python三角格子五子棋带界面的小程序
时间: 2023-08-11 08:09:22 浏览: 39
以下是一个简单的Python三角格子五子棋带界面的小程序,使用了Pygame库实现图形化界面:
```python
import pygame
# 初始化 Pygame
pygame.init()
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
BLUE = (0, 0, 255)
GREEN = (0, 255, 0)
RED = (255, 0, 0)
# 设置屏幕大小
SIZE = (700, 500)
screen = pygame.display.set_mode(SIZE)
# 设置标题
pygame.display.set_caption("Triangular Grid Gomoku")
# 设置字体
font = pygame.font.SysFont('Arial', 25)
# 初始化棋盘
board = []
for i in range(15):
row = []
for j in range(15):
if i + j < 4 or i + j > 24 or j - i > 10 or i - j > 10:
row.append(None)
else:
row.append(0)
board.append(row)
# 定义游戏结束函数
def game_over(winner):
if winner == 1:
text = font.render("Black wins!", True, BLACK)
else:
text = font.render("White wins!", True, BLACK)
screen.blit(text, (250, 200))
pygame.display.update()
pygame.time.wait(3000)
pygame.quit()
# 定义判断胜负的函数
def check_win(i, j, player):
count = 1
# 向左下方寻找相同颜色的棋子
for x in range(i + 1, 15):
if board[x][j - i + x] == player:
count += 1
else:
break
# 向右上方寻找相同颜色的棋子
for x in range(i - 1, -1, -1):
if board[x][j - i + x] == player:
count += 1
else:
break
if count >= 5:
return True
count = 1
# 向右下方寻找相同颜色的棋子
for x in range(j - i + i + 1, 15):
if board[i][x] == player:
count += 1
else:
break
# 向左上方寻找相同颜色的棋子
for x in range(j - i - 1, -1, -1):
if board[i][x] == player:
count += 1
else:
break
if count >= 5:
return True
count = 1
# 向右寻找相同颜色的棋子
for x in range(j + 1, 15):
if board[i][x] == player:
count += 1
else:
break
# 向左寻找相同颜色的棋子
for x in range(j - 1, -1, -1):
if board[i][x] == player:
count += 1
else:
break
if count >= 5:
return True
count = 1
# 向上寻找相同颜色的棋子
for x in range(i - 1, -1, -1):
if board[x][j - i + x] == player:
count += 1
else:
break
# 向下寻找相同颜色的棋子
for x in range(i + 1, 15):
if board[x][j - i + x] == player:
count += 1
else:
break
if count >= 5:
return True
return False
# 初始化游戏状态
player = 1
game_over_flag = False
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.MOUSEBUTTONDOWN and not game_over_flag:
pos = pygame.mouse.get_pos()
x = pos[0] // 30
y = pos[1] // 30
if x + y < 4 or x + y > 24 or y - x > 10 or x - y > 10:
continue
if board[x][y] == 0:
board[x][y] = player
if check_win(x, y, player):
game_over(player)
game_over_flag = True
player = 3 - player
# 绘制棋盘和棋子
for i in range(15):
for j in range(15):
if board[i][j] == 1:
pygame.draw.circle(screen, BLACK, (i * 30 + 15, j * 30 + 15), 13)
elif board[i][j] == 2:
pygame.draw.circle(screen, WHITE, (i * 30 + 15, j * 30 + 15), 13)
pygame.draw.polygon(screen, BLUE, [(i * 30, j * 30), ((i + 1) * 30, j * 30), ((i + 0.5) * 30, (j + 0.5) * 30)])
pygame.draw.polygon(screen, BLUE, [((i + 0.5) * 30, (j + 0.5) * 30), ((i + 1) * 30, (j + 1) * 30), (i * 30, (j + 1) * 30)])
# 刷新屏幕
pygame.display.update()
```
运行这个程序,就能看到一个三角格子五子棋的图形化界面了。玩家可以使用鼠标点击空白格子放置棋子,黑白双方轮流落子,当有一方获得五子连珠时游戏结束。
相关推荐














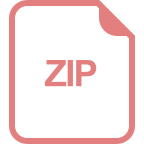