采用Java的BFS实现三个酒瓶的分酒问题
时间: 2024-04-08 12:31:20 浏览: 59
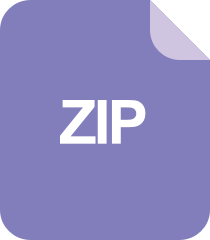
BFS-Labyrinth:带队列的广度优先搜索迷宫
好的,我可以帮你解答这个问题。以下是使用Java的BFS算法来实现三个酒瓶的分酒问题的示例代码:
```java
import java.util.*;
public class BottleProblem {
// 定义酒瓶的容量
private static final int[] CAPACITY = {12, 8, 5};
// 定义酒瓶状态类
private static class State {
private int[] bottles;
public State(int[] bottles) {
this.bottles = bottles;
}
public int[] getBottles() {
return bottles;
}
public boolean isGoalState() {
return bottles[0] == 6 && bottles[1] == 6 && bottles[2] == 0;
}
public List<State> getNextStates() {
List<State> nextStates = new ArrayList<>();
// 尝试所有可能的操作
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
if (i != j) {
int[] newBottles = Arrays.copyOf(bottles, 3);
int amount = Math.min(newBottles[i], CAPACITY[j] - newBottles[j]);
newBottles[i] -= amount;
newBottles[j] += amount;
nextStates.add(new State(newBottles));
}
}
}
return nextStates;
}
}
// 使用BFS算法求解
public static void solve() {
Queue<State> queue = new LinkedList<>();
Set<State> visited = new HashSet<>();
// 初始状态,三个酒瓶都为空
int[] initBottles = {0, 0, 0};
State initialState = new State(initBottles);
queue.add(initialState);
visited.add(initialState);
while (!queue.isEmpty()) {
State currentState = queue.poll();
if (currentState.isGoalState()) {
System.out.println("找到解决方案!");
System.out.println(Arrays.toString(currentState.getBottles()));
return;
}
List<State> nextStates = currentState.getNextStates();
for (State nextState : nextStates) {
if (!visited.contains(nextState)) {
queue.add(nextState);
visited.add(nextState);
}
}
}
System.out.println("未找到解决方案!");
}
public static void main(String[] args) {
solve();
}
}
```
这段代码使用BFS算法来搜索所有可能的状态,直到找到目标状态(即三个酒瓶中有6升、6升和0升的解决方案)或者搜索完所有可能的状态。在每个状态中,我们尝试所有可能的操作,即从一个酒瓶向另一个酒瓶倒酒,直到酒瓶中的酒量达到容量限制或者另一个酒瓶已满为止。
希望这个示例代码能够帮助到你!如果有任何问题,请随时提问。
阅读全文
相关推荐
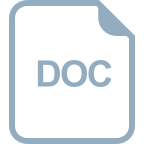
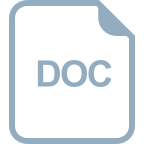
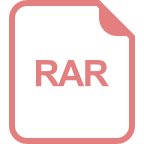
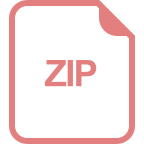
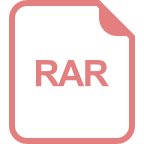
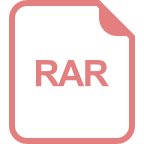
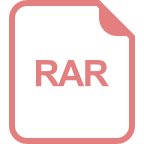
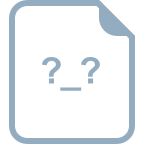
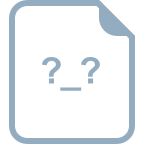
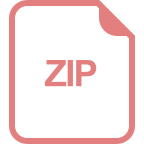
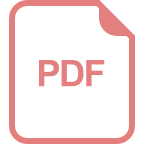
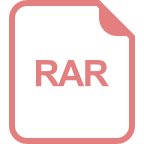
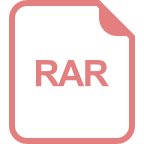
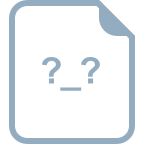