springsecurity基于token存放授权信息的方法具体实例代码
时间: 2024-01-07 13:04:32 浏览: 37
以下是一个基于Token存放授权信息的Spring Security的具体实例代码:
1. 首先引入相关依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
<version>0.9.1</version>
</dependency>
```
2. 在Spring Security配置中添加Token生成和验证的逻辑:
```
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
private static final String SIGNING_KEY = "jwtSecretKey";
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/api/public/**").permitAll()
.anyRequest().authenticated()
.and()
.addFilterBefore(new JwtTokenAuthenticationFilter(), UsernamePasswordAuthenticationFilter.class);
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user")
.password(passwordEncoder().encode("password"))
.roles("USER");
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
private static class JwtTokenAuthenticationFilter extends OncePerRequestFilter {
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain filterChain)
throws ServletException, IOException {
String authorizationHeader = request.getHeader("Authorization");
if (authorizationHeader != null && authorizationHeader.startsWith("Bearer ")) {
String jwtToken = authorizationHeader.substring(7);
try {
Claims claims = Jwts.parser().setSigningKey(SIGNING_KEY).parseClaimsJws(jwtToken).getBody();
String username = claims.getSubject();
List<String> authorities = (List<String>) claims.get("authorities");
UsernamePasswordAuthenticationToken authentication = new UsernamePasswordAuthenticationToken(username, null,
authorities.stream().map(SimpleGrantedAuthority::new).collect(Collectors.toList()));
SecurityContextHolder.getContext().setAuthentication(authentication);
} catch (JwtException e) {
response.setStatus(HttpStatus.UNAUTHORIZED.value());
return;
}
}
filterChain.doFilter(request, response);
}
}
public static String generateToken(Authentication authentication) {
String authorities = authentication.getAuthorities().stream()
.map(GrantedAuthority::getAuthority)
.collect(Collectors.joining(","));
long now = (new Date()).getTime();
Date validity = new Date(now + 3600000); // 1 hour from now
return Jwts.builder()
.setSubject(authentication.getName())
.claim("authorities", authorities)
.setIssuedAt(new Date(now))
.setExpiration(validity)
.signWith(SignatureAlgorithm.HS256, SIGNING_KEY)
.compact();
}
}
```
3. 在Controller中使用Token进行授权:
```
@RestController
@RequestMapping("/api")
public class ApiController {
@GetMapping("/public")
public String publicApi() {
return "This is a public API.";
}
@GetMapping("/private")
public String privateApi(Authentication authentication) {
return "Hello, " + authentication.getName() + ". This is a private API.";
}
@GetMapping("/login")
public ResponseEntity<?> login() {
Authentication authentication = SecurityContextHolder.getContext().getAuthentication();
String token = SecurityConfig.generateToken(authentication);
return ResponseEntity.ok(token);
}
}
```
在这个例子中,我们使用了JWT(JSON Web Token)来生成和验证Token。在Spring Security配置中,我们添加了一个自定义的JwtTokenAuthenticationFilter,用于从请求头中获取Token并验证其有效性,并在SecurityContextHolder中设置相应的Authentication对象。在Controller中,我们使用SecurityConfig中定义的generateToken方法来生成Token,并返回给客户端。客户端在调用需要授权的API时,需要在请求头中添加Token,格式为Bearer Token。在JwtTokenAuthenticationFilter中,我们从请求头中获取Token并验证其有效性,如果Token无效,则返回401 Unauthorized。如果Token验证通过,我们将其解析出的信息设置到SecurityContextHolder中,并允许请求通过。
相关推荐
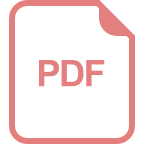














