C# TCP通讯接收信息后弹窗提示
时间: 2023-08-12 17:04:14 浏览: 142
在 C# 中,你可以使用 MessageBox 类来创建弹窗提示。下面是一个示例代码,用于在接收到 TCP 通讯信息后弹窗提示:
```csharp
using System;
using System.Net;
using System.Net.Sockets;
using System.Text;
using System.Windows.Forms;
public class TCPListenerExample
{
private static bool isRunning = true;
public static void Main()
{
// 创建一个 TCP 服务器
TcpListener server = null;
try
{
// 设置服务器 IP 地址和端口号
IPAddress ipAddress = IPAddress.Parse("127.0.0.1");
int port = 5555;
// 创建监听器
server = new TcpListener(ipAddress, port);
// 开始监听
server.Start();
// 在循环中接收客户端连接和消息
while (isRunning)
{
Console.WriteLine("等待客户端连接...");
// 接受客户端连接
TcpClient client = server.AcceptTcpClient();
Console.WriteLine("客户端已连接,等待消息...");
// 从客户端获取网络流
NetworkStream stream = client.GetStream();
// 读取客户端发送的消息
byte[] buffer = new byte[1024];
int bytesRead = stream.Read(buffer, 0, buffer.Length);
string message = Encoding.ASCII.GetString(buffer, 0, bytesRead);
Console.WriteLine("接收到消息: {0}", message);
// 弹窗提示接收到的消息
MessageBox.Show("接收到消息: " + message);
// 关闭客户端连接
client.Close();
}
}
catch (Exception e)
{
Console.WriteLine("发生异常: {0}", e);
}
finally
{
// 停止监听
server.Stop();
}
Console.WriteLine("程序已退出");
}
}
```
在上述代码中,我们创建了一个 TCP 服务器,并在循环中接收客户端连接和消息。当接收到消息后,会使用 MessageBox 类创建一个弹窗提示。
请注意,这只是一个简单的示例代码,你可能需要根据你的具体需求进行更多的功能扩展和错误处理。
阅读全文
相关推荐
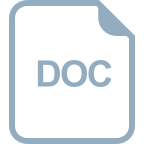
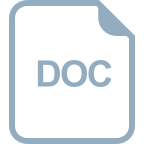
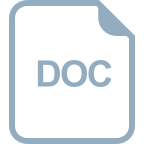
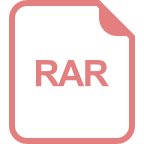
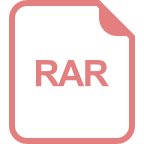
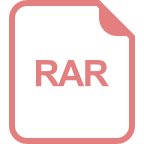
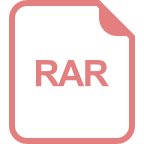
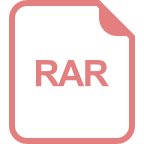
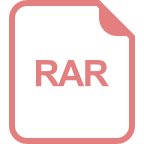
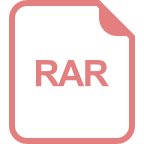
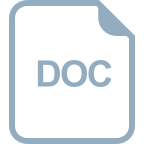
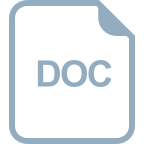
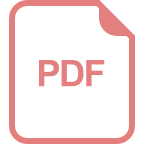
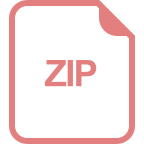
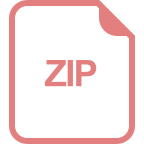
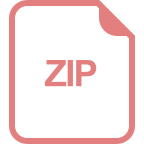
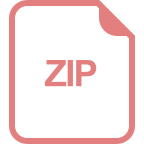