如何使用python对neo4j进行遍历?
时间: 2024-05-09 11:14:21 浏览: 265
使用Python对Neo4j进行遍历可以使用Neo4j提供的Python驱动程序。以下是一个简单的遍历示例:
首先,需要安装neo4j-driver模块:
```
pip install neo4j-driver
```
然后,可以使用以下代码遍历一个简单的图:
```python
from neo4j import GraphDatabase
# 连接数据库
driver = GraphDatabase.driver("bolt://localhost:7687", auth=("neo4j", "password"))
# 定义遍历函数
def traverse(tx):
result = tx.run("MATCH (n) RETURN n")
for record in result:
print(record["n"])
# 执行遍历
with driver.session() as session:
session.read_transaction(traverse)
```
这个遍历函数会返回数据库中所有节点的名称和属性。你可以根据需要修改查询语句来获取你所需的节点和关系。
相关问题
如何使用python对neo4j进行广度优先遍历?
要在Python中实现Neo4j图形数据库的广度优先遍历,您需要使用Neo4j Python驱动程序和适当的算法。以下是一个简单的示例,演示如何使用Neo4j Python驱动程序实现广度优先遍历:
```python
from neo4j import GraphDatabase
# Neo4j数据库驱动程序
uri = "bolt://localhost:7687"
user = "neo4j"
password = "password"
driver = GraphDatabase.driver(uri, auth=(user, password))
# 广度优先遍历函数
def bfs(tx, start_node):
queue = [(start_node, 0)]
visited = set([start_node])
while queue:
node, level = queue.pop(0)
print(node, level)
for rel in node.relationships:
next_node = rel.end_node
if next_node not in visited:
visited.add(next_node)
queue.append((next_node, level + 1))
# 在Neo4j中执行广度优先遍历
with driver.session() as session:
result = session.read_transaction(bfs, start_node="start_node_id")
```
在此示例中,我们首先建立与Neo4j数据库的连接,然后定义了一个名为`bfs`的函数,该函数使用广度优先遍历算法遍历从`start_node`节点开始的Neo4j图形数据库。最后,我们使用Neo4j Python驱动程序和`bfs`函数在Neo4j数据库中执行广度优先遍历。
注意,这只是一个简单的示例,您可以根据自己的需求进行修改。还要注意,Neo4j Python驱动程序支持多线程,您可以在多个线程中执行广度优先遍历以提高性能。
如何在Python中操作Neo4j数据库?
在Python中操作Neo4j数据库,通常我们会使用官方提供的`neo4j`驱动库。以下是基本步骤:
1. **安装**:首先需要通过pip安装`neo4j`驱动程序,命令如下:
```
pip install neo4j
```
2. **连接到数据库**:创建`GraphDatabaseDriver`实例,并连接到服务器,例如:
```python
from neo4j import GraphDatabase
uri = "bolt://localhost:7687" # 根据实际情况替换地址和端口
driver = GraphDatabase.driver(uri, auth=("neo4j", "password")) # 输入正确的用户名和密码
session = driver.session()
```
3. **执行查询**:编写Cypher查询并执行:
```python
result = session.run("MATCH (n) RETURN n")
for record in result:
node = record["n"]
print(node)
```
4. **处理结果**:遍历查询结果,处理每个返回的数据点。
5. **关闭连接**:完成后记得关闭session和driver:
```python
session.close()
driver.close()
```
阅读全文
相关推荐
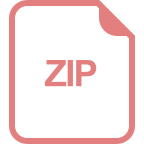
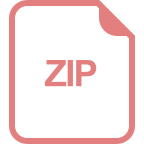
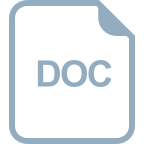
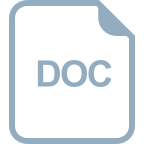
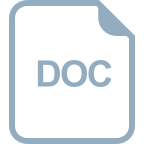









