使用python构建一个决策树,使用sklearn里的iris数据集
时间: 2024-02-11 09:08:26 浏览: 65
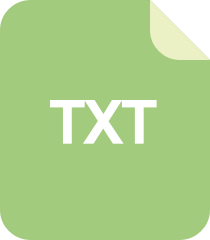
决策树莺尾花-Python scikit-learn库

好的,下面是使用Python和Scikit-learn构建决策树并使用Iris数据集进行训练和测试的代码:
首先,我们需要导入必要的库和数据集:
```python
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# load the iris dataset
iris = load_iris()
X = iris.data
y = iris.target
```
接着,将数据集分成训练集和测试集:
```python
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
```
我们将使用DecisionTreeClassifier类来构建决策树:
```python
# create a decision tree classifier
clf = DecisionTreeClassifier()
```
使用训练集来训练决策树:
```python
# train the classifier
clf.fit(X_train, y_train)
```
使用测试集来预测:
```python
# make predictions on the test set
y_pred = clf.predict(X_test)
```
最后,计算预测准确率:
```python
# calculate the accuracy of the classifier
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:", accuracy)
```
完整代码如下所示:
```python
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# load the iris dataset
iris = load_iris()
X = iris.data
y = iris.target
# split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# create a decision tree classifier
clf = DecisionTreeClassifier()
# train the classifier on the training set
clf.fit(X_train, y_train)
# make predictions on the test set
y_pred = clf.predict(X_test)
# calculate the accuracy of the classifier
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:", accuracy)
```
阅读全文
相关推荐
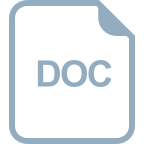
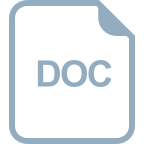





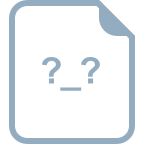







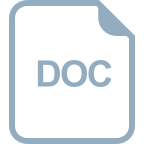
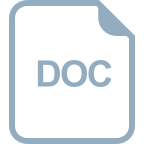