w=data.quantile(np.arange(0,1+1.0/k,1.0/k)) data=pd.cut(data,w)
时间: 2024-05-31 19:13:51 浏览: 17
这段代码的作用是将数据进行分箱(binning)处理,将连续的数值型数据离散化成指定数量的区间,常用于特征工程中对连续型变量进行离散化处理,以便更好地挖掘特征。具体来说,代码中首先使用 `quantile()` 函数将数据分成 `k` 份,每份的数据量相等,然后使用 `cut()` 函数将数据按照这些分位数进行分箱,得到的结果是一个 `pandas` 序列,每个元素表示原始数据所在的区间。这个操作可以用于处理连续型变量,将其转化为类别型变量,便于进一步处理。
相关问题
data = data[data <= data.quantile(0.2)]
这段代码的作用是将数据框中小于等于该数据框的0.2分位数的数据筛选出来。具体来说,它会将数据框中所有小于等于0.2分位数的数据保留下来,而将大于0.2分位数的数据删除掉。这个操作可以用于数据的预处理,例如去除异常值或者将数据离散化。需要注意的是,这个操作只适用于数值型数据,对于字符型数据或其他类型的数据可能会出现错误。
hbin = 10 for i in tqdm(data['WindNumber'].unique()): col = 'WindSpeed' cond = (data.WindNumber==i) & (data.label == 0) temp_df = data[cond] h_bins = pd.cut(temp_df.Power, np.arange(-1000, 3000, hbin)) temp_df['hbins'] = h_bins groups = [] for index,temp in temp_df.groupby("hbins"): if temp.shape[0]==0: continue iqr = temp[col].quantile(0.75) - temp[col].quantile(0.25) t1 = temp[col].quantile(0.25) - 1.5 * iqr t2 = temp[col].quantile(0.75) + 1.5 * iqr temp = temp[((temp[col]<t1) | (temp[col]>t2))] groups.append(temp) groups = pd.concat(groups).reset_index(drop = True) cond = (data.WindNumber==i) & (data.Time.isin(groups.Time)) data.loc[cond,'label'] = 3 cond = (data.WindNumber==1) vis_result_2D(data[cond],data[cond]['label'])详细解释
这段代码是对数据集中的每个风机进行处理,首先根据风机编号筛选数据,然后根据功率值将数据分为若干个区间,每个区间内的数据按照风速进行离群值检测,如果检测出离群值,则将对应的数据标记为异常(标签为3),最后将标记为异常的数据可视化展示。
具体解释如下:
1. `hbin = 10`:将功率值分为10个区间,每个区间的宽度为1000W。
2. `for i in tqdm(data['WindNumber'].unique()):`:循环遍历数据集中的所有风机。
3. `cond = (data.WindNumber==i) & (data.label == 0)`:根据风机编号和标签筛选数据,其中标签为0表示数据未被标记为异常。
4. `temp_df = data[cond]`:将筛选后的数据赋值给临时变量temp_df。
5. `h_bins = pd.cut(temp_df.Power, np.arange(-1000, 3000, hbin))`:根据功率值将数据分为若干个区间,并将区间标签赋值给 h_bins 变量。
6. `temp_df['hbins'] = h_bins`:将区间标签添加到数据集中。
7. `groups = []`:创建一个空列表,用于存储检测出的离群值数据。
8. `for index,temp in temp_df.groupby("hbins"):`:根据区间标签将数据分组,循环遍历每个区间。
9. `if temp.shape[0]==0:`:如果区间内没有数据,则跳过本次循环。
10. `iqr = temp[col].quantile(0.75) - temp[col].quantile(0.25)`:计算数据的四分位距。
11. `t1 = temp[col].quantile(0.25) - 1.5 * iqr`:计算下界阈值。
12. `t2 = temp[col].quantile(0.75) + 1.5 * iqr`:计算上界阈值。
13. `temp = temp[((temp[col]<t1) | (temp[col]>t2))]`:筛选出离群值数据,即风速小于下界阈值或大于上界阈值的数据。
14. `groups.append(temp)`:将检测出的离群值数据添加到 groups 列表中。
15. `groups = pd.concat(groups).reset_index(drop = True)`:将 groups 列表中的数据合并为一个数据集。
16. `cond = (data.WindNumber==i) & (data.Time.isin(groups.Time))`:根据风机编号和时间标记异常数据。
17. `data.loc[cond,'label'] = 3`:将标记为异常的数据标签设置为3。
18. `cond = (data.WindNumber==1)`:根据风机编号筛选数据。
19. `vis_result_2D(data[cond],data[cond]['label'])`:将标记后的数据可视化展示,其中 vis_result_2D 是一个自定义的函数。
相关推荐
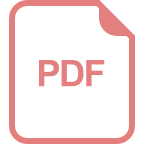
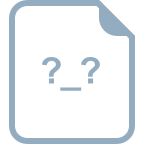
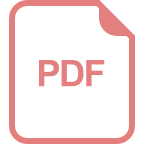












