创建一个C++学生类课程类选课系统的代码
时间: 2023-06-08 16:05:50 浏览: 228
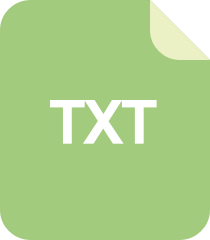
c++学生选课系统代码

好的,以下是一个简单的 C++ 学生课程选课系统的代码框架:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 课程类
class Course {
public:
Course(string name, int credit, int capacity) {
name_ = name;
credit_ = credit;
capacity_ = capacity;
student_list_.resize(capacity_);
}
// 获取课程名称
string name() const {
return name_;
}
// 获取学分
int credit() const {
return credit_;
}
// 获取当前选课人数
int current_capacity() const {
return current_capacity_;
}
// 获取总容量
int capacity() const {
return capacity_;
}
// 添加学生
bool add_student(string name, string id) {
if (current_capacity_ >= capacity_) {
return false;
}
student_list_[current_capacity_++] = name + ", " + id;
return true;
}
// 展示当前选课人员名单
void show_student_list() const {
cout << "Current student list of course " << name_ << ":" << endl;
for (int i = 0; i < current_capacity_; ++i) {
cout << student_list_[i] << endl;
}
}
private:
string name_; // 课程名称
int credit_; // 学分
int capacity_; // 可容纳人数
int current_capacity_ = 0; // 当前选课人数
vector<string> student_list_; // 学生名单
};
// 学生类
class Student {
public:
Student(string name, string id) {
name_ = name;
id_ = id;
}
// 获取学生姓名
string name() const {
return name_;
}
// 获取学生 ID
string id() const {
return id_;
}
// 注册课程
bool register_course(Course& course) {
if (courses_.size() >= kMaxCourseNum) {
return false;
}
courses_.push_back(&course);
return course.add_student(name_, id_);
}
// 展示当前注册的课程
void show_courses() const {
cout << "Current courses of student " << name_ << ":" << endl;
for (auto course : courses_) {
cout << course->name() << endl;
}
}
private:
string name_; // 姓名
string id_; // 学号
vector<Course*> courses_; // 注册课程列表
static const int kMaxCourseNum = 5; // 最大选课数目
};
// 主函数可以按需添加
int main() {
return 0;
}
```
该代码框架实现了一个简单的学生选课系统,包含了学生和课程两个核心类。课程类可以记录课程名称、学分、总容量、已选人数以及学生名单,并提供注册学生和显示学生名单等方法;学生类可以记录学生姓名、学号以及注册的课程列表,并提供注册课程和显示已注册课程等方法。
需要注意的点有:
1. 当前实现中,选课人数已满时,不能再添加新的学生选课。
2. 学生的选课数目是有上限的,当前实现中最多可注册 5 门课程。
3. 程序中未进行任何输入输出的操作,需要按需添加,以实现完整的选课系统。
阅读全文
相关推荐
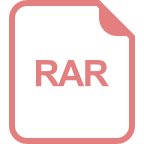
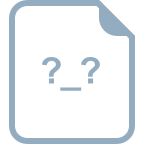
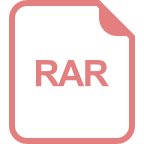
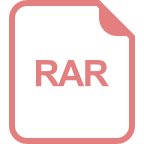
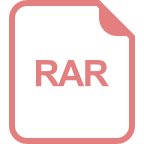
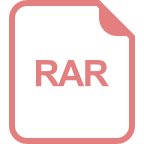
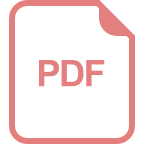
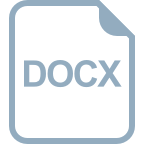
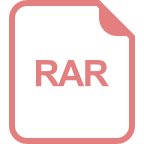
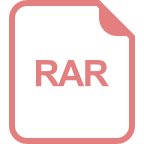
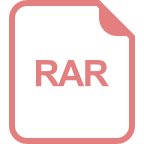
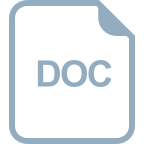
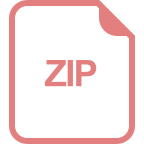
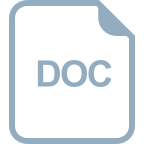
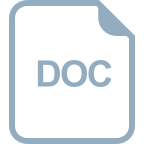
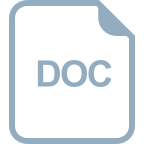