使用Java设计一个药店的药品销售统计系统,实现医药公司定期对销售各药品的记录进行统计,可按药品的编号、单价、销售量或销售额做出排名。 在本设计中,首先从txt文件中读出各药品的信息记录,存储在顺序表中。各药品的信息包括:药品编号、药名、药品单价、销出数量、销售额。药品编号共4位,采用字母和数字混合编号,如:A125,前一位为大写字母,后三位为数字,按药品编号进行排序时,可采用多种排序法。对各药品的单价、销售量或销售额进行排序时,可采用多种排序方法,如直接插入排序、冒泡排序、快速排序,直接选择排序等方法。在本系统中,对单价的排序采用冒泡排序法,对销售量的排序采用快速排序法,对销售额的排序采用堆排序法。
时间: 2024-03-03 16:52:36 浏览: 16
好的,这是一个非常详细的需求,需要设计一个药品销售统计系统,实现医药公司定期对销售各药品的记录进行统计,可按药品的编号、单价、销售量或销售额做出排名,其中药品编号共4位,采用字母和数字混合编号,如:A125,前一位为大写字母,后三位为数字。首先,我们需要从txt文件中读取各药品的信息记录,存储在顺序表中。各药品的信息包括药品编号、药名、药品单价、销出数量、销售额。
对于排序方法,我们采用以下的排序方法:
- 对单价的排序采用冒泡排序法
- 对销售量的排序采用快速排序法
- 对销售额的排序采用堆排序法
设计思路如下:
1. 设计药品信息类,包含药品编号、药名、药品单价、销售量、销售额等属性,以及相应的getter和setter方法。
2. 设计顺序表类,实现向表中添加药品信息、按编号、单价、销售量或销售额进行排序等方法。
3. 从txt文件中读取各药品的信息记录,存储在顺序表中。
4. 实现按编号、单价、销售量或销售额进行排序的方法,分别采用冒泡排序法、快速排序法、堆排序法。
5. 编写主程序,实现药品信息的读取、排序和输出。
示例代码如下:
```
//药品信息类
public class Medicine {
private String id; //药品编号
private String name; //药名
private double price; //药品单价
private int quantity; //销出数量
private double amount; //销售额
public Medicine(String id, String name, double price, int quantity, double amount) {
this.id = id;
this.name = name;
this.price = price;
this.quantity = quantity;
this.amount = amount;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
public double getAmount() {
return amount;
}
public void setAmount(double amount) {
this.amount = amount;
}
}
//顺序表类
public class MedicineList {
private ArrayList<Medicine> list;
public MedicineList() {
list = new ArrayList<>();
}
//向列表中添加药品信息
public void add(Medicine medicine) {
list.add(medicine);
}
//按编号排序
public void sortByNumber() {
Collections.sort(list, new Comparator<Medicine>() {
@Override
public int compare(Medicine o1, Medicine o2) {
return o1.getId().compareTo(o2.getId());
}
});
}
//按单价排序
public void sortByPrice() {
for (int i = 0; i < list.size() - 1; i++) {
for (int j = 0; j < list.size() - i - 1; j++) {
if (list.get(j).getPrice() > list.get(j + 1).getPrice()) {
Collections.swap(list, j, j + 1);
}
}
}
}
//按销售量排序
public void sortByQuantity() {
quickSort(0, list.size() - 1, 2);
}
//按销售额排序
public void sortByAmount() {
int n = list.size();
for (int i = n / 2 - 1; i >= 0; i--)
heapify(n, i, 3);
for (int i = n - 1; i > 0; i--) {
Collections.swap(list, 0, i);
heapify(i, 0, 3);
}
}
//快速排序
private void quickSort(int low, int high, int col) {
if (low < high) {
int i = low, j = high;
Medicine pivot = list.get(low + (high - low) / 2);
while (i <= j) {
while (list.get(i).getQuantity() > pivot.getQuantity() && col == 2) {
i++;
}
while (list.get(i).getAmount() > pivot.getAmount() && col == 3) {
i++;
}
while (list.get(j).getQuantity() < pivot.getQuantity() && col == 2) {
j--;
}
while (list.get(j).getAmount() < pivot.getAmount() && col == 3) {
j--;
}
if (i <= j) {
Collections.swap(list, i, j);
i++;
j--;
}
}
if (low < j) {
quickSort(low, j, col);
}
if (high > i) {
quickSort(i, high, col);
}
}
}
//堆排序
private void heapify(int n, int i, int col) {
int largest = i;
int l = 2 * i + 1;
int r = 2 * i + 2;
if (l < n && ((col == 2 && list.get(l).getQuantity() > list.get(largest).getQuantity())
|| (col == 3 && list.get(l).getAmount() > list.get(largest).getAmount()))) {
largest = l;
}
if (r < n && ((col == 2 && list.get(r).getQuantity() > list.get(largest).getQuantity())
|| (col == 3 && list.get(r).getAmount() > list.get(largest).getAmount()))) {
largest = r;
}
if (largest != i) {
Collections.swap(list, i, largest);
heapify(n, largest, col);
}
}
}
//主程序
public class Main {
public static void main(String[] args) {
MedicineList medicineList = new MedicineList();
try {
BufferedReader reader = new BufferedReader(new FileReader("medicine.txt"));
String line = "";
while ((line = reader.readLine()) != null) {
String[] data = line.split(",");
Medicine medicine = new Medicine(data[0], data[1], Double.parseDouble(data[2]),
Integer.parseInt(data[3]), Double.parseDouble(data[4]));
medicineList.add(medicine);
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
System.out.println("按编号排序:");
medicineList.sortByNumber();
printMedicineList(medicineList);
System.out.println("按单价排序:");
medicineList.sortByPrice();
printMedicineList(medicineList);
System.out.println("按销售量排序:");
medicineList.sortByQuantity();
printMedicineList(medicineList);
System.out.println("按销售额排序:");
medicineList.sortByAmount();
printMedicineList(medicineList);
}
private static void printMedicineList(MedicineList medicineList) {
for (Medicine medicine : medicineList.getList()) {
System.out.println(medicine.getId() + " " + medicine.getName() + " " +
medicine.getPrice() + " " + medicine.getQuantity() + " " + medicine.getAmount());
}
}
}
```
这是一个简单的示例,你可以根据实际需求进行修改和完善。
相关推荐
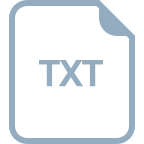
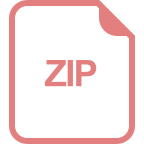
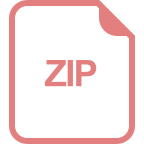













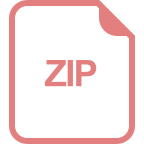
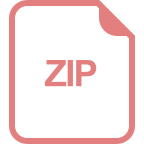