give me an Python example using clamped cubic spline,do not use Scipy
时间: 2024-05-12 19:18:08 浏览: 64
Sure, here's an example Python code for clamped cubic spline without using Scipy:
```
import numpy as np
def clamped_cubic_spline(x, y, dydx0, dydxn):
n = len(x)
h = np.diff(x)
alpha = np.zeros(n)
l = np.ones(n)
u = np.zeros(n)
z = np.zeros(n)
for i in range(1, n - 1):
alpha[i] = (3 / h[i]) * (y[i+1] - y[i]) - (3 / h[i-1]) * (y[i] - y[i-1])
l[i] = 2 * (x[i+1] - x[i-1]) - h[i-1] * u[i-1]
u[i] = h[i] / l[i]
z[i] = (alpha[i] - h[i-1] * z[i-1]) / l[i]
b = np.zeros(n)
c = np.zeros(n)
d = np.zeros(n)
l[n-1] = 1
z[n-1] = 0
c[n-1] = 0
for j in range(n-2, -1, -1):
c[j] = z[j] - u[j] * c[j+1]
b[j] = (y[j+1] - y[j]) / h[j] - h[j] * (c[j+1] + 2 * c[j]) / 3
d[j] = (c[j+1] - c[j]) / (3 * h[j])
a = y[:-1]
b = b[:-1]
c = c[:-1]
d = d[:-1]
return a, b, c, d
x = np.array([0, 1, 2, 3, 4])
y = np.array([1, 2, 3, 2, 1])
dydx0 = 0
dydxn = 0
a, b, c, d = clamped_cubic_spline(x, y, dydx0, dydxn)
print("a =", a)
print("b =", b)
print("c =", c)
print("d =", d)
```
This code takes in arrays of `x` and `y` values, as well as the derivative values `dydx0` and `dydxn` at the endpoints. It outputs the coefficients `a`, `b`, `c`, and `d` of the clamped cubic spline. Note that this implementation assumes that the `x` values are evenly spaced.
阅读全文
相关推荐
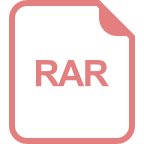
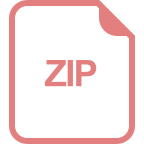
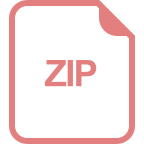
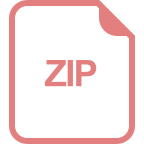
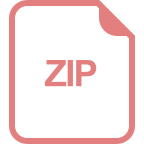
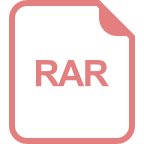
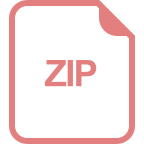
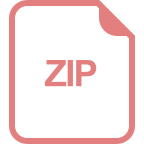
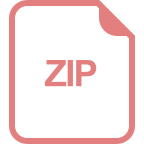
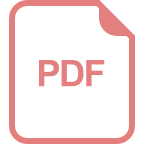
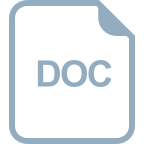

