esp32 wifi
时间: 2023-07-03 13:04:48 浏览: 108
ESP32是一种低成本、低功耗的微控制器,它内置了WiFi和蓝牙模块,可以轻松地连接到互联网或其他设备。
要使用ESP32连接WiFi网络,您可以使用ESP-IDF(ESP32开发框架)提供的WiFi库。以下是基本的步骤:
1. 在ESP-IDF中配置WiFi连接参数,例如SSID和密码。
2. 初始化WiFi连接并启用WiFi。
3. 使用WiFi管理器扫描可用的WiFi网络。
4. 连接到您选择的WiFi网络。
5. 确认连接成功并开始使用网络。
以下是一个简单的ESP-IDF程序,演示了如何连接到WiFi网络:
```
#include <string.h>
#include <stdlib.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "esp_system.h"
#include "esp_wifi.h"
#include "esp_event.h"
#include "esp_log.h"
#define WIFI_SSID "your_wifi_ssid"
#define WIFI_PASS "your_wifi_password"
static const char *TAG = "wifi station";
static void wifi_event_handler(void* arg, esp_event_base_t event_base,
int32_t event_id, void* event_data)
{
if (event_id == WIFI_EVENT_STA_START) {
esp_wifi_connect();
} else if (event_id == WIFI_EVENT_STA_DISCONNECTED) {
esp_wifi_connect();
ESP_LOGI(TAG,"retry to connect to the AP");
} else if (event_id == WIFI_EVENT_STA_CONNECTED) {
ESP_LOGI(TAG,"connected to AP");
}
}
void wifi_init_sta(void)
{
tcpip_adapter_init();
ESP_ERROR_CHECK(esp_event_loop_create_default());
wifi_init_config_t cfg = WIFI_INIT_CONFIG_DEFAULT();
ESP_ERROR_CHECK(esp_wifi_init(&cfg));
esp_event_handler_instance_t instance_any_id;
esp_event_handler_instance_t instance_got_ip;
esp_event_handler_instance_register(WIFI_EVENT,
ESP_EVENT_ANY_ID,
&wifi_event_handler,
NULL,
&instance_any_id);
esp_event_handler_instance_register(IP_EVENT,
IP_EVENT_STA_GOT_IP,
&wifi_event_handler,
NULL,
&instance_got_ip);
wifi_config_t wifi_config = {
.sta = {
.ssid = WIFI_SSID,
.password = WIFI_PASS,
.threshold.authmode = WIFI_AUTH_WPA2_PSK,
.pmf_cfg = {
.capable = true,
.required = false
},
},
};
ESP_ERROR_CHECK(esp_wifi_set_mode(WIFI_MODE_STA));
ESP_ERROR_CHECK(esp_wifi_set_config(ESP_IF_WIFI_STA, &wifi_config));
ESP_ERROR_CHECK(esp_wifi_start());
ESP_LOGI(TAG, "wifi_init_sta finished.");
}
void app_main()
{
ESP_ERROR_CHECK(nvs_flash_init());
wifi_init_sta();
}
```
请注意,此示例仅适用于连接到使用WPA2密码保护的WiFi网络。如果您的网络使用不同的安全协议或没有密码,请相应地更改WiFi配置。
阅读全文
相关推荐
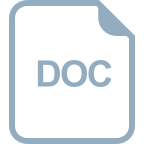
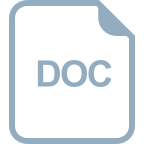
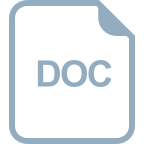
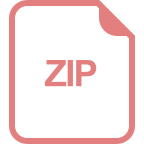
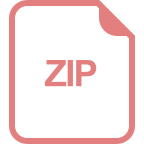
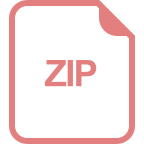
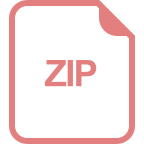
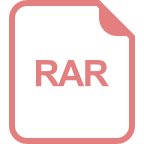










