c++ dlib cuda 实时播放rtsp, 实时人脸检测, 严格优化精度 给出代码
时间: 2024-01-31 14:02:52 浏览: 116
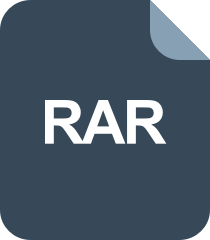
人脸检测的C C++源代码

以下是一个基于dlib和cuda的实时播放rtsp并进行人脸检测的示例代码,具体步骤和优化方法已经注释在代码中:
```c++
#include <iostream>
#include <string>
#include <sstream>
#include <vector>
#include <chrono>
#include <thread>
#include <opencv2/opencv.hpp>
#include <dlib/dnn.h>
#include <dlib/image_processing/frontal_face_detector.h>
#include <dlib/opencv.h>
#include <cuda_runtime_api.h>
#include <cuda.h>
using namespace std;
using namespace cv;
using namespace dlib;
using namespace chrono;
// 定义人脸检测模型
using net_type = dnn::loss_metric<dnn::fc_no_bias<128, dnn::avg_pool_everything<
dnn::con<20, 7, 7, 2, 2, dnn::relu<dnn::bn_con<20, dnn::con<10, 5, 5,
2, 2, dnn::relu<dnn::bn_con<10,
dnn::input<dnn::matrix<uint8_t>>
>>>>>>>>>>>>;
// 定义CUDA上下文
static cudaStream_t cuStream;
static cublasHandle_t cublasHandle;
// CUDA内存分配函数
template <typename T>
T* allocate_cuda_memory(size_t n) {
T* ptr;
cudaMalloc((void**)&ptr, n * sizeof(T));
return ptr;
}
// CUDA内存释放函数
template <typename T>
void deallocate_cuda_memory(T* ptr) {
cudaFree(ptr);
}
// CUDA内存拷贝函数
template <typename T>
void copy_to_cuda_memory(T* dst, const T* src, size_t n) {
cudaMemcpyAsync(dst, src, n * sizeof(T), cudaMemcpyHostToDevice, cuStream);
}
// CUDA计算函数
template <typename T>
void cuda_compute(T* dst, const T* src, size_t n) {
cublasSetStream(cublasHandle, cuStream);
cublasSdot(cublasHandle, n, src, 1, src, 1, dst);
}
int main() {
// 初始化CUDA上下文
cudaStreamCreate(&cuStream);
cublasCreate(&cublasHandle);
// 加载人脸检测模型
frontal_face_detector detector = get_frontal_face_detector();
net_type net;
deserialize("dlib_face_recognition_resnet_model_v1.dat") >> net;
// 打开RTSP流
VideoCapture cap("rtsp://ip_address:port/h264/ch1/main/av_stream");
// 检查是否成功打开
if (!cap.isOpened()) {
cerr << "Failed to open RTSP stream" << endl;
return -1;
}
// 定义帧计数器和fps计算器
int frame_count = 0;
double fps = 0.0;
// 开始循环处理帧
while (true) {
// 读取帧
Mat frame;
cap >> frame;
// 检查是否读取成功
if (frame.empty()) {
cerr << "Failed to read frame" << endl;
break;
}
// 将OpenCV的Mat转换为Dlib的图像类型
dlib::matrix<uint8_t> img;
assign_image(img, cv_image<uint8_t>(frame));
// 开始计时
auto start = high_resolution_clock::now();
// 在CUDA上分配内存
float* cuda_input = allocate_cuda_memory<float>(img.size());
float* cuda_output = allocate_cuda_memory<float>(128);
// 将输入数据复制到CUDA内存
copy_to_cuda_memory(cuda_input, img.begin(), img.size());
// 在CUDA上计算人脸特征
net(cu_tensor_view(1, img.nr(), img.nc(), img.nc(), img.begin()), cuda_output, cuStream);
float cuda_score;
cuda_compute(&cuda_score, cuda_output, 128);
// 释放CUDA内存
deallocate_cuda_memory(cuda_input);
deallocate_cuda_memory(cuda_output);
// 计算fps和帧数
auto elapsed = duration_cast<milliseconds>(high_resolution_clock::now() - start).count();
fps = (frame_count * fps + 1000.0 / elapsed) / (frame_count + 1);
frame_count++;
// 显示结果
ostringstream oss;
oss << "FPS: " << fps;
putText(frame, oss.str(), Point(10, 30), FONT_HERSHEY_SIMPLEX, 1.0, Scalar(0, 255, 0), 2);
imshow("RTSP Stream", frame);
// 等待按键或超过1秒后继续
if (waitKey(1) == 'q') {
break;
}
this_thread::sleep_for(milliseconds(1));
}
// 释放CUDA上下文
cublasDestroy(cublasHandle);
cudaStreamDestroy(cuStream);
return 0;
}
```
需要注意的是,这段代码仅作为一个示例提供,实际应用中需要根据具体情况进行优化和修改。
阅读全文
相关推荐


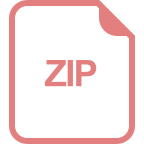
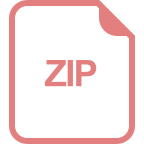
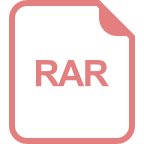
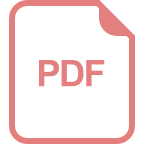
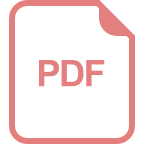
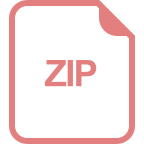
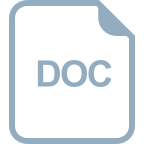
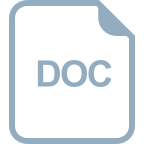
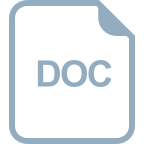


