给一个C语言摄氏温度习题的例子
时间: 2024-10-05 15:01:25 浏览: 27
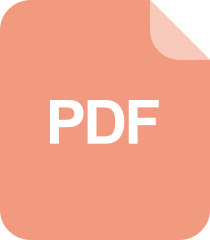
pta题库答案c语言 - C语言PTA平台习题与答案
在C语言中,我们可以编写一个简单的程序来将摄氏温度转换为华氏温度,这是多态的一个简单应用场景。由于C语言本身并不支持面向对象编程的多态,这里我们将使用函数作为"方法",来展示如何处理不同的温度单位。下面是一个例子:
```c
#include <stdio.h>
// 摄氏到华氏的转换函数
double celsius_to_fahrenheit(double celsius) {
return (celsius * 9.0 / 5.0) + 32;
}
// 华氏到摄氏的转换函数
double fahrenheit_to_celsius(double fahrenheit) {
return (fahrenheit - 32) * 5.0 / 9.0;
}
int main() {
double temperature; // 用户输入的温度
char unit; // 用户选择的温度单位
printf("请输入温度值: ");
scanf("%lf", &temperature);
printf("请输入温度单位('C' 或 'F'): ");
scanf(" %c", &unit); // 注意添加空格以避免读入前一字符
if (unit == 'C') {
double converted_temp = celsius_to_fahrenheit(temperature);
printf("%.2lf 摄氏度等于 %.2lf 华氏度.\n", temperature, converted_temp);
} else if (unit == 'F') {
double converted_temp = fahrenheit_to_celsius(temperature);
printf("%.2lf 华氏度等于 %.2lf 摄氏度.\n", temperature, converted_temp);
} else {
printf("无效的温度单位.\n");
}
return 0;
}
```
在这个例子中,`celsius_to_fahrenheit` 和 `fahrenheit_to_celsius` 可以看作是处理温度转换的"方法",它们根据用户输入的单位动态选择相应的转换。虽然这不是严格意义上的多态,但展示了函数在不同场景下的应用。
阅读全文
相关推荐
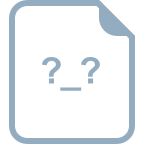
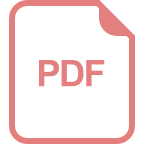
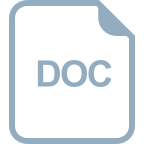
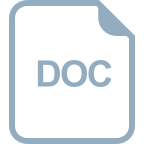
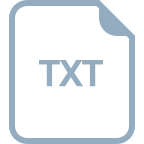
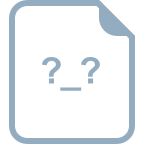
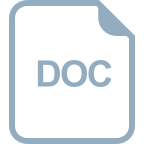
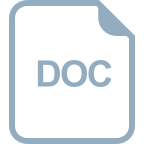
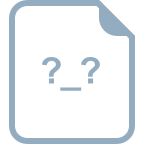
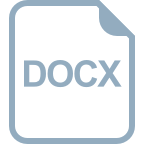
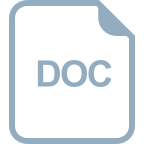
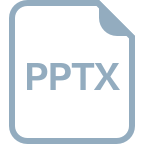
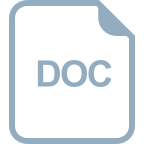
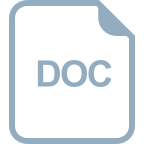
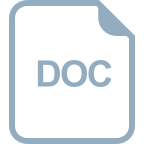
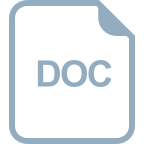
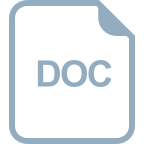