java中jsoup爬取网页中存在分页的数据如何爬取
时间: 2024-01-14 22:03:12 浏览: 123
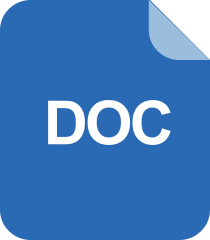
实例讲解Java语言利用搜索引擎收集网址
使用Jsoup爬取分页数据的步骤如下:
1. 定义要爬取的网页地址和需要爬取的内容。
2. 使用Jsoup连接网页,获取网页内容。
3. 使用Jsoup解析网页内容,提取需要的数据。
4. 如果网页中存在多页数据,使用循环遍历所有网页,重复步骤2和步骤3。
5. 将提取的数据保存到本地或数据库中。
下面是一个示例代码,演示如何使用Jsoup爬取分页数据:
```java
import java.io.IOException;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
public class PageCrawler {
public static void main(String[] args) throws IOException {
// 定义要爬取的网页地址和需要爬取的内容
String url = "https://example.com/page/1";
String cssSelector = ".list-item";
// 使用Jsoup连接网页,获取网页内容
Document doc = Jsoup.connect(url).get();
// 使用Jsoup解析网页内容,提取需要的数据
Elements items = doc.select(cssSelector);
for (Element item : items) {
// 处理每个数据项
String title = item.select(".title").text();
String description = item.select(".description").text();
System.out.println(title);
System.out.println(description);
System.out.println("------------");
}
// 如果网页中存在多页数据,使用循环遍历所有网页
for (int i = 2; i <= 10; i++) {
String nextUrl = "https://example.com/page/" + i;
doc = Jsoup.connect(nextUrl).get();
items = doc.select(cssSelector);
for (Element item : items) {
// 处理每个数据项
String title = item.select(".title").text();
String description = item.select(".description").text();
System.out.println(title);
System.out.println(description);
System.out.println("------------");
}
}
// 将提取的数据保存到本地或数据库中
// ...
}
}
```
在示例代码中,我们首先定义了要爬取的网页地址和需要爬取的内容。
然后,我们使用Jsoup连接网页,获取网页内容,并使用Jsoup解析网页内容,提取需要的数据。
如果网页中存在多页数据,我们使用循环遍历所有网页,重复步骤2和步骤3。
最后,我们可以将提取的数据保存到本地或数据库中。
阅读全文
相关推荐
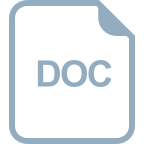
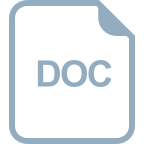
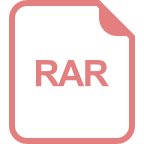
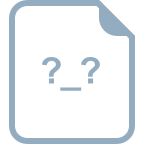
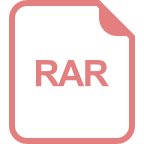
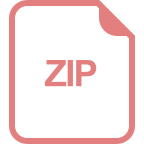
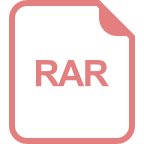
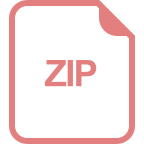
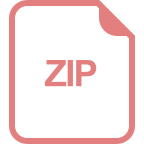
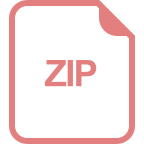
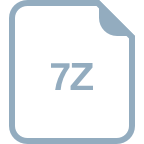
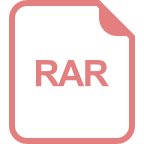
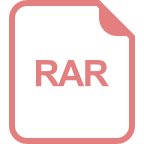
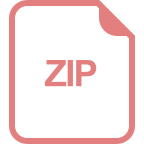
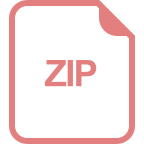
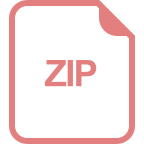
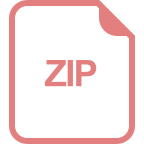
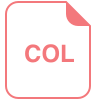