ejor算法复现
时间: 2023-07-10 14:04:23 浏览: 78
EJOR算法是一种基于遗传算法的优化算法,其主要思想是将优化问题转化为一个带约束的组合优化问题,并通过遗传算法的方式来求解最优解。下面是一个简单的EJOR算法复现步骤:
1. 定义遗传算法的参数,包括种群大小、交叉率、变异率等。
2. 编码问题的解,将问题的解表示为一个二进制串。
3. 初始化种群,生成随机的二进制串作为初始种群。
4. 计算每个个体的适应度,根据问题的目标函数来定义适应度函数。
5. 采用选择、交叉和变异等操作对种群进行迭代优化,直到满足停止条件(例如达到预设的迭代次数)为止。
6. 根据迭代过程中产生的最优个体得到最优解。
下面是一个简单的Python代码实现:
```python
import random
# 定义问题的目标函数
def objective_function(x):
return x ** 2 - 10 * math.cos(2 * math.pi * x)
# 定义适应度函数
def fitness_function(x):
return 1 / (1 + objective_function(x))
# 定义编码函数
def encode(x, l, u, n):
return bin(round((x - l) * 2 ** n / (u - l)))[2:].rjust(n, '0')
# 定义解码函数
def decode(x, l, u, n):
return l + int(x, 2) * (u - l) / 2 ** n
# 初始化种群
def initialize_population(population_size, l, u, n):
population = []
for i in range(population_size):
x = random.uniform(l, u)
chromosome = encode(x, l, u, n)
population.append(chromosome)
return population
# 选择操作
def selection(population, fitness_values):
total_fitness = sum(fitness_values)
probabilities = [fitness / total_fitness for fitness in fitness_values]
selected_indices = random.choices(range(len(population)), weights=probabilities, k=2)
return population[selected_indices[0]], population[selected_indices[1]]
# 交叉操作
def crossover(parent1, parent2, crossover_rate):
if random.random() < crossover_rate:
crossover_point = random.randint(1, len(parent1) - 1)
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
else:
return parent1, parent2
# 变异操作
def mutation(chromosome, mutation_rate):
mutated_chromosome = ''
for bit in chromosome:
if random.random() < mutation_rate:
mutated_bit = '0' if bit == '1' else '1'
mutated_chromosome += mutated_bit
else:
mutated_chromosome += bit
return mutated_chromosome
# EJOR算法主函数
def ejor_algorithm(population_size, l, u, n, crossover_rate, mutation_rate, max_generations):
population = initialize_population(population_size, l, u, n)
best_solution = None
for generation in range(max_generations):
fitness_values = [fitness_function(decode(chromosome, l, u, n)) for chromosome in population]
best_index = fitness_values.index(max(fitness_values))
best_chromosome = population[best_index]
best_fitness = fitness_values[best_index]
if best_solution is None or best_fitness > fitness_function(decode(best_solution, l, u, n)):
best_solution = best_chromosome
new_population = []
while len(new_population) < population_size:
parent1, parent2 = selection(population, fitness_values)
child1, child2 = crossover(parent1, parent2, crossover_rate)
child1 = mutation(child1, mutation_rate)
child2 = mutation(child2, mutation_rate)
new_population.append(child1)
new_population.append(child2)
population = new_population
return decode(best_solution, l, u, n)
```
注意,这里的代码只是一个简单的实现示例,实际应用中需要根据具体问题进行适当的修改和优化。
相关推荐
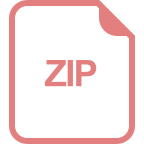
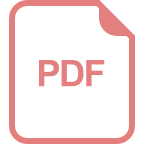
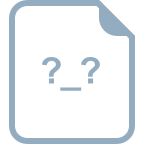
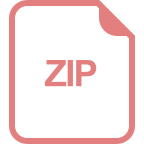
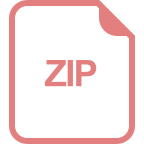
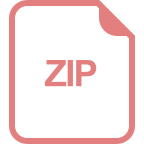
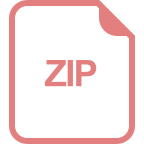
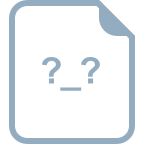
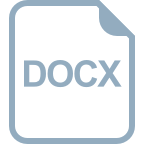
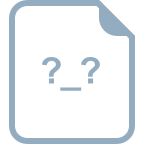
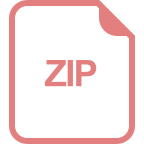
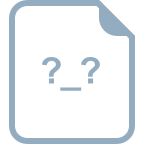
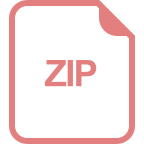
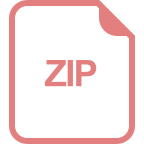
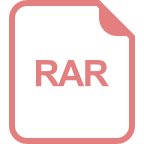