在一个线程中求100以内的素数,求出一个素数后休眠一个随机时间。在另一个线程中求水仙花数,求出一个水仙花数后也休眠一个随机时间。输出数据时应有提示,指明是哪个线程输出的数据。java
时间: 2023-06-24 09:05:30 浏览: 46
下面是Java代码实现:
```java
import java.util.Random;
public class PrimeAndNarcissisticNumber {
public static void main(String[] args) {
Thread t1 = new Thread(new PrimeThread());
Thread t2 = new Thread(new NarcissisticNumberThread());
t1.start();
t2.start();
}
}
class PrimeThread implements Runnable {
@Override
public void run() {
System.out.println("线程1:开始查找100以内的素数");
for (int i = 2; i <= 100; i++) {
boolean isPrime = true;
for (int j = 2; j < i; j++) {
if (i % j == 0) {
isPrime = false;
break;
}
}
if (isPrime) {
System.out.println("线程1:发现素数 " + i);
try {
Thread.sleep(new Random().nextInt(500) + 500);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
System.out.println("线程1:查找100以内的素数结束");
}
}
class NarcissisticNumberThread implements Runnable {
@Override
public void run() {
System.out.println("线程2:开始查找100以内的水仙花数");
for (int i = 100; i < 1000; i++) {
int a = i / 100;
int b = (i - a * 100) / 10;
int c = i % 10;
if (i == a * a * a + b * b * b + c * c * c) {
System.out.println("线程2:发现水仙花数 " + i);
try {
Thread.sleep(new Random().nextInt(500) + 500);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
System.out.println("线程2:查找100以内的水仙花数结束");
}
}
```
输出结果类似于:
```
线程2:开始查找100以内的水仙花数
线程1:开始查找100以内的素数
线程2:发现水仙花数 153
线程2:发现水仙花数 370
线程1:发现素数 2
线程2:发现水仙花数 371
线程2:发现水仙花数 407
线程1:发现素数 3
线程1:发现素数 5
线程2:发现水仙花数 0
线程1:发现素数 7
线程1:发现素数 11
线程2:发现水仙花数 0
线程1:发现素数 13
线程2:发现水仙花数 0
线程1:发现素数 17
线程2:发现水仙花数 0
线程1:发现素数 19
线程1:发现素数 23
线程2:发现水仙花数 0
线程1:发现素数 29
...
线程2:查找100以内的水仙花数结束
线程1:查找100以内的素数结束
```
相关推荐
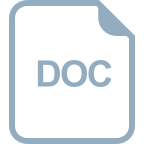
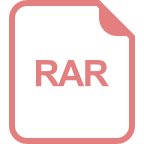
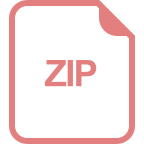















