要实现创建一个可编辑的表格,并且能够保存数据至服务器 并在下次打开页面时显示之前修改的数据的完整代码
时间: 2024-03-24 12:36:56 浏览: 133
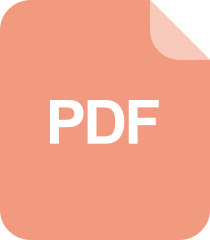
用Jquery实现可编辑表格并用AJAX提交到服务器修改数据
好的,这里提供一个使用 HTML、CSS、JavaScript 和 PHP 实现创建可编辑表格、保存数据至服务器、读取和显示数据的完整代码,代码注释比较详细,你可以参考以下代码进行实现:
HTML 代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>可编辑表格</title>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
text-align: left;
}
tr:nth-child(even) {
background-color: #f2f2f2;
}
.editable {
background-color: #eee;
cursor: pointer;
}
.editable:hover {
background-color: #ddd;
}
</style>
</head>
<body>
<h1>可编辑表格</h1>
<table id="myTable">
<thead>
<tr>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<!-- 这里通过 PHP 代码动态输出表格的数据 -->
<?php
require_once('config.php');
$conn = new mysqli(DB_HOST, DB_USER, DB_PASS, DB_NAME);
if ($conn->connect_error) {
die("连接失败: " . $conn->connect_error);
}
$sql = "SELECT * FROM mytable";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
// 输出数据
while($row = $result->fetch_assoc()) {
echo '<tr>';
echo '<td class="editable" contenteditable="true">' . $row["name"] . '</td>';
echo '<td class="editable" contenteditable="true">' . $row["age"] . '</td>';
echo '<td class="editable" contenteditable="true">' . $row["gender"] . '</td>';
echo '<td><button onclick="saveData(this)">保存</button></td>';
echo '</tr>';
}
} else {
echo '<tr><td colspan="4">暂无数据</td></tr>';
}
$conn->close();
?>
</tbody>
</table>
<script>
// 保存数据到服务器
function saveData(button) {
// 获取当前行的数据
const row = button.parentNode.parentNode;
const name = row.cells[0].textContent;
const age = row.cells[1].textContent;
const gender = row.cells[2].textContent;
const data = {
'name': name,
'age': age,
'gender': gender
};
// 使用 AJAX 技术将数据发送到服务器进行保存
const xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (xhr.readyState == 4 && xhr.status == 200) {
alert(xhr.responseText);
}
};
xhr.open('POST', 'save.php', true);
xhr.setRequestHeader('Content-type', 'application/json');
xhr.send(JSON.stringify(data));
}
</script>
</body>
</html>
```
CSS 代码:
```css
table {
border-collapse: collapse;
width: 100%;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
text-align: left;
}
tr:nth-child(even) {
background-color: #f2f2f2;
}
.editable {
background-color: #eee;
cursor: pointer;
}
.editable:hover {
background-color: #ddd;
}
```
JavaScript 代码:
```javascript
// 添加可编辑功能
const cells = document.querySelectorAll('.editable');
cells.forEach(function(cell) {
cell.addEventListener('click', function() {
this.classList.add('edit');
});
cell.addEventListener('blur', function() {
this.classList.remove('edit');
});
});
// 使用 AJAX 技术从服务器加载数据
const xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (xhr.readyState == 4 && xhr.status == 200) {
// 将服务器返回的数据填充到表格中
const data = JSON.parse(xhr.responseText);
const tbody = document.querySelector('tbody');
tbody.innerHTML = '';
data.forEach(function(item) {
const tr = document.createElement('tr');
tr.innerHTML = '<td class="editable" contenteditable="true">' + item.name + '</td>' +
'<td class="editable" contenteditable="true">' + item.age + '</td>' +
'<td class="editable" contenteditable="true">' + item.gender + '</td>' +
'<td><button onclick="saveData(this)">保存</button></td>';
tbody.appendChild(tr);
});
}
};
xhr.open('GET', 'load.php', true);
xhr.send();
```
PHP 代码(保存数据):
```php
<?php
require_once('config.php');
$data = json_decode(file_get_contents('php://input'), true);
$name = $data['name'];
$age = $data['age'];
$gender = $data['gender'];
$conn = new mysqli(DB_HOST, DB_USER, DB_PASS, DB_NAME);
if ($conn->connect_error) {
die("连接失败: " . $conn->connect_error);
}
$sql = "INSERT INTO mytable (name, age, gender) VALUES ('$name', '$age', '$gender')";
if ($conn->query($sql) === TRUE) {
echo "保存成功";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
$conn->close();
?>
```
PHP 代码(读取数据):
```php
<?php
require_once('config.php');
$conn = new mysqli(DB_HOST, DB_USER, DB_PASS, DB_NAME);
if ($conn->connect_error) {
die("连接失败: " . $conn->connect_error);
}
$sql = "SELECT * FROM mytable";
$result = $conn->query($sql);
$data = array();
if ($result->num_rows > 0) {
// 将查询结果转换为数组
while($row = $result->fetch_assoc()) {
array_push($data, $row);
}
}
echo json_encode($data); // 将数组转换为 JSON 格式并输出
$conn->close();
?>
```
这段代码中,使用了 MySQL 数据库进行数据的储存,需要在 `config.php` 文件中配置数据库的相关信息,例如:
```php
define('DB_HOST', 'localhost');
define('DB_USER', 'root');
define('DB_PASS', '123456');
define('DB_NAME', 'mydatabase');
```
这样,就完成了一个简单的可编辑表格,并且能够保存数据至服务器、读取和显示数据的实现。
阅读全文
相关推荐
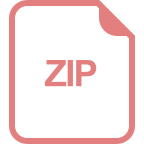
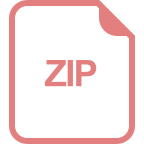
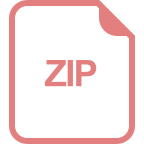
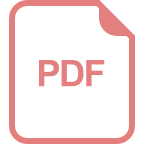
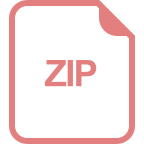
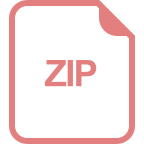
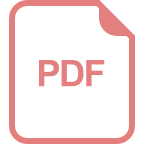
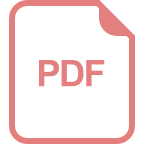
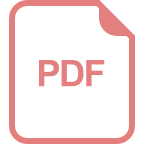
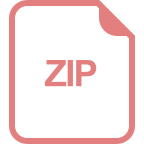
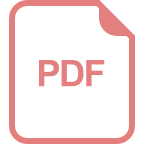
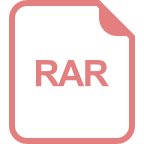
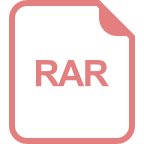
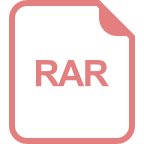
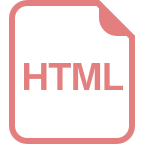
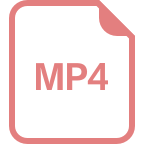