Pyqt中让progressbar根据Qprocess执行的进度不断更新
时间: 2024-05-07 21:19:54 浏览: 101
你可以通过以下代码实现让progressbar根据Qprocess执行的进度不断更新:
```python
from PyQt5.QtCore import QProcess, pyqtSignal, Qt
from PyQt5.QtGui import QTextCursor
from PyQt5.QtWidgets import QApplication, QDialog, QDialogButtonBox, QProgressBar, QVBoxLayout, QTextEdit
class ProcessDialog(QDialog):
def __init__(self, parent=None):
super(ProcessDialog, self).__init__(parent)
self.process = QProcess(self)
self.process.readyReadStandardOutput.connect(self.updateOutput)
self.process.finished.connect(self.processFinished)
self.progressBar = QProgressBar(self)
self.progressBar.setRange(0, 100)
self.logWindow = QTextEdit(self)
self.logWindow.setReadOnly(True)
self.buttonBox = QDialogButtonBox(Qt.Horizontal, self)
self.buttonBox.setStandardButtons(QDialogButtonBox.Cancel)
layout = QVBoxLayout(self)
layout.addWidget(self.progressBar)
layout.addWidget(self.logWindow)
layout.addWidget(self.buttonBox)
self.setLayout(layout)
self.buttonBox.rejected.connect(self.reject)
def startProcess(self, program, arguments):
self.process.start(program, arguments)
if not self.process.waitForStarted():
self.logWindow.append("Failed to start process")
self.reject()
self.logWindow.append("Process started: %s %s" % (program, ' '.join(arguments)))
def updateOutput(self):
cursor = self.logWindow.textCursor()
cursor.movePosition(QTextCursor.End)
cursor.insertText(str(self.process.readAllStandardOutput(), encoding='utf-8'))
self.logWindow.setTextCursor(cursor)
self.logWindow.ensureCursorVisible()
# 根据进程执行情况更新进度条
progress = self.process.readAll().data().decode('utf-8').strip()
if progress.isnumeric():
self.progressBar.setValue(int(progress))
def processFinished(self):
self.logWindow.append("Process finished with exit code %d" % self.process.exitCode())
self.buttonBox.button(QDialogButtonBox.Cancel).setText("Close")
if __name__ == '__main__':
import sys
app = QApplication(sys.argv)
dialog = ProcessDialog()
dialog.startProcess('ping', ['-c', '10', 'google.com'])
dialog.exec_()
sys.exit(app.exec_())
```
在这个例子中,我们创建了一个ProcessDialog类,该类包含了一个QProgressBar和QTextEdit。我们使用QProcess执行了一个ping命令,并在QTextEdit中实时显示输出。在updateOutput函数中,我们还根据进程执行情况更新了QProgressBar。
相关推荐
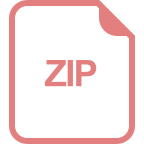
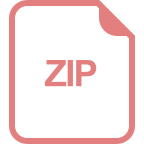
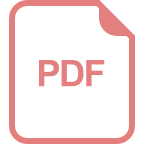














