ll1文法分析器 c语言
时间: 2023-06-21 08:16:10 浏览: 249
LL(1)文法是一种上下文无关文法,可以用于描述程序语言的语法结构。在编译原理中,LL(1)文法分析器是一种自顶向下的语法分析器,它可以根据输入的符号串,判断是否符合给定文法的规则,并构建出语法树。
在C语言中,可以使用LL(1)文法分析器来解析C源代码,以便进行语法检查、代码生成等操作。下面是一个简单的LL(1)文法分析器的实现,可以用来解析C语言的基本语法结构:
1. 定义文法规则
首先,需要定义C语言的文法规则。例如,可以定义如下的文法规则:
```
<program> ::= <declaration-list>
<declaration-list> ::= <declaration> | <declaration> <declaration-list>
<declaration> ::= <type-specifier> <identifier> ";" | <type-specifier> <identifier> "(" <parameter-list> ")" <compound-statement>
<type-specifier> ::= "void" | "int" | "float" | "double" | "char" | "short" | "long"
<identifier> ::= <letter> | <identifier> <letter> | <identifier> <digit>
<letter> ::= "a" | "b" | "c" | ... | "z" | "A" | "B" | "C" | ... | "Z"
<digit> ::= "0" | "1" | "2" | ... | "9"
<parameter-list> ::= <parameter-declaration> | <parameter-declaration> "," <parameter-list>
<parameter-declaration> ::= <type-specifier> <identifier>
<compound-statement> ::= "{" <declaration-list> <statement-list> "}"
<statement-list> ::= <statement> | <statement> <statement-list>
<statement> ::= <expression-statement> | <compound-statement> | <selection-statement> | <iteration-statement> | <jump-statement>
<expression-statement> ::= <expression> ";"
<expression> ::= <assignment-expression> | <expression> "," <assignment-expression>
<assignment-expression> ::= <conditional-expression> | <unary-expression> <assignment-operator> <assignment-expression>
<conditional-expression> ::= <logical-or-expression> | <logical-or-expression> "?" <expression> ":" <conditional-expression>
<logical-or-expression> ::= <logical-and-expression> | <logical-or-expression> "||" <logical-and-expression>
<logical-and-expression> ::= <equality-expression> | <logical-and-expression> "&&" <equality-expression>
<equality-expression> ::= <relational-expression> | <equality-expression> "==" <relational-expression> | <equality-expression> "!=" <relational-expression>
<relational-expression> ::= <additive-expression> | <relational-expression> "<" <additive-expression> | <relational-expression> "<=" <additive-expression> | <relational-expression> ">" <additive-expression> | <relational-expression> ">=" <additive-expression>
<additive-expression> ::= <multiplicative-expression> | <additive-expression> "+" <multiplicative-expression> | <additive-expression> "-" <multiplicative-expression>
<multiplicative-expression> ::= <unary-expression> | <multiplicative-expression> "*" <unary-expression> | <multiplicative-expression> "/" <unary-expression>
<unary-expression> ::= <primary-expression> | <unary-operator> <unary-expression>
<primary-expression> ::= <identifier> | <constant> | "(" <expression> ")"
<constant> ::= <integer-constant> | <floating-constant> | <character-constant>
<integer-constant> ::= <digit> | <integer-constant> <digit>
<floating-constant> ::= <digit> "." <digit> | <digit> "." <digit> <exponent-part> | "." <digit> <exponent-part> | <digit> <exponent-part>
<exponent-part> ::= "e" <sign> <digit> | "E" <sign> <digit> | <empty>
<sign> ::= "+" | "-"
<character-constant> ::= "'" <character> "'"
<character> ::= <letter> | <digit> | <special-character>
<special-character> ::= "~" | "!" | "@" | "#" | "$" | "%" | "^" | "&" | "*" | "(" | ")" | "-" | "+" | "=" | "{" | "}" | "[" | "]" | ":" | ";" | "<" | ">" | "," | "." | "?" | "/"
<unary-operator> ::= "+" | "-" | "!" | "~"
<assignment-operator> ::= "=" | "+=" | "-=" | "*=" | "/="
<selection-statement> ::= "if" "(" <expression> ")" <statement> | "if" "(" <expression> ")" <statement> "else" <statement> | "switch" "(" <expression> ")" <compound-statement>
<iteration-statement> ::= "while" "(" <expression> ")" <statement> | "do" <statement> "while" "(" <expression> ")" ";" | "for" "(" <expression> ";" <expression> ";" <expression> ")" <statement> | "for" "(" <declaration> <expression> ";" <expression> ")" <statement>
<jump-statement> ::= "goto" <identifier> ";" | "continue" ";" | "break" ";" | "return" <expression> ";" | "return" ";"
```
2. 实现LL(1)文法分析器
接下来,可以根据上述文法规则,实现LL(1)文法分析器。具体实现步骤如下:
(1)将输入的符号串转换为Token序列,即将源代码中的每个单词转换为对应的Token类型。
(2)定义LL(1)分析表,该表包含所有的非终结符和终结符,以及它们之间的推导规则。根据文法规则,可以逐步构建出LL(1)分析表。
(3)根据LL(1)分析表和Token序列,进行语法分析。具体步骤如下:
- 从Token序列中读入下一个Token,记为lookahead。
- 根据当前的非终结符和lookahead,查找LL(1)分析表中对应的推导规则。
- 如果推导规则中存在非终结符,则将该非终结符入栈,继续进行分析。
- 如果推导规则中存在终结符,则将该终结符与lookahead进行匹配,如果匹配成功,则弹出栈顶的非终结符,继续进行分析;否则,分析失败。
- 如果Token序列已经分析完毕,并且栈中只剩下一个非终结符(即开始符号),则分析成功;否则,分析失败。
下面是一个简单的LL(1)文法分析器的伪代码实现:
```
function LL1Parser(input):
initialize stack with start symbol
initialize lookahead with first token
while stack is not empty:
if top of stack is non-terminal:
if LL(1) table contains (top of stack, lookahead):
replace top of stack with corresponding production rule
continue parsing
else:
report syntax error
return
else if top of stack is terminal:
if top of stack matches lookahead:
pop top of stack
read next token from input and set it as lookahead
continue parsing
else:
report syntax error
return
if input is fully parsed and stack is empty:
report success
else:
report syntax error
```
以上就是一个简单的LL(1)文法分析器的实现方法,可以用来解析C语言的基本语法结构。
阅读全文
相关推荐

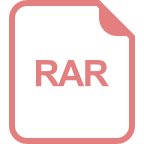



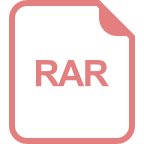
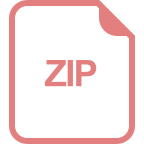
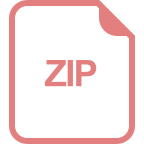
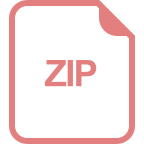
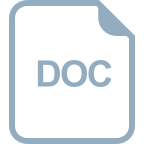
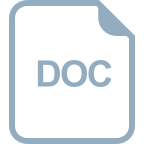