使用arraylist存储一个班的学生信息,实现对学生类的对象进行插入、遍历、查找、排序(按学生姓名)等功能。
时间: 2023-05-31 18:20:16 浏览: 178
### 回答1:
可以使用ArrayList来存储一个班级的学生信息,并实现对学生类的插入、遍历、查找、排序(按学生姓名)等功能。每个学生信息可以通过一个包含学生姓名、学号、出生日期等属性的Student类来实现。学生类可以重写compareTo方法来实现按姓名排序。
### 回答2:
使用ArrayList来存储一个班的学生信息,可以方便地对学生类的对象进行插入、遍历、查找和排序(按学生姓名)等操作。下面我们分别介绍这些功能实现的方法和代码示例。
1.插入学生信息
通过ArrayList的add()方法可以向集合中添加学生对象,可以直接创建一个学生类,或者通过用户输入信息来创建学生对象。
代码示例:
```
// 创建一个ArrayList来存储学生信息
ArrayList<Student> students = new ArrayList<>();
// 创建一个学生对象,并添加到ArrayList中
Student student1 = new Student("张三", "男", 18, "计算机科学");
students.add(student1);
// 通过用户输入信息来创建学生对象,并添加到ArrayList中
Scanner scanner = new Scanner(System.in);
System.out.print("请输入学生姓名:");
String name = scanner.nextLine();
System.out.print("请输入学生性别:");
String gender = scanner.nextLine();
System.out.print("请输入学生年龄:");
int age = scanner.nextInt();
scanner.nextLine();
System.out.print("请输入学生专业:");
String major = scanner.nextLine();
Student student2 = new Student(name, gender, age, major);
students.add(student2);
```
2.遍历学生信息
通过ArrayList的for循环可以遍历集合中的所有学生对象,并输出每个学生的信息。
代码示例:
```
// 遍历ArrayList中的所有学生对象
for (Student student : students) {
System.out.println("姓名:" + student.getName() + ",性别:" + student.getGender() + ",年龄:" + student.getAge() + ",专业:" + student.getMajor());
}
```
3.查找学生信息
通过ArrayList的contains()方法可以判断集合中是否包含某个学生对象,通过indexOf()方法可以获取某个学生对象在集合中的索引位置。
代码示例:
```
// 判断ArrayList是否包含某个学生对象
Student student3 = new Student("王五", "男", 20, "软件工程");
if (students.contains(student3)) {
System.out.println("该学生已存在");
} else {
System.out.println("该学生不存在");
}
// 获取某个学生对象在ArrayList中的索引位置
int index = students.indexOf(student2);
System.out.println("该学生在ArrayList中的索引位置为:" + index);
```
4.排序学生信息
通过实现Comparable接口并重写compareTo()方法可以对学生对象进行排序(按学生姓名),然后通过Collections的sort()方法可以对集合中的所有学生对象进行排序。
代码示例:
```
// 实现Comparable接口并重写compareTo()方法,使得可以按照学生姓名进行排序
public class Student implements Comparable<Student> {
// ...
@Override
public int compareTo(Student o) {
return this.name.compareTo(o.getName());
}
}
// 调用Collections的sort()方法对ArrayList中的学生对象按照姓名进行排序
Collections.sort(students);
// 输出排序后的学生信息
System.out.println("按照姓名排序后的学生信息:");
for (Student student : students) {
System.out.println("姓名:" + student.getName() + ",性别:" + student.getGender() + ",年龄:" + student.getAge() + ",专业:" + student.getMajor());
}
```
以上就是使用ArrayList存储一个班的学生信息,实现对学生类的对象进行插入、遍历、查找、排序(按学生姓名)等功能的方法和代码示例。
### 回答3:
关于使用arraylist存储一个班的学生信息,我们可以首先定义一个学生类,该类包含学生姓名、学号、班级、年龄等属性。可以使用ArrayList<Student>来存储学生的信息,其中Student表示学生类的对象。
一、插入操作
添加学生信息时,可以通过 ArrayList 的add()方法实现添加操作,示例如下:
ArrayList<Student> list = new ArrayList<Student>();
Student student1 = new Student("张三", "001", "一班", "18");
list.add(student1);
其中student1为学生类的对象,上述代码表示将学生对象student1添加到list中。同样地,可以继续添加其他学生信息。
二、遍历操作
遍历操作是指对存储在ArrayList中的学生信息进行遍历,可以使用foreach循环或者for循环实现。示例如下:
//使用foreach循环遍历
for (Student student : list) {
System.out.println(student.toString());
}
//使用for循环遍历
for (int i = 0; i < list.size(); i++) {
System.out.println(list.get(i).toString());
}
其中toString()方法可以将学生类对象的信息以字符串的形式输出。
三、查找操作
查找操作是指在ArrayList中查找特定的学生信息,可以使用for循环或者foreach循环遍历ArrayList,逐个进行比较查找。示例如下:
//使用foreach循环查找
for (Student student : list) {
if (student.getStudentName().equals("张三")) {
System.out.println(student.toString());
break;
}
}
//使用for循环查找
for (int i = 0; i < list.size(); i++) {
if (list.get(i).getStudentName().equals("张三")) {
System.out.println(list.get(i).toString());
break;
}
}
在上述代码中,使用equals()方法比较学生姓名是否与查找条件相同,如果相同,则输出该学生的信息。
四、排序操作
排序操作是指对存储在ArrayList中的学生信息按照学生姓名进行排序,可以使用Collections类的sort()方法实现。示例如下:
Collections.sort(list, new Comparator<Student>() {
@Override
public int compare(Student o1, Student o2) {
return o1.getStudentName().compareTo(o2.getStudentName());
}
});
上述代码表示使用Comparator接口的compare()方法比较两个学生对象的姓名,如果o1的姓名比o2的姓名小,则返回负数,反之则返回正数。最终实现了按照学生姓名进行排序的功能。
综上,通过定义学生类和使用ArrayList存储学生信息,我们可以实现对学生类的对象进行插入、遍历、查找、排序(按学生姓名)等功能。
阅读全文
相关推荐
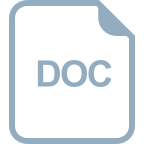
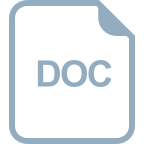
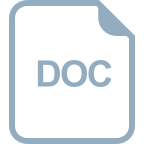

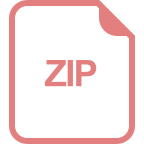
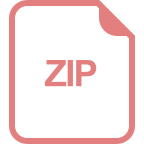
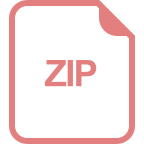
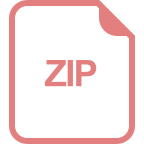
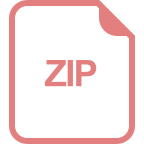
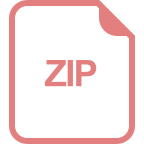
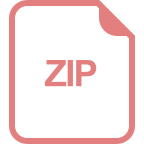
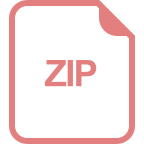
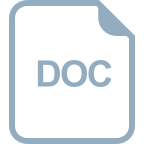



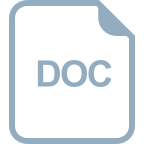
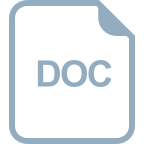
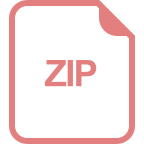