)
{
float pivot = arr[high].salary;
int i = low - 1;
for (int j = low; j < high; j++) {
if (arr[j].salary > pivot) {
i++;
swap(&arr[i], &arr[j]);
}
}
swap(&arr[i+1], &arr[high]);
return i + 1;
}
void quicksort(struct Employee arr[], int low, int high)
{
if (low < high) {
int pi = partition(arr, low, high);
quicksort(arr, low, pi - 1);
quicksort(arr, pi + 1, high);
}
}
void sort_employees()
{
quicksort(employees, 0, count - 1);
}
```
4. 缴税及统计
按照工资排序后,我们就可以统计缴税信息了。根据题目要求,工资超过3500元的部分需要缴税,缴税比例为5%。统计缴税员工占总员工的比例时,可以使用一个计数器来记录缴税员工的数量。
```c
void calculate_tax()
{
float total_tax = 0;
int tax_count = 0;
printf("Employee salary information (sorted):\n");
for (int i = 0; i < count; i++) {
printf("%s: %.2f\n", employees[i].name, employees[i].salary);
if (employees[i].salary > 3500) {
float tax = (employees[i].salary - 3500) * 0.05;
total_tax += tax;
tax_count++;
}
}
printf("Total tax: %.2f\n", total_tax);
printf("Tax employee ratio: %.2f%%\n", (float)tax_count / count * 100);
}
```
5. 完整代码
最后,我们把以上几个部分整合起来,得到完整的企业员工管理系统程序。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_ATTEMPTS 3 // 最多尝试次数
#define PASSWORD "123456" // 管理员密码
#define MAX_EMPLOYEES 50 // 最多员工数
struct Employee {
char name[20];
float salary;
};
struct Employee employees[MAX_EMPLOYEES];
int count = 0; // 当前员工数
void input_employee_info()
{
printf("Please input employee information (name salary):\n");
printf("(Press Enter to exit input)\n");
while (count < MAX_EMPLOYEES) {
scanf("%s", employees[count].name);
if (strlen(employees[count].name) == 0) {
break;
}
scanf("%f", &employees[count].salary);
count++;
}
}
void swap(struct Employee *a, struct Employee *b)
{
struct Employee tmp = *a;
*a = *b;
*b = tmp;
}
int partition(struct Employee arr[], int low, int high)
{
float pivot = arr[high].salary;
int i = low - 1;
for (int j = low; j < high; j++) {
if (arr[j].salary > pivot) {
i++;
swap(&arr[i], &arr[j]);
}
}
swap(&arr[i+1], &arr[high]);
return i + 1;
}
void quicksort(struct Employee arr[], int low, int high)
{
if (low < high) {
int pi = partition(arr, low, high);
quicksort(arr, low, pi - 1);
quicksort(arr, pi + 1, high);
}
}
void sort_employees()
{
quicksort(employees, 0, count - 1);
}
void calculate_tax()
{
float total_tax = 0;
int tax_count = 0;
printf("Employee salary information (sorted):\n");
for (int i = 0; i < count; i++) {
printf("%s: %.2f\n", employees[i].name, employees[i].salary);
if (employees[i].salary > 3500) {
float tax = (employees[i].salary - 3500) * 0.05;
total_tax += tax;
tax_count++;
}
}
printf("Total tax: %.2f\n", total_tax);
printf("Tax employee ratio: %.2f%%\n", (float)tax_count / count * 100);
}
int main()
{
char password[20];
int attempts = 0;
printf("Please enter password to login as administrator:\n");
while (attempts < MAX_ATTEMPTS) {
scanf("%s", password);
if (strcmp(password, PASSWORD) == 0) {
printf("Login successful!\n");
break;
} else {
attempts++;
printf("Incorrect password, please try again.\n");
}
}
if (attempts == MAX_ATTEMPTS) {
printf("Too many attempts, exit program.\n");
exit(1);
}
input_employee_info();
sort_employees();
calculate_tax();
return 0;
}
```
相关推荐
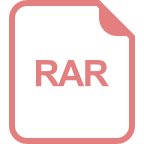














