选出数组对象中重复最多的字段id,这个id是一个变量,可以为数组中的任何元素
时间: 2024-03-27 18:40:55 浏览: 19
好的,可以使用 JavaScript 代码来实现此功能,具体实现方法如下:
```javascript
function findMostFrequentId(arr) {
// 统计每个id出现的次数
const idCount = {};
arr.forEach(obj => {
if (obj.hasOwnProperty('id')) {
const id = obj.id;
idCount[id] = idCount[id] ? idCount[id] + 1 : 1;
}
});
// 找出出现次数最多的id
let mostFrequentId;
let maxCount = 0;
for (const id in idCount) {
if (idCount[id] > maxCount) {
maxCount = idCount[id];
mostFrequentId = id;
}
}
return mostFrequentId;
}
```
该函数接收一个数组参数 `arr`,遍历数组中的每个对象,统计每个id出现的次数,最终返回出现次数最多的id。注意,该函数假设数组中的每个对象都有一个 `id` 属性。
调用该函数的示例代码如下:
```javascript
const arr = [
{ id: 'a', name: 'apple' },
{ id: 'b', name: 'banana' },
{ id: 'c', name: 'cherry' },
{ id: 'a', name: 'apricot' },
{ id: 'a', name: 'avocado' },
{ name: 'watermelon' },
{ id: 'c', name: 'cantaloupe' },
{ id: 'c', name: 'coconut' }
];
const mostFrequentId = findMostFrequentId(arr);
console.log(mostFrequentId); // 输出 "c"
```
在上述示例中,数组中出现次数最多的id是 "c",因此函数返回了该值。
相关推荐
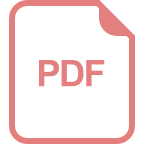
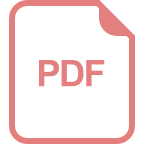














