我使用pytorch框架训练好了一个resnet18图像分类模型,并实现了摄像头实时识别。现在想将它展示在用Django实现的web端,具体需求是在web端点击开始识别,会调用电脑摄像头加载模型进行实时识别并将画面展现在web端,点击停止识别,画面消失。应该如何实现这个功能,能给出具体完整的代吗
时间: 2023-12-30 07:02:34 浏览: 161
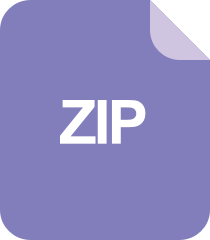
yolo3-pytorch-框架,可以使用该框架训练所需要的识别口罩、头盔、害虫、摩托车等模型
实现这个功能,需要进行以下几个步骤:
1. 在 Django 项目中创建一个视图函数,用于接收用户的请求,开始或停止识别。
2. 在该视图函数中,调用摄像头并使用 OpenCV 进行图像处理,将图像传入已训练好的 ResNet18 模型进行识别,并将结果返回。
3. 将识别结果实时展示在 web 页面上,可以使用 WebSocket 或 Comet 技术实现。
下面是一个可能的实现过程,仅供参考:
1. 安装必要的库
```
pip install django opencv-python numpy torch torchvision
```
2. 创建 Django 项目和应用
```
django-admin startproject image_classification
cd image_classification
python manage.py startapp classify
```
3. 在 classify/views.py 中实现视图函数
```python
import cv2
import numpy as np
from django.http import StreamingHttpResponse
from django.views.decorators import gzip
from django.shortcuts import render
import torch
import torchvision.models as models
import torchvision.transforms as transforms
# 加载模型
model = models.resnet18(pretrained=True)
model.eval()
# 定义图像转换
transform = transforms.Compose([
transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406],
std=[0.229, 0.224, 0.225])
])
# 定义分类标签
classes = ['class1', 'class2', ..., 'classN']
# 定义摄像头
cap = cv2.VideoCapture(0)
# 定义缓存
buffer = None
def classify_image(frame):
# 将图像转换为 PyTorch Tensor,调整维度
img_tensor = transform(frame).unsqueeze(0)
# 使用模型进行预测
with torch.no_grad():
outputs = model(img_tensor)
_, predicted = torch.max(outputs.data, 1)
# 返回分类结果
return classes[predicted[0]]
@gzip.gzip_page
def live_feed(request):
# 读取摄像头并处理
global buffer
success, frame = cap.read()
if success:
# 识别图像
label = classify_image(frame)
# 在图像上绘制分类标签
cv2.putText(frame, label, (10, 30),
cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 255, 0), 2)
# 转换为 JPEG 格式并压缩
ret, buffer = cv2.imencode('.jpg', frame)
response = StreamingHttpResponse(buffer.tobytes(),
content_type='image/jpeg')
response['Content-Length'] = buffer.tobytes().nbytes
return response
else:
return HttpResponse("Failed to open camera.")
def index(request):
return render(request, 'index.html')
```
4. 在 classify/urls.py 中定义 URL 路由
```python
from django.urls import path
from .views import index, live_feed
urlpatterns = [
path('', index, name='index'),
path('live_feed/', live_feed, name='live_feed'),
]
```
5. 在 image_classification/urls.py 中包含 classify 的 URL 路由
```python
from django.urls import include, path
urlpatterns = [
path('', include('classify.urls')),
]
```
6. 在模板文件 index.html 中添加开始和停止按钮,并使用 JavaScript 调用实时识别
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Real-time Image Classification</title>
</head>
<body>
<h1>Real-time Image Classification</h1>
<button id="start">Start</button>
<button id="stop">Stop</button>
<br>
<img id="video" alt="">
<script src="https://cdn.jsdelivr.net/npm/@tensorflow/tfjs@2.0.1/dist/tf.min.js"></script>
<script type="text/javascript">
var video = document.getElementById('video');
var startButton = document.getElementById('start');
var stopButton = document.getElementById('stop');
var ws;
startButton.onclick = function() {
ws = new WebSocket('ws://' + window.location.host + '/live_feed/');
ws.binaryType = 'arraybuffer';
ws.onmessage = function(event) {
var blob = new Blob([event.data], {type: 'image/jpeg'});
var url = window.URL || window.webkitURL;
var imageUrl = url.createObjectURL(blob);
video.src = imageUrl;
};
};
stopButton.onclick = function() {
ws.close();
};
</script>
</body>
</html>
```
7. 运行 Django 服务器,并在浏览器中访问 http://localhost:8000/,点击开始进行实时识别,点击停止结束识别。
```
python manage.py runserver
```
这是一个简单的实现过程,具体代码可能需要根据实际情况进行修改。同时,需要注意安全问题,如未授权访问、跨站脚本攻击等。
阅读全文
相关推荐
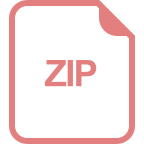
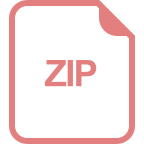
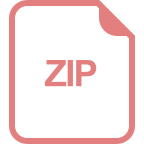

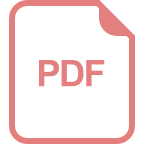
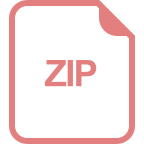
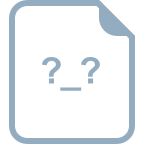
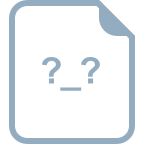
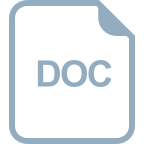
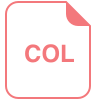
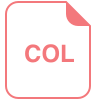
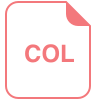
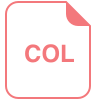
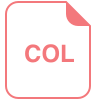



