结构型设计模式实训,利用外观模式实现文件加密功能。
时间: 2024-02-23 08:31:53 浏览: 77
好的,下面是一个简单的文件加密功能的外观模式实现:
首先我们需要定义一个加密器 Encryptor,它负责对文件进行加密操作:
```python
class Encryptor:
def encrypt(self, file_path):
print(f"Encrypting file: {file_path}")
# 实现加密操作
```
接下来我们定义一个文件操作器 FileManager,它负责读取和写入文件:
```python
class FileManager:
def read_file(self, file_path):
print(f"Reading file: {file_path}")
# 实现读取文件操作
def write_file(self, file_path, data):
print(f"Writing file: {file_path}")
# 实现写入文件操作
```
最后我们定义一个文件加密外观 Facade,它将文件操作和加密操作组合起来,提供一个简单的接口给用户使用:
```python
class FileEncryptorFacade:
def __init__(self):
self.encryptor = Encryptor()
self.file_manager = FileManager()
def encrypt_file(self, file_path):
data = self.file_manager.read_file(file_path)
encrypted_data = self.encryptor.encrypt(file_path)
self.file_manager.write_file(file_path, encrypted_data)
print(f"File {file_path} encrypted successfully.")
```
这样,用户只需要调用 FileEncryptorFacade 的 encrypt_file 方法,就可以对指定的文件进行加密操作了:
```python
facade = FileEncryptorFacade()
facade.encrypt_file("path/to/file")
```
这样就完成了一个简单的文件加密外观模式实现。
阅读全文
相关推荐
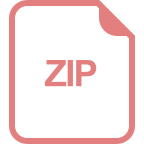
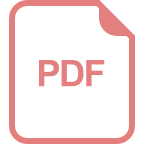
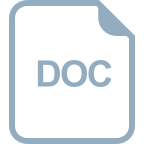
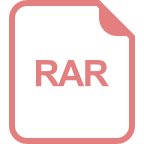
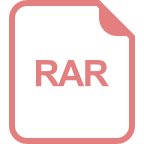
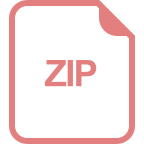
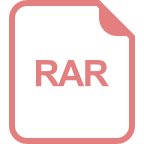
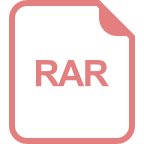
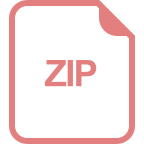
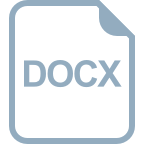
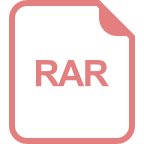
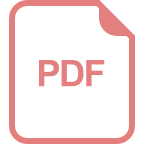
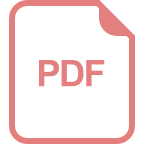
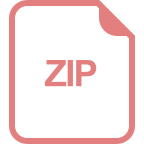
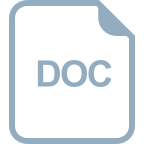